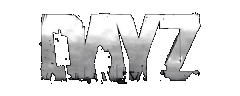 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
68 static const int VAR_TYPE_BOOL = 1;
69 static const int VAR_TYPE_INT = 2;
70 static const int VAR_TYPE_FLOAT = 4;
71 static const int VAR_TYPE_COLOR = 8;
72 static const int VAR_TYPE_VECTOR = 16;
74 static const int VAR_TYPE_BLENDABLE = VAR_TYPE_INT|VAR_TYPE_FLOAT|VAR_TYPE_COLOR;
75 static const int VAR_TYPE_TEXTURE = 32;
76 static const int VAR_TYPE_RESOURCE = 64;
80 static const int DEPENDENCY_ORDER_BASE = 0;
81 static const int DEPENDENCY_ORDER_HIGHEST = 1;
@ SUBSTRACT_REVERSE_RELATIVE
Param2< string, ref array< float > > PPETemplateDefVector
Param2< string, string > PPETemplateDefResource
Param5< string, float, float, float, float > PPETemplateDefColor
Param2 PPETemplateDefBool
Param4< string, int, int, int > PPETemplateDefInt
Param2< string, string > PPETemplateDefTexture
PostProcessPrioritiesCamera
PPE type priorities, C++ based. DO NOT CHANGE ORDER! Used only when calling 'SetCameraPostProcessEffe...
PPOperators
PP operators, specify operation between subsequent layers.
Param4< string, float, float, float > PPETemplateDefFloat