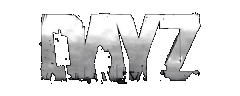 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
6 RegisterActions(action_array_names);
7 Sort(action_array_names,action_array_names.Count());
13 for(
int i = 0; i < action_array_names.Count(); i++)
18 new_action.CreateConditionComponents();
19 new_action.SetID(actionsArray.Count());
20 actionsArray.Insert(new_action);
21 actionNameActionMap.Insert(action_array_names[i], new_action);
204 actions.Insert(ActionActionBuildPartNoTool);
318 actions.Insert(ActionSwapItemToHands);
338 actions.Insert(ActionUnpin);
339 actions.Insert(ActionPin);
ActionDigWormsCB ActionDigOutStash
ActionDismantlePartCB ActionDismantleOven
ActionDigGardenPlotCB ActionDialCombinationLockOnTarget
PlaceObjectActionReciveData ActionReciveData ActionDeployObject()
ActionBuildOvenCB ActionContinuousBaseCB ActionBuildOven()
ActionDetachFromTarget_SpecificSlot_WoodenPlanks ActionDetachFromTarget_SpecificSlot ActionDetachFromTarget_SpecificSlot_MetalSheets()
ActionSplintSelfCB ActionSewTarget
ActionUncoverHeadSelfCB ActionTurnValveUndergroundReservoir
ActionLoadMagazineCB ActionLightItemOnFireWithBlowtorch
proto void Sort(void param_array[], int num)
Sorts static array of integers(ascendically) / floats(ascendically) / strings(alphabetically)
ActionRepairCarChassisWithBlowtorchCB ActionRepairCarChassisCB ActionRepairCarChassisWithBlowtorch()
ActionMineTreeBark ActionMineTree
ActionDisarmExplosiveCB ActionContinuousBaseCB ActionDisarmExplosive()
ActionEatBigCB ActionDrinkWellContinuous
ActionEnterLadder ActionDetachFromTarget_SpecificSlotsCategory_WoodenCrate
ActionSewSelfCB ActionContinuousBaseCB ActionSewSelf()
void ActionRepairCarEngine()
ActionExtinguishFireplaceByExtinguisherCB ActionContinuousBaseCB ActionExtinguishFireplaceByExtinguisher()
ActionRepairCarPartWithBlowtorchCB ActionRepairCarPartCB ActionRepairCarPartWithBlowtorch()
ActionWashHandsWaterCB ActionContinuousBaseCB ActionWashHandsWater()
ActionDisinfectPlantCB ActionDisarmMine
ActionDrainLiquidCB ActionDismantleStoneCircle
ActionBreakLongWoodenStickCB ActionAttachExplosivesTrigger
FirearmActionLoadMultiBullet FirearmActionLoadBulletQuick
void ActionForceConsumeSingle()
ActionFoldBaseBuildingObjectCB ActionContinuousBaseCB ActionFoldBaseBuildingObject()
ActionWaterGardenSlotCB ActionWashHandsWell
ActionDeCraftRopeBeltCB ActionContinuousBaseCB ActionDeCraftRopeBelt()
ActionActivateTrapCB ActionContinuousBaseCB ActionActivateTrap()
ActionDestroyCombinationLockCB ActionDeCraftWitchHoodCoif
ActionRepairItemWithBlowtorchCB ActionContinuousBaseCB ActionRepairItemWithBlowtorch()
ActionDisarmExplosiveWithRemoteDetonatorUnpairedCB ActionDisarmExplosiveCB ActionDisarmExplosiveWithRemoteDetonatorUnpaired()
ActionBandageSelfCB ActionContinuousBaseCB ActionBandageSelf()
ActionSortAmmoPileCB ActionSkinning
array< typename > TTypenameArray
ActionTurnValveCB ActionContinuousBaseCB ActionTurnValve()
ActionTurnOffHeadtorch ActionTurnOffAlarmClock
ActionPickBerryCB ActionPackTent
ActionUncoverHeadTargetCB ActionUncoverHeadSelf
void Spawn()
spawn damage trigger
ActionDialCombinationLockCB ActionContinuousBaseCB ActionDialCombinationLock()
ActionArmExplosiveCB ActionContinuousBaseCB ActionArmExplosive()
ActionBuildStoneCircleCB ActionContinuousBaseCB ActionBuildStoneCircle()
ActionWashHandsItem ActionUnfoldMap
ActionLightItemOnFireCB ActionContinuousBaseCB ActionLightItemOnFire()
ActionTurnOnChemlight ActionTurnOnAlarmClock
void ActionRepairCarPart()
ActionForceDrinkCB ActionForceConsume
ActionRefuelTorch ActionRaiseMegaphone
ActionAttachWheels ActionAttach
ActionTuneFrequencyCB ActionContinuousBaseCB ActionTuneFrequency()
ActionTurnOnWeaponFlashlight ActionTurnOnTransmitter
ActionCraftBoltsCB ActionCraftArmband
void ActionDisinfectSelf()
void ActionStartEngine()
DEPRECATED.
ActionBurnSewSelfCB ActionBandageTarget
ActionForceFeedSmallCB ActionForceFeed
ActionDeCraftDrysackBagCB ActionContinuousBaseCB ActionDeCraftDrysackBag()
ActionFillBrakesCB ActionCarHornShort
ActionTurnOffWeaponFlashlight ActionTurnOffTransmitter
ActionFertilizeSlotCB ActionExtinguishFireplaceByLiquid
ActionRepairCarEngineWithBlowtorchCB ActionRepairCarEngineCB ActionRepairCarEngineWithBlowtorch()
ActionTakeABiteCB ActionResetKitchenTimer
void ActionGetOutTransport()
ActionTuneRadioStationCB ActionContinuousBaseCB ActionTuneRadioStation()
void ActionDrinkPondContinuous()
ActionDisarmExplosiveWithRemoteDetonatorCB ActionDisarmExplosiveCB ActionDisarmExplosiveWithRemoteDetonator()
ActionBuryBodyCB ActionBuryAshes
ActionDetachFromTarget_SpecificSlotsCategory ActionDetachFromTarget ActionDetachFromTarget_SpecificSlot_WoodenLogs()