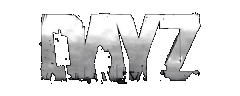 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
6 static void CreateManagerStatic()
10 Debug.Log(
"PPEManagerStatic | CreateManagerStatic - PPEManager already exists");
17 static void DestroyManagerStatic()
67 PPERequesterBank.Init();
72 PPERequesterBank.Cleanup();
96 m_ManagerInitialized =
true;
139 m_PPEClassMap.Set(material_class.GetPostProcessEffectID(), material_class);
146 mat_class.InsertParamValueData(data);
153 if ( order > PPEConstants.DEPENDENCY_ORDER_HIGHEST )
172 mat_class.SetParameterUpdating(order,parameter_id);
196 if ( active && found == -1 )
200 else if ( !active && found > -1 )
213 Debug.Log(
"PPEManager | SetRequestUpdating | !m_UpdatingRequests");
219 if ( active && idx == -1 )
223 else if ( !active && idx > -1 )
243 foreach (
typename requesterType : requesters)
247 if (ppeRequester && ppeRequester.IsRequesterRunning())
260 int count = req.GetActiveRequestStructure().Count();
262 for (
int i = 0; i < count; i++)
264 mat_id = req.GetActiveRequestStructure().GetKey(i);
266 mat_class.RemoveRequest(req.GetRequesterIDX());
293 req.OnUpdate(timeslice);
307 mat_class.OnUpdate(timeslice,i);
319 mat_class.ApplyValueChanges();
341 return mat_class.GetParameterCommandData(parameter).GetDefaultValues();
348 return mat_class.GetParameterCommandData(parameter).GetCurrentValues();
359 typename name = mat_class.Type();
361 postprocess_capsule.ChangeMaterialPathUsed(
path);
363 if (postprocess_capsule.GetMaterial() == 0x0)
365 Debug.Log(
"PPEManager | Invalid material path " +
path +
" used for " +
name );
374 if (!scriptside_only)
385 if (requester.GetCategoryMask() & mask)
PostProcessEffectType
Post-process effect type.
void SetRequestActive(PPERequesterBase request, bool active)
Marks requester as 'active'. Currently indistinguiishable from 'updating' requester,...
proto native CGame GetGame()
Created once, on manager init. Script-side representation of C++ material class, separate handling.
void InsertUpdatedMaterial(int mat_id)
Marks material class as updated and values to be set in the course of update - 'ProcessApplyValueChan...
EV postprocess, does not directly use materials.
protected ref array< int > m_UpdatedMaterials
FXAA - PostProcessEffectType.FXAA.
Base Param Class with no parameters. Used as general purpose parameter overloaded with Param1 to Para...
Dummy class - PostProcessEffectType.None.
protected void InitPPEManagerClassMap()
Ordered by 'PostProcessEffectType' for easy access through the same enum; ID saved all the same.
protected void ProcessApplyValueChanges()
ModifiersManager m_Manager
protected ref array< ref PPERequesterBase > m_UpdatingRequests
SSAO - PostProcessEffectType.SSAO.
protected void ProcessRequesterUpdates(float timeslice)
GodRays - PostProcessEffectType.GodRays.
void SetRequestUpdating(PPERequesterBase request, bool active)
Marks requester as 'updating' and to be processed on manager update.
void SetMaterialParamUpdating(int material_id, int parameter_id, int order)
Queues material/parameter to update (once)
class PPEManagerStatic CAMERA_ID
protected void RegisterPPEClass(PPEClassBase material_class)
Registeres material class and creates data structure within.
GaussFilter - PostProcessEffectType.GaussFilter.
void Init()
Launched from 'DayZGame.DeferredInit' to make earlier access, use, and updates impossible (downside o...
protected ref array< ref PPERequesterBase > m_ExistingPostprocessRequests
Param GetPostProcessDefaultValues(int material, int parameter)
Returns default values as Param. See 'PPEConstants' file for various typedefs used.
protected bool m_ManagerInitialized
protected void RemoveActiveRequestFromMaterials(PPERequesterBase req)
Static component of PPE manager, used to hold the instance.
Param GetPostProcessCurrentValues(int material, int parameter)
Returns current values as Param. See 'PPEConstants' file for various typedefs used.
void SendMaterialValueData(PPERequestParamDataBase data)
g_Game.NightVissionLightParams, does not directly use materials. Controls light multiplication and fi...
Rain - PostProcessEffectType.Rain.
SunMask - PostProcessEffectType.SunMask.
HBAO - PostProcessEffectType.HBAO.
Glow - PostProcessEffectType.Glow.
UnderWater - PostProcessEffectType.UnderWater.
protected void ClearMaterialUpdating()
void RemoveMaterialUpdating(int material_id, int order=0)
Currently unused, requests remain in the hierarchy and are used when needed (slightly faster than con...
Data for one material parameter, requester side.
WetDistort - PostProcessEffectType.WetDistort.
proto native void SetCameraPostProcessEffect(int cam, int priority, PostProcessEffectType type, string materialPath)
ChromAber - PostProcessEffectType.ChromAber.
Result for an object found in CGame.IsBoxCollidingGeometryProxy.
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
protected ref map< int, ref PPEClassBase > m_PPEClassMap
bool IsAnyRequesterRunning(array< typename > requesters)
DynamicBlur - PostProcessEffectType.DynamicBlur.
PostProcessPrioritiesCamera
PPE type priorities, C++ based. DO NOT CHANGE ORDER! Used only when calling 'SetCameraPostProcessEffe...
bool GetExistingRequester(typename req, out PPERequesterBase ret)
FilmGrain - PostProcessEffectType.FilmGrain.
DepthOfField - PostProcessEffectType.DepthOfField.
Colors - PostProcessEffectType.Colors.
Eye Accomodation postprocess, does not directly use materials.
SMAA - PostProcessEffectType.SMAA.
void StopAllEffects(int mask=0)
stops all effects of a certain kind
protected ref map< int, ref array< int > > m_PPEMaterialUpdateQueueMap
protected void RequestsCleanup()
Unused cleanup method, should it be ever needed.
DOF postprocess, does not directly use materials.
void DbgPrnt(string text)
void Update(float timeslice)
ColorGrading - PostProcessEffectType.ColorGrading.
RadialBlur - PostProcessEffectType.RadialBlur.
protected void ProcessMaterialUpdates(float timeslice)
void ChangePPEMaterial(PostProcessPrioritiesCamera priority, PostProcessEffectType type, string path, bool scriptside_only)
Changes material file associated with the script material class. Will be used very rarely,...