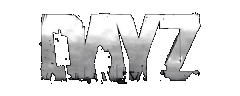 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
81 if ( !
GetGame().IsDedicatedServer() )
90 int id = element.GetType();
116 mask_array.Insert(mask);
120 mask = mask | (
GetElement(i).GetValue() << offset);
121 offset = offset +
GetElement(i).GetNumberOfBits();
124 mask_array.Insert(mask);
129 int maskArrayIndex = 0;
143 mask = mask_array.Get(maskArrayIndex);
145 offset = offset +
GetElement(i).GetNumberOfBits();
153 int value = mask & (compareMask << index);
154 value = value >> index;
174 rpc.Write(mask_array);
182 if ( array_a.Count() != array_b.Count() )
return false;
183 for (
int i = 0; i <array_a.Count(); i++)
185 if ( array_a.Get(i) != array_b.Get(i) )
198 if ( element && element.IsClientOnly() && element.IsValueChanged() )
209 if ( element && !element.IsClientOnly() && element.IsValueChanged() )
220 ctx.Read(mask_array);
proto native CGame GetGame()
VIRTUAL_HUD_UPDATE_INTERVAL
how often virtual hud checks if there is a difference since last sync
void VirtualHud(PlayerBase player)
class LogTemplates Log(string message, LogTemplateID template_id=0)
Creates debug log (optional) from LogTemplate which are registred.
ref array< int > m_LastSentArray
enum DSLevels WARNING_PLUS
DisplayElementBase GetElement(eDisplayElements element_id)
PluginBase GetPlugin(typename plugin_type)
Serialization general interface. Serializer API works with:
void OnRPC(ParamsReadContext ctx)
int BitToDec(int mask, int index, int compareMask)
enum DSLevels NUMBER_OF_MASKS
enum DSLevels WARNING_MINUS
void RegisterElement(DisplayElementBase element)
enum DSLevels BLINKING_PLUS
enum DSLevels CRITICAL_PLUS
void PrintString(string s)
Helper for printing out string expression. Example: PrintString("Hello " + var);.
Result for an object found in CGame.IsBoxCollidingGeometryProxy.
enum DSLevels CRITICAL_MINUS
void DeserializeElements(ref array< int > mask_array)
PluginPlayerStatus m_ModulePlayerStatus
enum DSLevels BLINKING_MINUS
ref array< ref Param > rpcParams
const int NUMBER_OF_ELEMENTS
void SerializeElements(ref array< int > mask_array)
bool AreArraysSame(notnull array< int > array_a, notnull array< int > array_b)
ref DisplayElementBase m_Elements[NUMBER_OF_ELEMENTS]