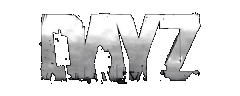 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
68 proto
bool WriteToString(
void variable_out,
bool nice, out
string result);
100 proto
bool ReadFromString(
void variable_in,
string jsonString, out
string error);
109 proto native
void Reset();
117 proto native
void Send(
Object target,
int rpc_type,
bool guaranteed,
PlayerIdentity recipient = NULL);
125 proto native
void Reset ();
126 proto native
void Send ();
130 proto native
static bool CanStoreInputUserData ();
147 proto native
void Reset ();
155 proto native
void Reset ();
161 proto native
int GetModesCount();
163 proto native owned
string GetModeName(
int index);
165 proto native owned
string GetAmmoTypeName(
int index);
167 proto native
float GetModeRange(
int index);
183 proto native owned
string GetName();
184 proto native
int GetVertexCount();
185 proto native
int GetLODVertexIndex(
int sel_vertex_index);
187 vector GetVertexPosition(
LOD lod,
int index)
189 int lodIndex = GetLODVertexIndex(index);
192 Error(
"Vertex doesn't exist");
196 return lod.GetVertexPosition(lodIndex);
205 static const string NAME_GEOMETRY =
"geometry";
206 static const string NAME_VIEW =
"view";
207 static const string NAME_FIRE =
"fire";
208 static const string NAME_MEMORY =
"memory";
209 static const string NAME_ROADWAY =
"roadway";
211 private void LOD() {}
212 private void ~
LOD() {}
214 proto native
int GetSelectionCount();
217 proto native
vector GetVertexPosition(
int vertex_index);
219 proto native owned
string GetName(
Object myObject);
224 GetSelections(selections);
226 for (
int i = 0; i < selections.Count(); ++i)
228 string selection_name = selections.Get(i).GetName();
229 selection_name.ToLower();
231 if (selection_name ==
name)
233 return selections.Get(i);
240 proto native
int GetPropertyCount();
241 proto native owned
string GetPropertyName(
int index);
242 proto native owned
string GetPropertyValue(
int index);
245 class Plant
extends Object
268 class ProxySubpart
extends Entity
277 proto native
void SetItem(
EntityAI object);
280 proto native
int GetView();
287 proto native
void SetView(
int viewIndex);
289 proto native
void SetModelOrientation(
vector vOrientation);
290 proto native
vector GetModelOrientation();
291 proto native
void SetModelPosition(
vector vPos);
292 proto native
vector GetModelPosition();
294 proto native
void SetForceFlipEnable(
bool enable);
295 proto native
void SetForceFlip(
bool value);
301 proto native
void UpdateItemInHands(
EntityAI object);
302 proto native
void SetPlayer(
DayZPlayer player);
306 proto native
void Refresh();
308 proto native
void SetModelOrientation(
vector vOrientation);
309 proto native
vector GetModelOrientation();
310 proto native
void SetModelPosition(
vector vPos);
311 proto native
vector GetModelPosition();
317 proto native
void LoadFile(
string path);
323 proto native
void ClearUserMarks();
324 proto native
void AddUserMark(
vector pos,
string text,
int color ,
string texturePath);
325 proto native
vector GetMapPos();
326 proto native
void SetMapPos(
vector worldPos);
327 proto native
float GetScale();
328 proto native
void SetScale(
float scale);
329 proto native
float GetContourInterval();
330 proto native
float GetCellSize(
float pLegendWidth);
340 proto
int GetPingAct();
342 proto
int GetPingMin();
344 proto
int GetPingMax();
346 proto
int GetPingAvg();
349 proto
int GetBandwidthMin();
351 proto
int GetBandwidthMax();
353 proto
int GetBandwidthAvg();
356 proto
string GetName();
358 proto
