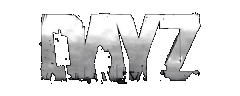 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
7 proto native owned
string GetClassName();
8 proto native owned
string GetName();
9 proto native
int VarIndex(
string varName);
10 proto native
bool IsVariableSet(
int varIndex);
11 proto
bool IsType(
int varIndex,
typename type);
12 proto
bool Get(
int varIndex, out
void val);
171 event protected void EOnTouch(
IEntity other,
int extra)
174 event protected void EOnInit(
IEntity other,
int extra)
177 event protected void EOnExtra(
IEntity other,
int extra)
180 event protected void EOnNotVisible(
IEntity other,
int extra)
183 event protected void EOnFrame(
IEntity other,
float timeSlice)
186 event protected int EOnVisible(
IEntity other,
int extra)
189 event protected void EOnPostFrame(
IEntity other,
int extra)
192 event protected void EOnWorldProcess(
IEntity other,
int extra)
201 event protected void EOnSimulate(
IEntity other,
float dt)
204 event protected void EOnPostSimulate(
IEntity other,
float timeSlice)
207 event protected void EOnJointBreak(
IEntity other,
int extra)
210 event protected void EOnPhysicsMove(
IEntity other,
int extra)
216 protected void EOnUser0(
IEntity other,
int extra)
219 protected void EOnUser1(
IEntity other,
int extra)
222 event protected void EOnEnter(
IEntity other,
int extra)
225 event protected void EOnLeave(
IEntity other,
int extra)
228 protected void EOnUser4(
IEntity other,
int extra)
231 protected void EOnDummy020(
IEntity other,
int extra)
234 protected void EOnDummy021(
IEntity other,
int extra)
237 protected void EOnDummy022(
IEntity other,
int extra)
240 protected void EOnDummy023(
IEntity other,
int extra)
243 protected void EOnDummy024(
IEntity other,
int extra)
246 protected void EOnDummy025(
IEntity other,
int extra)
249 protected void EOnDummy026(
IEntity other,
int extra)
252 protected void EOnDummy027(
IEntity other,
int extra)
255 protected void EOnDummy028(
IEntity other,
int extra)
258 protected void EOnDummy029(
IEntity other,
int extra)
261 protected void EOnDummy030(
IEntity other,
int extra)
264 protected void EOnDummy031(
IEntity other,
int extra)
288 proto external
void GetTransform(out
vector mat[]);
303 proto external
void GetRenderTransform(out
vector mat[]);
318 proto external
void GetLocalTransform(out
vector mat[]);
337 proto native external
vector GetTransformAxis(
int axis);
356 proto native external
void SetTransform(
vector mat[4]);
368 proto native external
vector GetOrigin();
380 proto external
vector GetLocalPosition();
392 proto native external
vector GetYawPitchRoll();
397 proto native external
vector GetAngles();
409 proto native external
vector GetLocalYawPitchRoll();
414 proto native external
vector GetLocalAngles();
427 proto native external
void SetYawPitchRoll(
vector angles);
432 proto native external
void SetAngles(
vector angles);
445 proto native external
void SetOrigin(
vector orig);
447 proto native external
float GetScale();
448 proto native external
void SetScale(
float scale);
460 proto native external
vector VectorToParent(
vector vec);
473 proto native external
vector CoordToParent(
vector coord);
499 proto native external
vector CoordToLocal(
vector coord);
518 proto native
int GetID();
531 proto native
void SetID(
int id);
533 proto native
void SetName(
string name);
534 proto native external owned
string GetName();
551 proto native external
bool AddChild(notnull
IEntity child,
int pivot,
bool positionOnly =
false);
560 proto native external
bool RemoveChild(notnull
IEntity child,
bool keepTransform =
false);
563 proto native
bool IsHierarchyPositionOnly();
566 proto native
int GetHierarchyPivot();
569 proto native
IEntity GetParent();
571 proto native
IEntity GetChildren();
573 proto native
IEntity GetSibling();
593 proto external
void GetBounds(out
vector mins, out
vector maxs);
612 proto external
void GetWorldBounds(out
vector mins, out
vector maxs);
641 proto native external
bool IsFlagSet(
EntityFlags flags);
719 proto external
volatile void SendEvent(notnull
IEntity actor,
EntityEvent e,
void extra);
735 proto native external
void SetObject(
vobject object,
string options);
740 proto native
vobject GetVObject();
743 proto native external
int Animate(
float speed,
int loop);
745 proto native external
int AnimateEx(
float speed,
int loop, out
vector lin, out
vector ang);
748 proto native external
int SetCameraMask(
int mask);
754 proto native external
void FilterNextTrace();
764 proto native external
int Update();
766 #ifdef COMPONENT_SYSTEM
774 proto native
void SetFixedLOD(
IEntity ent,
int lod);
778 proto native
void SetRenderView(
IEntity ent,
int cam_index,
int width,
int height);
779 proto
void GetRenderView(
IEntity ent, out
int cam_index, out
int width, out
int height);
794 void ParamEnum(
string key,
string value,
string desc =
"")
802 class ParamEnumArray:
array<ref ParamEnum>
806 ParamEnumArray params =
new ParamEnumArray();
807 int cnt = e.GetVariableCount();
810 for (
int i = 0; i < cnt; i++)
812 if (e.GetVariableType(i) ==
int && e.GetVariableValue(NULL, i, val))
814 params.Insert(
new ParamEnum(e.GetVariableName(i), val.ToString()));
829 ref ParamEnumArray m_Enums;
831 void Attribute(
string defvalue,
string uiwidget,
string desc =
"",
string rangescale =
"", ParamEnumArray enums = NULL)
833 m_DefValue = defvalue;
834 m_UiWidget = uiwidget;
835 m_RangeScale = rangescale;
854 void EditorAttribute(
string style,
string category,
string description,
vector sizeMin,
vector sizeMax,
string color,
string color2 =
"0 0 0 0",
bool visible =
true,
bool insertable =
true,
bool dynamicBox =
false)
@ USER1
Flags for custom usage and filterings.
@ TOUCHTRIGGERS
Interacts with triggers.
vector m_SizeMax
max vector of a bounding box
@ ENTER
Object entered Trigger.
ParamEnum Managed FromEnum(typename e)
string m_Description
class purpose description
@ TOUCH
entity was touched by other entity
@ SOLID
Is collidable by various trace methods.
@ SYNCHRONIZATION_DIRTY
Entity wants to synchronize (network)
void EditorAttribute(string style, string category, string description, vector sizeMin, vector sizeMax, string color, string color2="0 0 0 0", bool visible=true, bool insertable=true, bool dynamicBox=false)
string m_Category
folder structure eg. StaticEntities/Walls
class Attribute m_Style
can be "box", "sphere", "cylinder", "pyramid", "diamond" or custom style name
vector m_SizeMin
min vector of a bounding box
Result for an object found in CGame.IsBoxCollidingGeometryProxy.
@ LEAVE
Object left Trigger.
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
@ WATER
Used by tracing methods. Traceable only with flag TraceFlags.WATER.
void ParamEnum(string key, string value, string desc="")
@ STATIC
Static objects are included in the query.
@ FEATURE
Scene rendering hint for dominant objects that are not culled by standard way.
EntityEvent
Entity events for event-mask, or throwing event from code.
@ TRIGGER
Is not collidable, but invokes touch events.