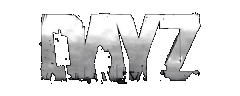 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
202 owned
string MaterialName;
203 owned
string OriginalMaterialName;
216 int LayerMask = 0xffffffff;
297 proto native
int GetResult();
300 proto native
void SetPosition(
vector pos);
302 proto native
void Destroy();
PostProcessEffectType
Post-process effect type.
proto native void FinalizeLandMarkDecal(hDecal lmDecal, bool addAlpha, float alphaDist)
proto native int CanAddToLandMarkDecal(hDecal lmDecal, IEntity entity, string mat, vector newPoint)
proto native hDecal CreateLandMarkDecal(IEntity entity, vector origin, vector normal, float edgeSize, float lifeTime, string materialName, hDecal prevDecal, float alpha)
proto native bool SetLightEx(HLIGHT light, float radius, vector color)
int[] HLIGHT
Light handle.
proto vector ProjectVector(int cam, IEntity ent, vector vec)
proto native IEntity FindEntityByName(IEntity worldEnt, string name)
proto native bool SetLightTexture(HLIGHT light, string cubemap)
sets lookup texture for projection lights
proto native int SetLightFlags(HLIGHT light, LightFlags flags)
proto native int P2PVisibilityEx(vector from, vector to, int flags)
proto native hDecal CreateDecal(IEntity entity, vector origin, vector project, float nearclip, float angle, float size, string materialName, float lifetime, int flags)
proto int VisEntities(vector origin, vector look, float angle, float radius, out IEntity ents[2], int maxents, int fmask)
proto native void SetCamera(int cam, vector origin, vector angle)
proto native float GetSceneHDRMul(int camera)
proto vector UnprojectVector(int cam, float x, float y, vector dir)
@ CHEAP
for cheaper dynamic lights, like muzzle flashes (might use cheaper rendering method)
proto native IEntity FindEntityByID(IEntity worldEnt, int ID)
proto native void SetCameraVerticalFOV(int cam, float fovy)
proto native int GetNumActiveEntities(IEntity worldEntity)
returns number of active (simulated) Entities in the world
proto native float GetWorldTime()
proto native WorldHandle SetCurrentWorld(WorldHandle world)
proto native void GetCamera(int cam, out vector mat[4])
Returns current camera transformation.
proto native int ClearLightFlags(HLIGHT light, LightFlags flags)
proto native float GetOceanHeight(float worldX, float worldZ)
proto native bool AddPointToLandMarkDecal(hDecal lmDecal, vector point, vector normal, float alpha)
proto native vector GetLastLandMarkPoint(hDecal lmDecal)
proto native vector GetOceanHeightAndDisplace(float worldX, float worldZ)
proto native bool RemoveLight(HLIGHT light)
removes light
proto volatile float TraceMove(TraceParam param, out IEntity cent, out float plane[4], out int surfparm, func filtercallback)
proto native bool IsOcean()
proto native void SetCameraFarPlane(int cam, float farplane)
proto native void SetCameraPostProcessEffect(int cam, int priority, PostProcessEffectType type, string materialPath)
@ DYNAMIC
Dynamic objects are included in the query.
TraceParam TraceLineToEntity
proto HLIGHT AddLight(IEntity owner, LightType type, LightFlags flags, float radius, vector color)
proto native IEntity GetActiveEntity(IEntity worldEntity, int index)
returns active entity
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
proto native void SetCameraType(int cam, CameraType type)
proto native void SetCameraEx(int cam, const vector mat[4])
Changes camera matrix.
proto native bool IsBoxVisible(vector mins, vector maxs, int flags)
proto native bool IsLandMarkFinalized(hDecal lmDecal)
proto native void SetListenerCamera(int camera)
sets which camera will be a listener (for sound engine)
proto native void SetGlobalLandMarkParams(float minSegmentLength, float maxSegmentLength, float degAngle)
proto native void RemoveDecal(hDecal decal)
proto int SphereQuery(vector origin, float radius, out IEntity visents[], int ents, int fmask)
finds all entities in a radius
proto native void SetCameraNearPlane(int cam, float nearplane)
proto native bool SetLightCone(HLIGHT light, float cone)
Sets light cone in degrees (for LightType.SPOT).