string GetPlainName();
360 proto
string GetFullName();
362 proto
string GetId();
364 proto
string GetPlainId();
366 proto
int GetPlayerId();
369 proto Man GetPlayer();
577 const string None =
"";
578 const string Cursor =
"set:dayz_gui image:cursor";
579 const string CloseDoors =
"set:dayz_gui image:close";
580 const string OpenDoors =
"set:dayz_gui image:open";
581 const string OpenCarDoors =
"set:dayz_gui image:open_car";
582 const string CloseCarDoors =
"set:dayz_gui image:close_car";
583 const string EngineOff =
"set:dayz_gui image:engine_off";
584 const string EngineOn =
"set:dayz_gui image:engine_on";
585 const string LadderDown =
"set:dayz_gui image:ladderdown";
586 const string LadderOff =
"set:dayz_gui image:ladderoff";
587 const string LadderUp =
"set:dayz_gui image:ladderup";
588 const string LootCorpse =
"set:dayz_gui image:gear";
589 const string CloseHood =
"set:dayz_gui image:close_hood";
590 const string OpenHood =
"set:dayz_gui image:open_hood";
591 const string GetOut =
"set:dayz_gui image:getout";
592 const string GetInCargo =
"set:dayz_gui image:get_in_cargo";
593 const string Reload =
"set:dayz_gui image:reload";
594 const string GetInDriver =
"set:dayz_gui image:get_in_driver";
595 const string GetInCommander =
"set:dayz_gui image:get_in_commander";
596 const string GetInPilot =
"set:dayz_gui image:get_in_pilot";
597 const string GetInGunner =
"set:dayz_gui image:get_in_gunner";
626 void Init(
Widget hud_panel_widget) {}
627 void DisplayNotifier(
int key,
int tendency,
int status) {}
628 void DisplayBadge(
int key,
int value) {}
629 void SetStamina(
int value,
int range) {}
630 void DisplayStance(
int stance) {}
631 void DisplayPresence() {}
632 void ShowCursor() { }
633 void HideCursor() { }
634 void SetCursorIcon(
string icon) { }
635 void SetCursorIconScale(
string type,
float percentage) { }
636 void SetCursorIconOffset(
string type,
float x,
float y) { }
637 void SetCursorIconSize(
string type,
float x,
float y) { }
638 void ShowWalkieTalkie(
bool show) { }
639 void ShowWalkieTalkie(
int fadeOutSeconds) { }
640 void SetWalkieTalkieText(
string text) { }
641 void RefreshQuickbar(
bool itemChanged =
false) {}
642 void Show(
bool show) {}
643 void UpdateBloodName() {}
644 void SetTemperature(
string temp);
645 void SetStaminaBarVisibility(
bool show);
646 void Update(
float timeslice){}
647 void ShowVehicleInfo();
648 void HideVehicleInfo();
649 void ToggleHeatBufferPlusSign(
bool show);
651 void ShowQuickbarUI(
bool show);
652 void ShowQuickbarPlayer(
bool show);
653 void ShowHudPlayer(
bool show);
654 void ShowHudUI(
bool show);
655 void ShowHudInventory(
bool show);
656 void ShowQuickBar(
bool show);
657 void UpdateQuickbarGlobalVisibility();
658 void ShowHud(
bool show);
660 void OnResizeScreen();
662 void SetPermanentCrossHair(
bool show) {}
664 void SpawnHitDirEffect(
DayZPlayer player,
float hit_direction,
float intensity_max);
685 void OnMissionStart() {}
686 void OnMissionFinish() {}
687 void OnUpdate(
float timeslice)
689 #ifdef FEATURE_CURSOR
693 void OnKeyPress(
int key) {}
694 void OnKeyRelease(
int key) {}
695 void OnMouseButtonPress(
int button){}
696 void OnMouseButtonRelease(
int button){}
699 void AddDummyPlayerToScheduler(Man player){}
702 void OnGameplayDataHandlerLoad(){}
709 ObjectSnapCallback GetInventoryDropCallback()
714 bool IsPlayerDisconnecting(Man player);
754 void AbortMission() {}
757 void StartLogoutMenu(
int time) {}
759 void CreateDebugMonitor() {}
760 void HideDebugMonitor() {}
