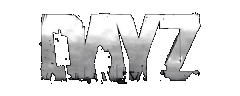 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
proto native CGame GetGame()
void Event_OnUnregistered()
Event called from SEffectManager when the Effect is unregistered.
EffectType
Enum to determine what type of effect the Effect is.
vector GetAttachedLocalOri()
Get the local orientation set by SetAttachedLocalOri.
protected vector m_LocalPos
Cached local pos.
protected bool m_IsPlaying
Whether the Effect is currently playing.
void SetAttachmentParent(Object obj)
Set parent for the Effect.
void Event_OnFrameUpdate(float time_delta)
Event called on frame when enabled by SetEnableEventFrame(true)
Object GetCurrentParent()
Get the current parent of the managed Effect.
vector GetCurrentPosition()
Get the current world position of the managed effect.
vector GetCurrentLocalPosition()
Get the current local position of the managed effect.
EffectType GetEffectType()
Get what type of effect the Effect is.
protected vector m_Position
Cached world position.
vector GetPosition()
Get the world position of the Effect.
protected bool m_IsPendingDeletion
Whether the Destroy process has already been called.
bool IsAutodestroy()
Get whether Effect automatically cleans up when it stops.
protected void SetID(int id)
Set the ID registered in SEffectManager.
protected Object m_ParentObject
Cached parent.
void OnCheckUpdate()
Event used when EffectParticle.CheckLifeSpan was called (DEPRECATED)
void SetAttachedLocalPos(vector pos)
Set local pos for the Effect relative to the parent.
void SetEnableEventFrame(bool enable)
Enable Event_OnFrameUpdate for the effect.
void SetAttachedLocalOri(vector ori)
Set local orientation for the Effectparticle to attach to when the Effect is started.
bool IsParticle()
Check whether the Effect is EffectParticle without casting.
int GetID()
Get the ID registered in SEffectManager.
protected void Destroy()
Cleans up the Effect, including unregistering if needed.
void SetCurrentParent(Object parent_obj, bool updateCached=true)
Set current parent of the managed effect.
vector GetAttachedLocalPos()
Get the local pos set by SetAttachedLocalPos.
bool IsPlaying()
Returns true when the Effect is playing, false otherwise.
vector GetLocalPosition()
Get the local position of the Effect.
bool IsRegistered()
Get whether this Effect is registered in SEffectManager.
void Event_OnRegistered(int id)
Event called from SEffectManager when the Effect is registered.
void SetAutodestroy(bool auto_destroy)
Sets whether Effect automatically cleans up when it stops.
ref ScriptInvoker Event_OnStopped
Event used when Stop was called.
ref ScriptInvoker Event_OnEffectEnded
Event used when the actual effect stopped playing.
bool IsSound()
Check whether the Effect is EffectSound without casting.
void SetCurrentLocalPosition(vector pos, bool updateCached=true)
Set the current local position of the managed effect.
void SetPosition(vector pos)
Set the world position of the Effect.
bool CanDestroy()
Get whether the Effect can be destroyed right now.
ref ScriptInvoker Event_OnEffectStarted
Event used when the actual effect started playing.
protected bool m_IsAutodestroy
Whether the Effect cleans up after itself when stopped.
bool IsPendingDeletion()
Get whether the Effect is queued up for being cleaned up.
Event_OnStarted
Event used when Start was called.
void SetParent(Object parent_obj)
Set parent of the Effect.
protected bool m_IsRegistered
Whether the effect is registered in SEffectManager.
void SetCurrentPosition(vector pos, bool updateCached=true)
Set the current world position of the managed effect.
protected vector m_LocalOri
Local orientation set by SetAttachedLocalOri, only used by EffectParticle.
void SetLocalPosition(vector pos)
Set the local position of the Effect.
void ValidateStart()
Validation whether an effect truly started playing or if the Effect should stop as none is present.
void Start()
Plays all elements this effects consists of.
Manager class for managing Effect (EffectParticle, EffectSound)
protected int m_ID
ID of effect, given by SEffectManager when registered (automatically done when playing through it)
Object GetAttachmentParent()
Get the parent set by SetAttachmentParent.
void Stop()
Stops all elements this effect consists of.
ScriptInvoker Class provide list of callbacks usage: