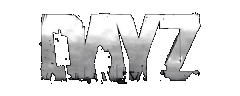 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
15 MultilineTextWidgetTypeID,
17 MultilineEditBoxWidgetTypeID,
21 RenderTargetWidgetTypeID,
29 RTTextureWidgetTypeID,
38 SimpleProgressBarWidgetTypeID,
39 ProgressBarWidgetTypeID,
41 BaseListboxWidgetTypeID,
42 TextListboxWidgetTypeID,
43 GenericListboxWidgetTypeID,
45 PasswordEditBoxWidgetTypeID,
46 WorkspaceWidgetTypeID,
47 GridSpacerWidgetTypeID,
48 WrapSpacerWidgetTypeID,
110 proto
private void Widget();
112 proto
static string TranslateString(
string stringId);
115 proto
static void SetLV(
float lv);
117 proto
static void SetTextLV(
float lv);
119 proto
static void SetObjectLighting(
float lighting);
121 proto native owned
string GetName();
125 proto native
void Show(
bool show,
bool immedUpdate =
true);
126 proto native
void Enable(
bool enable);
128 proto native
int SetFlags(
int flags,
bool immedUpdate =
true);
130 proto native
void SetSort(
int sort,
bool immedUpdate =
true);
131 proto native
int ClearFlags(
int flags,
bool immedUpdate =
true);
140 proto native
void SetPos(
float x,
float y,
bool immedUpdate =
true);
141 proto native
void SetSize(
float w,
float h,
bool immedUpdate =
true);
142 proto native
void SetScreenPos(
float x,
float y,
bool immedUpdate =
true);
143 proto native
void SetScreenSize(
float w,
float h,
bool immedUpdate =
true);
144 proto native
void SetColor(
int color);
146 proto native
void SetRotation(
float roll,
float pitch,
float yaw,
bool immedUpdate =
true);
149 proto native
void SetAlpha(
float alpha);
151 proto
void GetPos(out
float x, out
float y);
152 proto
void GetSize(out
float width, out
float height);
161 proto native
void AddChild(
Widget child,
bool immedUpdate =
true);
164 proto native
volatile void Update();
173 proto native
void Unlink();
179 proto native external
Widget CreateWidget(
WidgetType type,
int left,
int top,
int width,
int height,
WidgetFlags flags,
int color,
int sort,
Widget parentWidget = NULL);
181 proto native external
Widget CreateWidgets(
string layout,
Widget parentWidget = NULL,
bool immedUpdate =
true);
191 proto native
void SetTextSpacing(
int horiz,
int vert);
193 proto native
void SetTextExactSize(
int size);
194 proto native
void SetTextOffset(
int left,
int top);
195 proto native
void SetText(
string text,
bool immedUpdate =
true);
196 proto native
void SetOutline(
int outlineSize,
int argb = 0xFF000000);
197 proto native
int GetOutlineSize();
198 proto native
int GetOutlineColor();
199 proto native
void SetShadow(
int shadowSize,
int shadowARGB = 0xFF000000,
float shadowOpacity = 1,
float shadowOffsetX = 0,
float shadowOffsetY = 0);
200 proto native
int GetShadowSize();
201 proto native
int GetShadowColor();
202 proto native
float GetShadowOpacity();
203 proto
void GetShadowOffset(out
float sx, out
float sy);
204 proto native
void SetItalic(
bool italic);
205 proto native
bool GetItalic();
206 proto native
void SetBold(
bool bold);
207 proto native
bool GetBold();
210 proto
void GetTextSize(out
int sx, out
int sy);
211 proto
void SetTextFormat(
string text,
void param1 = NULL,
void param2 = NULL,
void param3 = NULL,
void param4 = NULL,
void param5 = NULL,
void param6 = NULL,
void param7 = NULL,
void param8 = NULL,
void param9 = NULL);
214 proto native
float GetTextProportion();
216 proto native
void SetTextProportion(
float val);
221 proto native
float SetLineBreakingOverride(
int mode);
227 proto native
float GetContentOffset();
228 proto native
void SetContentOffset(
float offset,
bool snapToLine =
false);
229 proto native
void ElideText(
int line,
float maxWidth,
string str);
230 proto native
int GetNumLines();
231 proto native
void SetLinesVisibility(
int lineFrom,
int lineTo,
bool visible);
232 proto native
float GetLineWidth(
int line);
233 proto native
float SetLineBreakingOverride(
int mode);
236 class RenderTargetWidget
extends Widget
239 proto native
void SetRefresh(
int period,
int offset);
240 proto native
void SetResolutionScale(
float xscale,
float ycale);
243 class RTTextureWidget
extends Widget
247 class ImageWidget
extends Widget
257 proto native
bool LoadImageFile(
int num,
string name,
bool noCache =
false);
258 proto native
void SetImageTexture(
int image, RTTextureWidget texture);
260 proto
void GetImageSize(
int image, out
int sx, out
int sy);
267 proto native
bool SetImage(
int num);
269 proto native
int GetImage();
275 proto native
void SetUV(
float uv[4][2]);
282 proto native
bool LoadMaskTexture(
string resource);
290 proto native
float GetMaskProgress();
296 proto native
void SetMaskProgress(
float value);
304 proto native
float GetMaskTransitionWidth();
310 proto native
void SetMaskTransitionWidth(
float value);
313 class MultilineEditBoxWidget
extends TextWidget
315 proto native
int GetLinesCount();
316 proto native
int GetCarriageLine();
317 proto native
int GetCarriagePos();
318 proto
void GetText(out
string text);
319 proto native
void SetLine(
int line,
string text);
320 proto
void GetLine(
int line, out
string text);
325 proto native
void SetTextColor(
int color);
326 proto native
void SetTextOutline(
int outlineSize,
int argb = 0xFF000000);
327 proto native
int GetTextOutlineSize();
328 proto native
int GetTextOutlineColor();
329 proto native
void SetTextShadow(
int shadowSize,
int shadowARGB = 0xFF000000,
float shadowOpacity = 1.0,
float shadowOffsetX = 0.0,
float shadowOffsetY = 0.0);
330 proto native
int GetTextShadowSize();
331 proto native
int GetTextShadowColor();
332 proto native
float GetTextShadowOpacity();
333 proto native
float GetTextShadowOffsetX();
334 proto native
float GetTextShadowOffsetY();
335 proto native
void SetTextItalic(
bool italic);
336 proto native
bool GetTextItalic();
337 proto native
void SetTextBold(
bool bold);
338 proto native
bool GetTextBold();
341 class CanvasWidget
extends Widget
343 proto native
void DrawLine(
float x1,
float y1,
float x2,
float y2,
float width,
int color);
344 proto native
void Clear();
350 proto native
void SetText(
string str);
355 proto native
void SetHideText(
bool hide);
360 proto native
void SetMinMax(
float minimum,
float maximum);
361 proto native
float GetMin();
362 proto native
float GetMax();
363 proto native
float GetCurrent();
364 proto native
void SetCurrent(
float curr);
365 proto native
float GetStep();
366 proto native
void SetStep(
float step);
371 proto native
float GetMin();
372 proto native
float GetMax();
373 proto native
float GetCurrent();
374 proto native
void SetCurrent(
float curr);
385 proto native
bool SetState(
bool state);
387 proto native
void SetText(
string text);
389 proto
void GetText(out
string text);
391 proto native
void SetTextOffset(
float xoffset,
float yoffset);
395 proto native
void SetTextHorizontalAlignment(
int align);
399 proto native
void SetTextVerticalAlignment(
int align);
402 proto native
float GetTextProportion();
404 proto native
void SetTextProportion(
float val);
407 class XComboBoxWidget
extends UIWidget
409 proto native
int AddItem(
string item);
410 proto native
void ClearAll();
411 proto native
void SetItem(
int item,
string value);
412 proto native
void RemoveItem(
int item);
413 proto native
int GetNumItems();
414 proto native
int SetCurrentItem(
int n);
415 proto native
int GetCurrentItem();
418 class CheckBoxWidget
extends UIWidget
420 proto native
void SetText(
string str);
421 proto native
bool IsChecked();
422 proto native
void SetChecked(
bool checked);
427 proto native
void ClearItems();
428 proto native
int GetNumItems();
429 proto native
void SelectRow(
int row);
430 proto native
int GetSelectedRow();
431 proto native
void RemoveRow(
int row);
432 proto native
void EnsureVisible(
int row);
442 proto native
int AddItem(
string text,
Class userData,
int column,
int row = -1);
443 proto native
void SetItem(
int position,
string text,
Class userData,
int column);
454 proto
bool GetItemText(
int row,
int column, out
string text);
455 proto
void GetItemData(
int row,
int column, out
Class data);
457 proto native
void SetItemColor(
int row,
int column,
int color );
460 class SpacerBaseWidget
extends UIWidget
481 class ScrollWidget
extends SpacerBaseWidget
542 class VideoWidget
extends Widget
545 proto native
bool Load(
string name,
bool looping =
false,
int startTime = 0);
547 proto native
void Unload();
550 proto native
bool Play();
552 proto native
bool Pause();
554 proto native
bool Stop();
557 proto native
bool SetTime(
int time,
bool preload);
561 proto native
int GetTotalTime();
564 proto native
void SetLooping(
bool looping);
566 proto native
bool IsLooping();
579 proto native
void DisableSubtitles(
bool disable);
581 proto native
bool IsSubtitlesDisabled();
622 bool LoadVideo(
string name,
int soundScene)
652 bool OnClick(
Widget w,
int x,
int y,
int button);
653 bool OnModalResult(
Widget w,
int x,
int y,
int code,
int result);
654 bool OnDoubleClick(
Widget w,
int x,
int y,
int button);
655 bool OnSelect(
Widget w,
int x,
int y);
656 bool OnItemSelected(
Widget w,
int x,
int y,
int row,
int column,
int oldRow,
int oldColumn);
657 bool OnFocus(
Widget w,
int x,
int y);
658 bool OnFocusLost(
Widget w,
int x,
int y);
659 bool OnMouseEnter(
Widget w,
int x,
int y);
661 bool OnMouseWheel(
Widget w,
int x,
int y,
int wheel);
662 bool OnMouseButtonDown(
Widget w,
int x,
int y,
int button);
663 bool OnMouseButtonUp(
Widget w,
int x,
int y,
int button);
665 bool OnController(
Widget w,
int control,
int value);
666 bool OnKeyDown(
Widget w,
int x,
int y,
int key);
667 bool OnKeyUp(
Widget w,
int x,
int y,
int key);
668 bool OnKeyPress(
Widget w,
int x,
int y,
int key);
669 bool OnChange(
Widget w,
int x,
int y,
bool finished);
670 bool OnDrag(
Widget w,
int x,
int y);
675 bool OnResize(
Widget w,
int x,
int y);
679 bool OnEvent(
EventType eventType,
Widget target,
int parameter0,
int parameter1);
706 proto native
bool IsCircleToCrossSwapped();
proto native int GetState()
returns one of STATE_...
ParticleSource Play(int particle_id, Object parent_obj, vector local_pos="0 0 0", vector local_ori="0 0 0")
Legacy function for backwards compatibility with 1.01 and below.
protected void Clear(bool clearFile=false)
proto native float GetMax()
void SetState(bool state)
proto native float GetMin()
bool IsPlaying()
Returns true when the Effect is playing, false otherwise.
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
int[] TypeID
script representation for C++ RTTI types
void SetTime(float time)
DEPRECATED.
Super root of all classes in Enforce script.
proto native void Load(string noise_name)
void Stop()
Stops all elements this effect consists of.