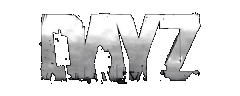 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
23 proto native external
bool IsInherited(
typename type);
37 proto native owned external
string ClassName();
39 string GetDebugName() {
return ClassName(); }
52 proto native external
typename Type();
64 proto external
static typename StaticType();
73 static typename StaticGetType(
typename t)
78 proto external
string ToString();
110 proto
static bool CastTo(out
Class to,
Class from);
113 private proto
static bool SafeCastType(
Class type, out
Class to,
Class from);
139 proto
volatile int Call(
Class inst,
string function,
void parm);
146 proto
volatile int CallFunction(
Class inst,
string function, out
void returnVal,
void parm);
147 proto
volatile int CallFunctionParams(
Class inst,
string function, out
void returnVal,
Class parms);
148 proto native
void Release();
188 static proto
int GetClassVar(
Class inst,
string varname,
int index, out
void result);
211 static proto
int SetClassVar(
Class inst,
string varname,
int index,
void input);
222 static proto
int SetVar(out
void var,
string value);
230 static proto
void Watch(
void var,
int flags);
253 proto
void Sort(
void param_array[],
int num);
255 proto
void copyarray(
void destArray,
void srcArray);
307 proto
int ParseString(
string input, out
string tokens[]);
323 proto
volatile void Idle();
356 proto native
int Count();
361 proto native
void Clear();
365 proto
void Set(
int n, T value);
370 proto
int Find(T value);
383 proto
int Insert(T value);
394 proto
int InsertAt(T value,
int index);
425 void InsertAll(notnull
array<T> from)
427 for (
int i = 0; i < from.Count(); i++ )
429 Insert( from.Get(i) );
438 proto native
void Remove(
int index);
445 proto native
void RemoveOrdered(
int index);
451 proto native
void Resize(
int newSize);
457 proto native
void Reserve(
int newSize);
463 proto native
void Swap(notnull
array<T> other);
468 proto native
void Sort(
bool reverse =
false);
474 proto
int Copy(notnull
array<T> from);
477 void RemoveItem(T value)
479 int remove_index = Find(value);
481 if ( remove_index >= 0 )
483 RemoveOrdered(remove_index);
487 void RemoveItemUnOrdered(T value)
489 int remove_index = Find(value);
491 if ( remove_index >= 0 )
497 bool IsValidIndex(
int index )
499 return ( index > -1 && index <
Count() );
525 Print(
string.Format(
"Array count: %1",
Count()));
526 for (
int i = 0; i <
Count(); i++)
529 Print(
string.Format(
"[%1] => %2", i, item));
563 return Get(GetRandomIndex());
566 void SwapItems(
int item1_index,
int item2_index)
568 T item1 =
Get(item1_index);
569 Set(item1_index,
Get(item2_index));
570 Set(item2_index, item1);
575 for (
int i = 0; i < other.Count(); i++)
577 T item = other.Get(i);
585 int right =
Count() - 1;
591 Set(left++,
Get(right));
610 int MoveIndex(
int curr_index,
int move_number)
613 int new_index = curr_index;
615 if ( move_number > 0 )
617 new_index = curr_index + move_number;
620 if ( move_number < 0 )
622 new_index = curr_index - move_number;
626 if ( new_index <= -count )
628 new_index = (new_index % count);
631 new_index = new_index + count;
635 if ( new_index >= count )
637 new_index = (new_index % count);
646 for (
int i = 0; i <
Count(); i++)
648 SwapItems(i,GetRandomIndex());
664 int DifferentAtPosition(
array<T> pOtherArray)
666 if (
Count() != pOtherArray.Count())
668 ErrorEx(
"arrays are not the same size");
672 for (
int i = 0; i < pOtherArray.Count(); ++i)
674 if (
Get(i) != pOtherArray.Get(i))
697 proto native
int Count();
698 proto native
void Clear();
703 proto
int Find(T value);
712 proto
int Insert(T value);
723 proto
int InsertAt(T value,
int index);
729 proto native
void Remove(
int index);
730 proto
int Copy(set<T> from);
731 proto native
void Swap(set<T> other);
734 void InsertSet(set<T> other)
736 int count = other.Count();
737 for (
int i = 0; i < count; i++)
744 void RemoveItem(T value)
746 int remove_index = Find(value);
747 if (remove_index >= 0)
753 void RemoveItems(set<T> other)
755 int count = other.Count();
756 for (
int i = 0; i < count; i++)
765 Print(
string.Format(
"Set count: %1",
Count()));
766 for (
int i = 0; i <
Count(); i++)
769 Print(
string.Format(
"[%1] => %2", i, item));
806 proto native
int Count();
811 proto native
void Clear();
820 proto TValue
Get(TKey key);
831 proto
bool Find(TKey key, out TValue val);
851 proto TKey GetKey(
int i);
856 proto
void Set(TKey key, TValue value);
860 proto
void Remove(TKey key);
867 proto
void RemoveElement(
int i);
871 proto
bool Contains(TKey key);
880 proto
bool Insert(TKey key, TValue value);
886 for (
int i = 0; i <
Count(); i++)
888 keys.Insert( GetKey( i ) );
896 for (
int i = 0; i <
Count(); i++)
903 bool ReplaceKey(TKey old_key, TKey new_key)
905 if (Contains(old_key))
907 Set(new_key,
Get(old_key));
914 TKey GetKeyByValue(TValue value)
917 for (
int i = 0; i <
Count(); i++)
929 bool GetKeyByValueChecked(TValue value, out TKey key)
931 for (
int i = 0; i <
Count(); i++)
map< Class, vector > TClassVectorMap
void End()
called on surrender end request end
proto owned string ThreadFunction(Class owner, string name, int backtrace, out int linenumber)
Debug function. Returns current function on stack of the thread.