762 void RefreshCrosshairVisibility() {}
764 void HideCrosshairVisibility() {}
766 bool IsMissionGameplay()
771 bool IsControlDisabled() {}
772 int GetControlDisabledMode() {}
774 void PlayerControlEnable(
bool bForceSupress);
775 void PlayerControlDisable(
int mode);
777 void RemoveActiveInputExcludes(
array<string> excludes,
bool bForceSupress =
false);
778 void RemoveActiveInputRestriction(
int restrictor);
780 void AddActiveInputRestriction(
int restrictor);
781 void RefreshExcludes();
782 bool IsInputExcludeActive(
string exclude);
783 bool IsInputRestrictionActive(
int restriction);
784 void EnableAllInputs(
bool bForceSupress =
false);
786 void ShowInventory() {}
787 void HideInventory() {}
791 void UpdateVoiceLevelWidgets(
int level) {}
793 void HideVoiceLevelWidgets() {}
794 bool IsVoNActive() {}
795 void SetVoNActive(
bool active) {}
797 bool InsertCorpse(Man player)
804 void SetPlayerRespawning(
bool state);
805 void OnPlayerRespawned(Man player);
806 bool IsPlayerRespawning();
810 void SetRespawnModeClient(
int mode);
811 int GetRespawnModeClient()
818 ImageWidget GetMicrophoneIcon()
823 WidgetFadeTimer GetMicWidgetFadeTimer() {}
833 if (!m_OnInputDeviceChanged)
838 return m_OnInputDeviceChanged;
843 if (!m_OnInputPresetChanged)
848 return m_OnInputPresetChanged;
853 if (!m_OnInputDeviceConnected)
858 return m_OnInputDeviceConnected;
863 if (!m_OnInputDeviceDisconnected)
868 return m_OnInputDeviceDisconnected;
873 if (!m_OnModMenuVisibilityChanged)
878 return m_OnModMenuVisibilityChanged;
881 #ifdef FEATURE_CURSOR
883 int GetTimeStamp() {
return m_TimeStamp; }
887 bool m_SuppressNextFrame =
true;
888 void SetInputSuppression(
bool state) {}
889 bool GetInputSuppression() {}
897 proto native
int GetCharactersCount();
898 proto native
int GetLastPlayedCharacter();
900 proto native Man CreateCharacterPerson(
int index);
902 proto
void GetLastServerAddress(
int index, out
string address);
903 proto native
int GetLastServerPort(
int index);
904 proto
void GetLastServerName(
int index, out
string address);
906 proto
void RequestSetDefaultCharacterData();
907 proto
bool RequestGetDefaultCharacterData();
912 if (!GetMenuDefaultCharacterDataInstance())
914 Error(
"MenuData | OnSetDefaultCharacter - failed to get data class!");
918 GetMenuDefaultCharacterDataInstance().SerializeCharacterData(ctx);
919 SaveCharactersLocal();
925 if (!GetMenuDefaultCharacterDataInstance())
927 Error(
"MenuData | OnGetDefaultCharacter - failed to get data class!");
931 if (GetMenuDefaultCharacterDataInstance().DeserializeCharacterData(ctx))
938 proto
void GetCharacterName(
int index, out
string name);
939 proto native
void SetCharacterName(
int index,
string newName);
941 proto native
void SaveCharacter(
bool localPlayer,
bool verified);
942 proto native
void SaveDefaultCharacter(Man character);
945 proto native
void SaveCharactersLocal();
947 proto native
void LoadCharactersLocal();
948 proto native
void ClearCharacters();
952 return GetGame().GetMenuDefaultCharacterData();
965 string m_CharacterName;
966 string m_CharacterType;
968 bool m_ForceRandomCharacter;
977 if (!
GetGame().IsDedicatedServer())
979 GetGame().GetMenuData().LoadCharactersLocal();
984 void ClearAttachmentsMap()
986 m_AttachmentsMap.Clear();
989 void SetDefaultAttachment(
int slotID,
string type)
991 m_AttachmentsMap.Set(slotID,type);
994 void GenerateRandomEquip()
996 ClearAttachmentsMap();
999 string attachment_type;
1000 for (
int i = 0; i < DefaultCharacterCreationMethods.GetAttachmentSlotsArray().
