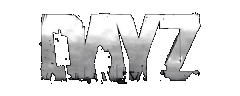 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
53 proto
void DebugBreak(
bool condition =
true,
void param1 = NULL,
void param2 = NULL,
void param3 = NULL,
void param4 = NULL,
void param5 = NULL,
void param6 = NULL,
void param7 = NULL,
void param8 = NULL,
void param9 = NULL);
59 proto
void DPrint(
string var);
87 proto native
void Error2(
string title,
string err);
96 proto
void Print(
void var);
108 proto
void PrintFormat(
string fmt,
void param1 = NULL,
void param2 = NULL,
void param3 = NULL,
void param4 = NULL,
void param5 = NULL,
void param6 = NULL,
void param7 = NULL,
void param8 = NULL,
void param9 = NULL);
156 proto
private void ~
Shape();
162 proto native
void SetColor(
int color);
170 proto
static native
Shape CreateFrustum(
float horizontalAngle,
float verticalAngle,
float length,
int color,
ShapeFlags flags);
171 proto
static native
Shape CreateCylinder(
int color,
ShapeFlags flags,
vector origin,
float radius,
float length);
180 vector dir2 = dir.Perpend() * size;
185 pts[2] = to - dir1 - dir2;
186 pts[3] = to - dir1 + dir2;
189 return CreateLines(color, flags, pts, 5);
200 vector dir2 = dir.Perpend() * size;
204 pts[1] = from +
"0 0 1";
205 pts[2] = to +
"0 0 1";
207 pts[4] = to - dir1 - dir2;
208 pts[5] = to - dir1 + dir2;
211 return CreateLines(color, flags, pts, 7);
214 static void CreateMatrix(
vector mat[4])
218 Create(
ShapeType.LINE, 0xffff0000, flags, org, mat[0] * 0.5 + org);
219 Create(
ShapeType.LINE, 0xff00ff00, flags, org, mat[1] * 0.5 + org);
220 Create(
ShapeType.LINE, 0xff0000ff, flags, org, mat[2] * 0.5 + org);
235 static proto
bool IsInitialized();
238 static proto
void InitScriptDiags();
240 static proto
void ClearScriptDiags();
248 static proto
void RegisterMenu(
int id,
string name,
int parent);
259 static proto
void RegisterItem(
int id,
string shortcut,
string name,
int parent,
string values,
func callback =
null);
272 static proto
void RegisterBool(
int id,
string shortcut,
string name,
int parent,
bool reverse =
false,
func callback =
null);
283 static proto
void RegisterRange(
int id,
string shortcut,
string name,
int parent,
string valuenames,
func callback =
null);
286 static proto
void Unregister(
int id);
289 static proto
bool IsRegistered(
int id);
309 static proto
bool BindCallback(
int id,
func callback);
311 static proto
void UnbindCallback(
int id);
314 static proto
bool GetBool(
int id,
bool reverse =
false);
316 static proto
int GetValue(
int id);
318 static proto
void SetValue(
int id,
int value);
321 static proto
float GetRangeValue(
int id);
323 static proto
void SetRangeValue(
int id,
float value);
326 static proto
int GetEngineValue(
int id);
328 static proto
void SetEngineValue(
int id,
int value);
331 static proto
float GetEngineRangeValue(
int id);
333 static proto
void SetEngineRangeValue(
int id,
float value);
336 static proto
bool MenuExists(
string name);
proto void DPrint(string var)
Prints content of variable to console/log. Should be used for critical messages so it will appear in ...
proto void ErrorExString(string err, out string str, ErrorExSeverity severity=ErrorExSeverity.ERROR)
void Error(string err)
Messagebox with error message.
proto void DumpStackString(out string stack)
Prints current call stack (stack trace) to given output.
proto native void SetColor(int color)
proto native void GetMatrix(out vector mat[4])
proto void Print(void var)
Prints content of variable to console/log.
proto native void Destroy()
Cleans up the Effect, including unregistering if needed.
proto native void Error2(string title, string err)
Messagebox with error message.
proto void PrintToRPT(void var)
Prints content of variable to RPT file (performance warning - each write means fflush!...
proto native void SetMatrix(vector mat[4])
proto void PrintFormat(string fmt, void param1=NULL, void param2=NULL, void param3=NULL, void param4=NULL, void param5=NULL, void param6=NULL, void param7=NULL, void param8=NULL, void param9=NULL)
Prints formated text to console/log.
proto native void SetFlags(ShapeFlags flags)
proto void DumpStack()
Prints current call stack (stack trace)
void CompileBreak()
Triggers breakpoint in C++ in compile time(when app is running in debug enviroment)
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
proto native void SetPosition(vector position)
Set the world position of the Effect.
proto void DebugBreak(bool condition=true, void param1=NULL, void param2=NULL, void param3=NULL, void param4=NULL, void param5=NULL, void param6=NULL, void param7=NULL, void param8=NULL, void param9=NULL)
Triggers breakpoint in C++ in run time(when app is running in debug enviroment)
proto native void SetDirection(vector direction)
proto native vector Vector(float x, float y, float z)
Vector constructor from components.
class DiagMenu Shape
don't call destructor directly. Use Destroy() instead