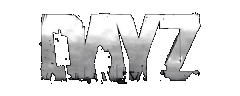 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
82 proto
volatile native
void EndSound(HSOUND snd);
86 proto native
int SetSoundFrequency(HSOUND sound,
int freq)
90 proto native
int GetSoundLength(HSOUND sound)
93 proto native
int GetSoundPosition(HSOUND sound)
104 class PacketOutputAdapter
107 proto native
void WriteInt(
int value);
122 proto native
bool ReadBool();
123 proto native
int ReadInt();
124 proto native
float ReadFloat();
125 proto
string ReadString();
126 proto native
vector ReadVector();
127 proto
void ReadMatrixAsQuaternionVector(
vector mat[4]);
128 proto native
int ReadIntAsByte();
129 proto native
int ReadIntAsUByte();
130 proto native
int ReadIntAsHalf();
131 proto native
int ReadIntAsUHalf();
132 proto native
float ReadFloatAsByte(
float min,
float max);
133 proto native
float ReadFloatAsHalf(
float min,
float max);
147 proto native
int GetFPS();
163 proto native
int InitSky(
string presetName);
171 proto native
int SetSkyPreset(
string presetName,
float stormy,
float dayTime);
182 proto native
int LerpSkyPreset(
string presetName1,
string presetName2,
float dayTime,
float stormy1,
float stormy2,
float lerpVal);
197 proto native
int LerpSkyPreset3(
string presetName1,
string presetName2,
string presetName3,
float dayTime,
float stormy1,
float stormy2,
float stormy3,
float w1,
float w2,
float w3);
212 proto native
bool SetSkyPlanet(
int index,
float azimuthDeg,
float zenithDeg);
231 proto native
void SetStarsObserverTime(
int year,
int month,
int day,
int hour,
int minute,
float sec,
int offsetSec);
274 proto
bool SetParam(
string propertyName,
void value);
280 proto native
void ResetParam(
string propertyName);
287 proto native
int GetParamIndex(
string paramName);
294 proto
void SetParamByIndex(
int paramIndex,
void value);
307 x =
x * 127.0 + 128.0;
308 y =
y * 127.0 + 128.0;
309 z = z * 127.0 + 128.0;
317 return r | g | b | a;
322 int ARGB(
int a,
int r,
int g,
int b)
327 return a | r | g | b;
332 int ARGBF(
float fa,
float fr,
float fg,
float fb)
334 return ARGB((
float)(fa * 255.0), (
float)(fr * 255.0), (
float)(fg * 255.0), (
float)(fb * 255.0));
340 return a << 24 | 0xffffff;
347 const int cmask = 0x00ff00ff;
349 cb1 = c1 >> 8 & cmask;
350 cb2 = c2 >> 8 & cmask;
351 cb1 = cb1 + cb2 >> 1;
357 return cb1 << 8 | c1;
363 proto
private native
void Init(T
init);
private void Release(vector pos)
proto native void WriteInt(int value)
int LerpARGB(int c1, int c2)
proto native void WriteIntAsUByte(int value)
proto native void SetSoundVolume(float vol, float time)
proto native int LerpSkyPreset3(string presetName1, string presetName2, string presetName3, float dayTime, float stormy1, float stormy2, float stormy3, float w1, float w2, float w3)
proto native void SetNightLayerRotMatrix(vector mat[3])
proto native int GetFPS()
proto native bool SetSkyPlanet(int index, float azimuthDeg, float zenithDeg)
proto native int SetSkyPreset(string presetName, float stormy, float dayTime)
proto native int InitSky(string presetName)
proto native void SetRealStarAutoUpdate(bool update)
proto native void WriteIntAsHalf(int value)
proto native void WriteVector(vector value)
proto native void SetStarsObserverTime(int year, int month, int day, int hour, int minute, float sec, int offsetSec)
class InventoryGridController extends ScriptedWidgetEventHandler Init
proto native void WriteIntAsUHalf(int value)
proto native void SetStarsObserverPosition(float latitudeDeg, float longitudeDeg)
proto native void SetSkyUserPlanets(bool enabled)
proto native void WriteIntAsByte(int value)
proto native void SetStarsRotMatrix(vector mat[3])
array< ref PlayerStatBase > Get()
int ARGBF(float fa, float fr, float fg, float fb)
Converts <0.0, 1.0> ARGB into color.
int VectortoRGBA(vector vec, float h)
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
enum MagnumStableStateID init
proto native void WriteFloatAsByte(float value, float min, float max)
proto native void WriteMatrixAsQuaternionVector(vector mat[4])
proto native int LerpSkyPreset(string presetName1, string presetName2, float dayTime, float stormy1, float stormy2, float lerpVal)
proto native bool SetSkyPlanetSize(int index, float angleDeg)
proto native void WriteFloatAsHalf(float value, float min, float max)
Super root of all classes in Enforce script.
proto native int LoadSkyPresets(string presetsFile)
proto native void WriteString(string value)
proto native void MakeScreenshot(string name)
proto native void WriteFloat(float value)
int ARGB(int a, int r, int g, int b)
class PacketInputAdapter WriteBool