Count(); i++)
1002 slot_ID = DefaultCharacterCreationMethods.GetAttachmentSlotsArray().Get(i);
1003 if (DefaultCharacterCreationMethods.GetConfigArrayCountFromSlotID(slot_ID) > 0)
1005 attachment_type = DefaultCharacterCreationMethods.GetConfigAttachmentTypes(slot_ID).GetRandomElement();
1007 SetDefaultAttachment(slot_ID,attachment_type);
1012 void EquipDefaultCharacter(Man player)
1016 ErrorEx(
"WARNING - trying to equip non-existent object! | MenuDefaultCharacterData::EquipDefaultCharacter");
1021 string attachment_type;
1022 string current_attachment_type;
1023 EntityAI current_attachment_object;
1025 for (
int i = 0; i < m_AttachmentsMap.Count(); i++)
1027 attachment_type =
"";
1028 current_attachment_type =
"";
1029 slot_ID = m_AttachmentsMap.GetKey(i);
1030 attachment_type = m_AttachmentsMap.GetElement(i);
1031 current_attachment_object = player.GetInventory().FindAttachment(slot_ID);
1033 if (current_attachment_object)
1035 current_attachment_type = current_attachment_object.GetType();
1037 if (current_attachment_type != attachment_type)
1039 if (current_attachment_object)
1040 g_Game.ObjectDelete(current_attachment_object);
1041 if (attachment_type !=
"")
1042 player.GetInventory().CreateAttachmentEx(attachment_type,slot_ID);
1050 ctx.Write(m_CharacterType);
1051 ctx.Write(m_AttachmentsMap);
1052 ctx.Write(m_ForceRandomCharacter);
1053 ctx.Write(m_CharacterName);
1059 if (!ctx.Read(m_CharacterType))
1061 if (!ctx.Read(m_AttachmentsMap))
1063 if (!ctx.Read(m_ForceRandomCharacter))
1065 if (!ctx.Read(m_CharacterName))
1072 void SetCharacterName(
string name)
1074 m_CharacterName =
name;
1077 string GetCharacterName()
1079 return m_CharacterName;
1082 void SetCharacterType(
string character_type)
1084 m_CharacterType = character_type;
1087 string GetCharacterType()
1089 return m_CharacterType;
1092 void SetRandomCharacterForced(
bool state)
1094 m_ForceRandomCharacter = state;
1097 bool IsRandomCharacterForced()
1099 return m_ForceRandomCharacter;
1104 return m_AttachmentsMap;
1108 void DumpAttMapContents()
1112 Print(
"-----------");
1113 Print(
"m_AttachmentsMap contents:");
1114 for (
int j = 0; j < m_AttachmentsMap.Count(); j++)
1116 debugID = m_AttachmentsMap.GetKey(j);
1117 debugType = m_AttachmentsMap.GetElement(j);
1118 Print(
"index " + j);
1119 Print(
"debugID: " + debugID);
1120 Print(
"debugType: " + debugType);
1122 Print(
"-----------");
1126 class DefaultCharacterCreationMethods
1160 const static string m_Path =
"cfgCharacterCreation";
1163 static string GetPathFromSlotID(
int slot_ID)
1166 string path =
"" + m_Path +
" " + m_ConfigArrayNames.Get(idx);
1171 static int GetConfigArrayCountFromSlotID(
int slot_ID)
1174 GetGame().ConfigGetTextArray(GetPathFromSlotID(slot_ID),types);
1175 return types.Count();
1182 GetGame().ConfigGetTextArray(GetPathFromSlotID(slot_ID),types);
1195 return m_ConfigArrayNames;
1299 proto native
int GetAccessType();
1305 proto native
int GetControlType();
1310 proto native
void Apply();
1315 proto native
void Test();
1320 proto native
void Revert();
1326 proto native
int IsChanged();
1332 proto native
int NeedRestart();
1338 proto native
int SetChangeImmediately();
1350 private proto
void SetScriptEvents(
Managed events);
1356 private proto
Managed GetScriptEvents();
1378 GetEvents().Event_OnRevert.Invoke(
this);
1395 proto native
float GetMin();
1396 proto native
float GetMax();
1403 proto native
int GetIndex();
1404 proto native
int GetDefaultIndex();
1405 proto native
void SetIndex(
int index);
1406 proto native
int GetItemsCount();
1407 proto
void GetItemText(
int index, out
string value);
1413 proto native
void Switch();
1414 proto
void GetItemText(out
string value);
1415 proto native
int GetIndex();
1416 proto native
int GetDefaultIndex();
1425 proto native
void Apply();
1430 proto native
void Revert();
1435 proto native
void Test();
1455 proto native
int GetOptionsCount();
1461 proto native
int NeedRestart();
1467 proto native
int IsChanged();
1472 proto native
void Initialize();
1488 return GameInventory.LocationCreateEntity(inv_loc, object_name,iSetupFlags,iRotation);
1498 static bool m_GunParticlesState =
true;
proto native CGame GetGame()
const EventType ClientReadyEventTypeID
params: ClientReadyEventParams
const EventType DLCOwnerShipFailedEventTypeID
params: DLCOwnerShipFailedParams
OptionIDsScript
Used for script-based game options. For anything C++ based, you would most likely use "Option Access ...
@ AT_OPTIONS_GAMMA_SLIDER
Param2< string, string > VONStartSpeakingEventParams
player name, player id
const EventType RespawnEventTypeID
params: RespawnEventParams
void Error(string err)
Messagebox with error message.
const EventType VONUserStoppedTransmittingAudioEventTypeID
no params
Invokers for ParticleManager events.
