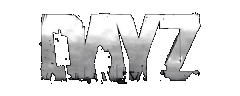 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
35 vector Frame[4] = {
Vector(1, 0, 0),
Vector(0, 1, 0),
Vector(0, 0, 1),
Vector(0, 0, 0)};
46 LayerMask = layerMask;
247 proto native
void dJointHingeSetLimits(
dJoint joint,
float low,
float high,
float softness,
float biasFactor,
float relaxationFactor);
265 proto native
void dJointConeTwistSetLimits(
dJoint joint,
float _swingSpan1,
float _swingSpan2,
float _twistSpan,
float _softness,
float _biasFactor,
float _relaxationFactor);
312 float PenetrationDepth;
315 float RelativeNormalVelocityBefore;
316 float RelativeNormalVelocityAfter;
320 vector RelativeVelocityBefore;
321 vector RelativeVelocityAfter;
323 proto native
vector GetNormalImpulse();
324 proto native
float GetRelativeVelocityBefore(
vector vel);
325 proto native
float GetRelativeVelocityAfter(
vector vel);
proto native void dSetTimeSlice(notnull IEntity worldEntity, float timeSlice)
Changes fixed time-slice. Default is 1/40, thus simulation runs on 40fps. With smaller values,...
proto native void dBodySetInertiaTensorV(notnull IEntity body, vector v)
proto native void SetVelocity(notnull IEntity ent, vector vel)
Sets linear velocity (for Rigid bodies)
proto native void dJointConeTwistSetLimits(dJoint joint, float _swingSpan1, float _swingSpan2, float _twistSpan, float _softness, float _biasFactor, float _relaxationFactor)
proto native int dBodyGetInteractionLayer(notnull IEntity ent)
proto native void dJointSliderSetAngularLimits(dJoint joint, float lowerLimit, float upperLimit)
proto native float dBodyComputeAngularImpulseDenominator(notnull IEntity ent, vector axis)
proto native void dBodyApplyTorque(notnull IEntity body, vector torque)
proto native float dJointSliderGetAngularPos(dJoint joint)
proto native float dBodyGetKineticEnergy(notnull IEntity body)
proto native void dSetGravity(notnull IEntity worldEntity, vector g)
Changes global gravity.
proto native vector dBodyGetCenterOfMass(notnull IEntity body)
returns center of mass offset
proto native void dBodyEnableCCD(notnull IEntity body, float maxMotion, float sphereCastRadius)
proto native dJoint dJointCreateFixed(notnull IEntity ent1, notnull IEntity ent2, vector point1, vector point2, bool block, float breakThreshold)
proto native float dBodyGetMass(notnull IEntity ent)
proto native dJoint dJointCreate6DOFSpring(notnull IEntity ent1, notnull IEntity ent2, vector matrix1[4], vector matrix2[4], bool block, float breakThreshold)
proto native bool dBodyEnableGravity(notnull IEntity ent, bool enable)
proto void dBodyApplyImpulse(notnull IEntity body, vector impulse)
Applies impuls on a rigidbody (origin)
proto native float dBodyComputeImpulseDenominator(notnull IEntity ent, vector position, vector normal)
proto native void dJointSliderSetLimLinear(dJoint joint, float softness, float restitution, float damping)
proto native dJoint dJointCreateHinge(notnull IEntity ent1, notnull IEntity ent2, vector point1, vector axis1, vector point2, vector axis2, bool block, float breakThreshold)
ActiveState
state of a rigidbody
proto bool dBodyCreateGhostEx(notnull IEntity ent, PhysicsGeomDef geoms[])
proto native void dBodySetInertiaTensorM(notnull IEntity body, vector m[3])
proto native void dBodyActive(notnull IEntity ent, ActiveState activeState)
proto native void dBodyDestroy(notnull IEntity ent)
Destroys attached physics body.
proto native void dBodySetSolid(notnull IEntity ent, bool solid)
proto native vector dBodyGetLocalInertia(notnull IEntity ent)
proto void dBodyApplyImpulseAt(notnull IEntity body, vector impulse, vector pos)
Applies impuls on a pos position in world coordinates.
proto native void dBodyApplyTorqueImpulse(notnull IEntity ent, vector torqueImpulse)
proto native dJoint dJointCreateSlider(notnull IEntity ent1, notnull IEntity ent2, vector matrix1[4], vector matrix2[4], bool block, float breakThreshold)
proto void dBodySetAngularVelocity(notnull IEntity body, vector angvel)
Changed an angular velocity.
proto native dBlock dBodyCollisionBlock(notnull IEntity ent1, notnull IEntity ent2)
Disables collisions between two entities.
proto native void dBodySetGeomInteractionLayer(notnull IEntity ent, int index, int mask)
proto native dJoint dJointCreateConeTwist(notnull IEntity ent1, notnull IEntity ent2, vector matrix1[4], vector matrix2[4], bool block, float breakThreshold)
proto native void dJoint6DOFSetAngularLimits(dJoint joint, vector angularLower, vector angularUpper)
proto native void dJointSliderSetOrthoLinear(dJoint joint, float softness, float restitution, float damping)
proto native dJoint dJointCreate6DOF(notnull IEntity ent1, notnull IEntity ent2, vector matrix1[4], vector matrix2[4], bool block, float breakThreshold)
proto native void dBodySetTargetMatrix(notnull IEntity body, vector matrix[4], float timeslice)
Sets target transformation. If timeslice == dt (simulation step delta time), it will happen in next s...