map< string, string > TStringStringMap
map< Class, typename > TClassTypenameMap
map< string, typename > TStringTypenameMap
map< Class, Managed > TClassManagedMap
array< vector > TVectorArray
array< float > TFloatArray
map< Managed, float > TManagedFloatMap
map< Class, string > TClassStringMap
proto void Sort(void param_array[], int num)
Sorts static array of integers(ascendically) / floats(ascendically) / strings(alphabetically)
string String(string s)
Helper for passing string expression to functions with void parameter. Example: Print(String("Hello "...
void Remove(Object object)
array< string > TStringArray
set< ref Managed > TManagedRefSet
map< ref Managed, string > TManagedRefStringMap
array< Managed > TManagedArray
protected void Clear(bool clearFile=false)
set< typename > TTypenameSet
map< ref Managed, ref Managed > TManagedRefManagedRefMap
map< Managed, string > TManagedStringMap
map< ref Managed, vector > TManagedRefVectorMap
proto void Print(void var)
Prints content of variable to console/log.
proto int ParseString(string input, out string tokens[])
Parses string into array of tokens returns number of tokens.
map< string, int > TStringIntMap
DisplayElementBase GetElement(eDisplayElements element_id)
map< int, Managed > TIntManagedMap
map< Class, Class > TClassClassMap
map< int, vector > TIntVectorMap
map< string, Managed > TStringManagedMap
array< ref Managed > TManagedRefArray
map< Managed, Managed > TManagedManagedMap
array< typename > TTypenameArray
map< ref Managed, typename > TManagedRefTypenameMap
proto int ParseStringEx(inout string input, string token)
Parses one token from input string. Result is put into token string, and type of token is returned....
map< Managed, Class > TManagedClassMap
map< Managed, vector > TManagedVectorMap
map< Managed, typename > TManagedTypenameMap
map< int, string > TIntStringMap
proto volatile void Idle()
map< int, Class > TIntClassMap
class InventoryGridController extends ScriptedWidgetEventHandler Init
proto void copyarray(void destArray, void srcArray)
map< ref Managed, Class > TManagedRefClassMap
map< typename, Managed > TTypeNameManagedMap
map< int, int > TIntIntMap
map< string, float > TStringFloatMap
void PrintString(string s)
Helper for printing out string expression. Example: PrintString("Hello " + var);.
map< typename, int > TTypeNameIntMap
map< string, vector > TStringVectorMap
map< Class, ref Managed > TClassManagedRefMap
map< string, ref Managed > TStringManagedRefMap
array< ref PlayerStatBase > Get()
map< ref Managed, Managed > TManagedRefManagedMap
map< typename, string > TTypeNameStringMap
array< Class > TClassArray
Result for an object found in CGame.IsBoxCollidingGeometryProxy.
map< Managed, ref Managed > TManagedManagedRefMap
map< typename, typename > TTypeNameTypenameMap
map< int, ref Managed > TIntManagedRefMap
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
enum MagnumStableStateID init
map< Class, float > TClassFloatMap
map< typename, ref Managed > TTypeNameManagedRefMap
map< Class, int > TClassIntMap
Module containing compiled scripts.
map< int, float > TIntFloatMap
map< ref Managed, int > TManagedRefIntMap
map< Managed, int > TManagedIntMap
proto native int KillThread(Class owner, string name)
Kills thread.
map< typename, vector > TTypeNameVectorMap
set< Managed > TManagedSet
int[] TypeID
script representation for C++ RTTI types
map< ref Managed, float > TManagedRefFloatMap
map< string, Class > TStringClassMap
Super root of all classes in Enforce script.
map< typename, float > TTypeNameFloatMap
map< typename, Class > TTypeNameClassMap
map< int, typename > TIntTypenameMap
proto void reversearray(void param_array)