Base Param Class with no parameters. Used as general purpose parameter overloaded with Param1 to Para...
@ AT_OPTIONS_MOUSE_XAXIS_AIM_MOD
const EventType NetworkManagerClientEventTypeID
no params
Param1< int > SQFConsoleEventParams
provides access to slot configuration
Param2< int, string > MPConnectionCloseEventParams
EClientKicked, AdditionalInfo.
const int INDEX_NOT_FOUND
PlayerIdentity PROGRESS_START
@ AT_OPTIONS_DRAWDISTANCE_SLIDER
const EventType VONUserStartedTransmittingAudioEventTypeID
no params
array< string > TStringArray
Param4< PlayerIdentity, Man, int, bool > ClientDisconnectedEventParams
PlayerIdentity, Man, LogoutTime, AuthFailed.
@ AT_OPTIONS_SHADOW_VISIBILITY_SLIDER
const EventType ClientNewReadyEventTypeID
params: ClientNewReadyEventParams
const EventType PartyChatStatusChangedEventTypeID
no params
class MeleeCombatData NullStringArray[1]
const EventType NetworkManagerServerEventTypeID
no params
@ AT_OPTIONS_VISIBILITY_SLIDER
Class for sending RPC over network.
Param2< PlayerIdentity, Man > ClientReconnectEventParams
PlayerIdentity, Man.
Param3< int, float, string > ProgressEventParams
state, progress, title
const EventType ServerFpsStatsUpdatedEventTypeID
params: ServerFpsStatsUpdatedEventParams
const EventType ClientRemovedEventTypeID
no params
Param2< bool, bool > VONStateEventParams
listening, toggled
const EventType ConnectingAbortEventTypeID
no params
Param4< int, string, string, string > ChatMessageEventParams
channel, from, text, color config class
proto void Print(void var)
Prints content of variable to console/log.
const EventType LogoutEventTypeID
params: LogoutEventParams
const EventType ClientConnectedEventTypeID
params: ClientConnectedEventParams
Param1< int > MPConnectionLostEventParams
Duration.
@ AT_OPTIONS_FIELD_OF_VIEW
const EventType MPSessionPlayerReadyEventTypeID
no params
proto native float GetMax()
const EventType DialogQueuedEventTypeID
no params
const EventType ClientNewEventTypeID
params: ClientNewEventParams
const EventType StartupEventTypeID
no params
const EventType VONStateEventTypeID
params: VONStateEventParams
Param1< string > ScriptLogEventParams
@ AT_CONFIG_MOUSE_FILTERING
@ AT_OPTIONS_VON_INPUT_MODE
Param1< int > RespawnEventParams
RespawnTime.
Param5< PlayerIdentity, bool, vector, float, int > ClientPrepareEventParams
PlayerIdentity, useDB, pos, yaw, preloadTimeout (= additional time in seconds to how long server wait...
Param2< PlayerIdentity, Man > ClientReadyEventParams
PlayerIdentity, Man.
proto native float GetDefault()
const EventType PlayerDeathEventTypeID
params: PlayerDeathEventParams
const EventType LoginStatusEventTypeID
params: LoginStatusEventParams
@ AT_OPTIONS_MOUSE_YAXIS_INVERTED
Param2< string, string > LoginStatusEventParams
text message for line 1, text message for line 2
const int PROGRESS_PROGRESS
@ AT_OPTIONS_CONTROLLER_LS_XAXIS
Param1< string > DLCOwnerShipFailedParams
world name
@ AT_OPTIONS_CONTROLLER_LS_XAXIS_VEHICLE_MOD
Serializer ParamsWriteContext
const EventType ScriptLogEventTypeID
params: ScriptLogEventParams
const EventType ChatMessageEventTypeID
params: ChatMessageEventParams
Serialization general interface. Serializer API works with:
const EventType ClientReconnectEventTypeID
params: ClientReconnectEventParams
Param1< float > ServerFpsStatsUpdatedEventParams
float
The class that will be instanced (moddable)
Param1< vector > PreloadEventParams
Position.
const EventType SelectedUserChangedEventTypeID
no params
@ AT_OPTIONS_EFFECTS_SLIDER
Serializer ParamsReadContext
@ AT_OPTIONS_CONTROLLER_RS_YAXIS
Player description (base engine class)
@ OPTION_CONNECTIVITY_INFO
@ AT_OPTIONS_MOUSE_AND_KEYBOARD
@ AT_OPTIONS_CONTROLLER_RS_XAXIS_AIM_MOD
Param1< PlayerIdentity > ConnectivityStatsUpdatedEventParams
PlayerIdentity.
proto native void WriteValue(float value)
@ AT_OPTIONS_CONTROLLER_LS_YAXIS
proto native float GetMin()
Param1< Man > LogoutCancelEventParams
Player.