proto native void dBodySetLinearFactor(notnull IEntity body, vector linearFactor)
proto native dJoint dJointCreateHinge2(notnull IEntity ent1, notnull IEntity ent2, vector matrix1[4], vector matrix2[4], bool block, float breakThreshold)
proto native void dJointHingeSetMotorTargetAngle(dJoint joint, float angle, float dt, float maxImpulse)
proto native void dSetInteractionLayer(notnull IEntity worldEntity, int mask1, int mask2, bool enable)
proto native int dBodyGetGeom(notnull IEntity ent, string name)
proto native void dJointSliderSetOrthoAngular(dJoint joint, float softness, float restitution, float damping)
proto native float dJointSliderGetLinearPos(dJoint joint)
proto native void dJointHingeSetLimits(dJoint joint, float low, float high, float softness, float biasFactor, float relaxationFactor)
proto bool dBodyCreateDynamicEx(notnull IEntity ent, vector centerOfMass, float mass, PhysicsGeomDef geoms[])
proto native void dJointSliderSetLinearMotor(dJoint joint, float velocity, float force)
proto native void dBodyDynamic(notnull IEntity ent, bool dynamic)
proto native bool dBodyIsSet(notnull IEntity ent)
Has the entity attached physics body?
proto native void dJointConeTwistSetAngularOnly(dJoint joint, bool angularOnly)
proto void dBodyGetInvInertiaTensorWorld(notnull IEntity body, out vector inertiaTensorWS[3])
proto native void dBodyRemoveBlock(notnull IEntity worldEntity, dBlock block)
proto native vector dBodyGetInvInertiaDiagLocal(notnull IEntity ent)
proto native void dJoint6DOFSpringSetSpring(dJoint joint, int axis, float stiffness, float damping)
owned string MaterialName
proto native void dBodySetDamping(notnull IEntity ent, float linearDamping, float angularDamping)
proto native bool dBodyIsDynamic(notnull IEntity ent)
proto native void dJointDestroy(dJoint joint)
proto native void dJointSliderSetLimAngular(dJoint joint, float softness, float restitution, float damping)
proto native bool dBodyIsActive(notnull IEntity ent)
proto native void dJointSliderSetDirLinear(dJoint joint, float softness, float restitution, float damping)
proto native void dJoint6DOFSetLinearLimits(dJoint joint, vector linearLower, vector linearUpper)
proto native void dBodySetMass(notnull IEntity body, float mass)
proto native IEntity dGetDynamicBody(notnull IEntity worldEnt, int index)
proto native int dGetNumDynamicBodies(notnull IEntity worldEnt)
proto vector dBodyGetAngularVelocity(notnull IEntity body)
Gets angular velocity for a rigidbody.
proto native void dBodySetSleepingTreshold(notnull IEntity body, float linearTreshold, float angularTreshold)
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
proto native vector dGetGravity(notnull IEntity worldEntity)
Gets global gravity.
proto native bool dGetInteractionLayer(notnull IEntity worldEntity, int mask1, int mask2)
proto native void dJoint6DOFSetLimit(dJoint joint, int axis, float lo, float hi)
proto native bool dBodyIsSolid(notnull IEntity ent)
proto native dGeom dGeomCreateBox(vector size)
Creates box geometry.
proto bool dBodyCreateStaticEx(notnull IEntity ent, PhysicsGeomDef geoms[])
proto native void dJointSliderSetAngularMotor(dJoint joint, float velocity, float force)
proto native void dBodySetInteractionLayer(notnull IEntity ent, int mask)
proto native dGeom dGeomCreateSphere(float radius)
Creates sphere geometry.
proto native void dJointConeTwistSetLimit(dJoint joint, int limitIndex, float limitValue)
proto native vector dBodyGetVelocityAt(notnull IEntity body, vector globalpos)
proto void dBodyApplyForce(notnull IEntity body, vector force)
Applies constant force on a rigidbody (origin)
proto native dGeom dGeomCreateCylinder(float radius, vector extent)
Creates cylinder geometry.
proto native void dJointSliderSetDirAngular(dJoint joint, float softness, float restitution, float damping)
proto void dBodyApplyForceAt(notnull IEntity body, vector pos, vector force)
Applies constant force on a position.
proto native int dBodyGetGeomInteractionLayer(notnull IEntity ent, int index)
proto native void dJointHingeSetAxis(dJoint joint, vector axis)
proto native int dBodyGetNumGeoms(notnull IEntity ent)
proto native vector GetVelocity(notnull IEntity ent)
Returns linear velocity.
proto native dGeom dGeomCreateCapsule(float radius, vector extent)
Creates capsule geometry.
proto native vector Vector(float x, float y, float z)
Vector constructor from components.
proto native void dJointSliderSetLinearLimits(dJoint joint, float lowerLimit, float upperLimit)
proto native void dGeomDestroy(dGeom geom)
Destroys geometry.
proto native dJoint dJointCreateBallSocket(notnull IEntity ent1, notnull IEntity ent2, vector point1, vector point2, bool block, float breakThreshold)