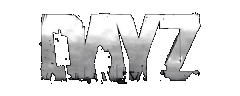 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
8 static int m_ForcedIndex = -1;
13 protected string m_RootPath =
"Gui/layouts/new_ui/hints/in_game_hints.layout";
14 protected const string m_DataPath =
"scripts/data/hints.json";
51 void Init(DayZGame game)
79 ErrorEx(
"Could not create the hint panel. The data are missing!");
173 #ifdef DIAG_DEVELOPER
176 if (m_ForcedIndex != -1)
185 Math.RandomFloat01();
295 override void Init(DayZGame game)
297 m_RootPath =
"Gui/layouts/new_ui/hints/in_game_hints_load.layout";
proto native CGame GetGame()
protected void StartSlideshow()
protected string m_RootPath
protected int m_PreviousRandomIndex
protected void SetHintDescription()
protected TextWidget m_UiHeadlineLabel
class UiHintPanel extends ScriptedWidgetEventHandler Init(DayZGame game)
void UiHintPanel(Widget parent_widget)
protected ImageWidget m_UiHintImage
protected Widget m_SpacerFrame
protected TextWidget m_UiPageingLabel
protected void ShowNextPage()
override bool OnMouseLeave(Widget w, Widget enterW, int x, int y)
protected void LoadContentList()
protected void RestartSlideShow()
protected ref array< ref HintPage > m_ContentList
protected int m_SlideShowDelay
protected ButtonWidget m_UiRightButton
protected void SlideshowThread()
protected void PopulateLayout()
protected void StopSlideShow()
const protected string m_DataPath
protected void SetHintPaging()
protected void SetHintHeadline()
protected void RandomizePageIndex()
protected void SetHintImage()
protected bool m_Initialized
protected Widget m_ParentWidget
protected RichTextWidget m_UiDescLabel
override bool OnClick(Widget w, int x, int y, int button)
protected Widget m_RootFrame
override bool OnMouseEnter(Widget w, int x, int y)
protected void ShowPreviousPage()
protected ButtonWidget m_UiLeftButton
protected void BuildLayout(Widget parent_widget)
protected DayZGame m_Game
protected int m_PageIndex