@ AT_OPTIONS_CONTROLLER_RS_XAXIS
const EventType PreloadEventTypeID
params: PreloadEventParams
const EventType MPConnectionCloseEventTypeID
params: MPConnectionCloseEventParams
const EventType SetFreeCameraEventTypeID
params: SetFreeCameraEventParams
Param2< DayZPlayer, Object > PlayerDeathEventParams
Player, "Killer" (Beware: Not necessarily actually the killer, Client doesn't have this info)
const EventType VONStopSpeakingEventTypeID
params: VONStopSpeakingEventParams
const EventType ClientDisconnectedEventTypeID
params: ClientDisconnectedEventParams
const EventType ConnectingStartEventTypeID
no params
OptionAccessType
C++ OptionAccessType.
@ AT_OPTIONS_VON_THRESHOLD_SLIDER
Param2< PlayerIdentity, Man > ClientNewReadyEventParams
PlayerIdentity, Man.
Keeps information about currently loaded world, like temperature.
@ OPTION_BLEEDINGINDICATION
Param1< FreeDebugCamera > SetFreeCameraEventParams
Camera.
Result for an object found in CGame.IsBoxCollidingGeometryProxy.
Param1< int > LoginTimeEventParams
LoginTime.
Param2< string, string > ClientConnectedEventParams
Name, uid.
Param1< int > ChatChannelEventParams
Param2< string, string > VONStopSpeakingEventParams
player name, player id
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
Param3< int, int, bool > WindowsResizeEventParams
Width, Height, Windowed.
@ AT_OPTIONS_TERRAIN_SHADER
@ AT_OPTIONS_MUSIC_SLIDER
Module containing compiled scripts.
const EventType VONStartSpeakingEventTypeID
params: VONStartSpeakingEventParams
InventoryTraversalType
tree traversal type, for more see http://en.wikipedia.org/wiki/Tree_traversal
const EventType ConnectivityStatsUpdatedEventTypeID
params: ConnectivityStatsUpdatedEventParams
const EventType MPSessionFailEventTypeID
no params
@ AT_OPTIONS_OBJECT_VISIBILITY_SLIDER
@ AT_OPTIONS_CONTROLLER_RS_YAXIS_AIM_MOD
Param1< int > LogoutEventParams
logoutTime
const int PROGRESS_UPDATE
@ AT_OPTIONS_BRIGHT_SLIDER
const EventType LogoutCancelEventTypeID
params: LogoutCancelEventParams
const EventType ChatChannelEventTypeID
params: ChatChannelEventParams
const EventType LoginTimeEventTypeID
params: LoginTimeEventParams
@ AT_OPTIONS_CONTROLLER_RS_YAXIS_INVERTED
const EventType WorldCleaupEventTypeID
no params
const int PROGRESS_FINISH
private proto Managed GetScriptEvents()
Get the events.
const EventType ClientRespawnEventTypeID
params: ClientRespawnEventParams
const EventType MPConnectionLostEventTypeID
params: MPConnectionLostEventParams
Param3< PlayerIdentity, vector, Serializer > ClientNewEventParams
PlayerIdentity, PlayerPos, Top, Bottom, Shoe, Skin.
const EventType MPSessionStartEventTypeID
no params
@ AT_OPTIONS_MASTER_VOLUME
class ListOptionsAccess extends OptionsAccess ReadValue
class MenuDefaultCharacterData m_AttachmentSlots
@ AT_OPTIONS_DISPLAY_MODE
@ AT_OPTIONS_MOUSE_YAXIS_AIM_MOD
const EventType ProgressEventTypeID
params: ProgressEventParams
const EventType MPSessionEndEventTypeID
no params
script counterpart to engine's class Inventory
EntityAI SpawnEntity(string object_name, notnull InventoryLocation inv_loc, int iSetupFlags, int iRotation)
const EventType ClientPrepareEventTypeID
params: ClientPrepareEventParams
Param2< PlayerIdentity, Man > ClientRespawnEventParams
PlayerIdentity, Man.
ScriptInvoker Class provide list of callbacks usage: