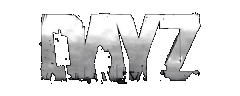 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
8 WaterLevel = pWaterLevel;
28 float RemainingDuration;
95 protected static const string WATER_LEVEL_MAX =
"water_level_max";
96 protected static const string WATER_LEVEL_AVERAGE =
"water_level_average";
97 protected static const string WATER_LEVEL_ABOVE_PIPES =
"water_level_above_pipes";
98 protected static const string WATER_LEVEL_MIN =
"water_level_min";
104 WATER_LEVEL_ABOVE_PIPES,
135 protected bool m_PipeUnderwaterSoundRunning
144 "pipe_creaking_sound_pos_1",
145 "pipe_creaking_sound_pos_2",
146 "pipe_creaking_sound_pos_3",
147 "pipe_creaking_sound_pos_4"
179 float waterHeight, pressureLevel;
208 if (wlStageSettings.Duration == -1.0)
218 float adjustedDuration = wlStageSettings.Duration;
227 float start = adjustedWaterHeight;
249 int allValvesStates = 0;
261 if (plStageSettings.Duration == -1.0)
301 if (allValvesStates ==
false)
310 super.OnVariablesSynchronized();
380 RegisterNetSyncVariableInt(
"m_ValveStatesPacked", 0);
381 RegisterNetSyncVariableInt(
"m_ValveManipulatedIndex", -1,
VALVES_COUNT - 1);
382 RegisterNetSyncVariableInt(
"m_WaterLevelActual",
WL_MIN,
WL_MAX);
383 RegisterNetSyncVariableInt(
"m_WaterLevelPrev",
WL_MIN,
WL_MAX);
406 GetGame().RegisterNetworkStaticObject(
this);
470 string targetedValveName = GetActionComponentName(pComponentIndex);
549 valvePositions.Insert(posPoint);
550 valvePositions.Insert(dirPoint);
552 return valvePositions;
642 switch (pPressureLevel)
686 return wlStageSettings;
703 return wlStageSettings;
740 if (pDeanimationRequest)
743 return plStageSettings;
756 if (pDeanimationRequest)
759 return plStageSettings;
773 return plStageSettings;
783 if (pDeanimationRequest)
786 return plStageSettings;
792 if (pDeanimationRequest)
795 return plStageSettings;
802 return plStageSettings;
903 #ifdef DIAG_DEVELOPER
905 if (FeatureTimeAccel.GetFeatureTimeAccelEnabled(ETimeAccelCategories.UNDERGROUND_RESERVOIR))
907 timeAccel = FeatureTimeAccel.GetFeatureTimeAccelValue();
908 return originalTime * timeAccel;
918 if (MemoryPointExists(pMemoryPoint))
920 pos = GetMemoryPointPos(pMemoryPoint);
921 pos = ModelToWorld(pos);
925 ErrorEx(
string.Format(
"Memory point %1 not found, falling back to vector.Zero", pMemoryPoint));
982 if (drainPressureLevel >= 0.4 && drainPressureLevel < 0.8)
995 if (drainPressureLevel < 0.4)
1007 if (fillPressureLevel >= 0.4)
1019 if (fillPressureLevel < 0.4)
1049 if (m_PipeUnderwaterSoundRunning)
1052 m_PipeUnderwaterSoundRunning =
false;
1238 for (
int i = 0; i < pStates.Count(); ++i)
1240 if (pStates[i] ==
true)
1242 packedBits |= 1 << i;
1252 for (
int i = 0; i < pArrayLength; ++i)
1254 if ((pPackedBits & 1 << i) != 0)
1256 unpackedBools.Insert(
true);
1260 unpackedBools.Insert(
false);
1264 return unpackedBools;
1270 string debug_output =
"";
1281 return debug_output;
1284 override void GetDebugActions(out TSelectableActionInfoArrayEx outputList)
1291 super.GetDebugActions(outputList);
1296 if (super.OnAction(action_id, player, ctx))
1300 if (action_id ==
EActions.SPECIALIZED_ACTION1)
1308 else if (action_id ==
EActions.SPECIALIZED_ACTION2)
1317 else if (action_id ==
EActions.SPECIALIZED_ACTION3)
protected ref array< bool > m_PressureAnimationRequests
proto native CGame GetGame()
const protected int WATER_LEVELS_COUNT
const protected string SOUND_NAME_PIPE_CREAKING
protected bool IsValvePressureLevelGaugeAtBase(int pValveIndex)
protected ref WaterLevelSnapshot m_WaterLevelSnapshot
for deanimation purposes
protected ref ParticleSourceArray m_ValveParticles
const protected string PIPE_NAME_LEAKING_FILL
const protected int PARTICLE_FILL_PIPE_JET
protected ref array< ref PressureLevelSettings > m_DrainValvePressureStageSettings
ActionUncoverHeadSelfCB ActionTurnValveUndergroundReservoir
const protected string ANIM_PHASE_VALVE_DRAIN
const int INDEX_NOT_FOUND
protected void ResetState()
protected float m_WaterLevelHeightActual
protected int m_ValveStatesPackedPrev
protected void CleanSoundEffects()
protected int HeightToWaterLevel(float pHeight)
protected ref EffectSound m_ValveManipulationSound
const protected string SOUND_NAME_VALVE_MANIPULATION
const protected string PIPE_NAME_LEAKING_DRAIN
const protected int PIPE_CREAKING_MAX_TIME_DELAY_MS
override int GetTurnableValveIndex(int pComponentIndex)
protected int m_FillValveWaterLevelStageIndex
protected ref array< ref WaterLevelSettings > m_DrainValveWaterStageSettings
valve/pipe stages for water and pressure levels
protected int m_DrainValvePressureLevelStageIndex
protected int m_ValveManipulatedIndex
protected ref array< ref PressureLevelSettings > m_FillValvePressureStageSettings
protected ref array< bool > m_PressureDeanimationRequests
const protected string ANIM_PHASE_VALVE_GAUGE_FILL
override void SetActions()
protected ref array< ref PressureLevelSettings > m_FillValvePressureDeanimationSettings
const protected string SOUND_NAME_PIPE_SPRINKLING_END
const protected int WL_AVERAGE
protected PressureLevelSettings PreviousPressureLevelStageSettings(int pValveIndex, bool pDeanimationRequest=false)
const protected string SOUND_NAME_WATER_FILL_LOOP
protected array< bool > UnpackBitsToArrayOfBoolStates(int pPackedBits, int pArrayLength)
protected ref EffectSound m_PipeCreakingSounds
protected ref map< string, vector > m_WaterLevelsAvailable
Input value between 0 and 1, returns value adjusted by easing, no automatic clamping of input(do your...
protected void ConfigureValvesAndGaugesCourse()
protected float WaterLevelToHeight(int pWaterLevel)
protected void PlayValveManipulationSound()
protected void SetDefaultPressureLevelStageSettings(int pValveIndex)
protected PressureLevelSettings ActualPressureLevelStageSettings(int pValveIndex, bool pDeanimationRequest=false)
protected Object m_SpawnedWaterObject
const protected int PIPE_INDEX_BROKEN2
main broken pipe
protected void HandleVisualEffects()
protected bool IsAnyValveActive()
const protected string SOUND_NAME_PIPE_SPRINKLING_START
Serialization general interface. Serializer API works with:
array< ParticleSource > ParticleSourceArray
protected int m_FillValvePressureLevelStageIndex
protected float GetValvePressureLevelGauge(int pValveIndex)
protected void HandleSoundEffectsUnderwaterPipeSounds()
protected void SetWaterLevelHeight(float pHeight)
protected ref array< EffectSound > m_PipeSounds
protected vector m_WaterLevelDefault
protected void PlayPipeCreakingSoundOnLocation()
protected void LateInit()
Wrapper class for managing sound through SEffectManager.
protected WaterLevelSettings ActualWaterLevelStageSettings(int pValveIndex)
protected bool m_ValveManipulationSoundRequested
VFX/SFX.
protected ref array< ref PressureLevelSettings > m_DrainValvePressureDeanimationSettings
protected ref array< bool > m_ValveStates
protected bool m_PipeUnderwaterSoundRunning protected ref EffectSound m_PipeUnderwaterSound
const protected string SOUND_NAME_UPIPE_SPRINKLING_LOOP
protected void SetValvePressureLevelGauge(int pValveIndex, float pValue)
override bool HasTurnableValveBehavior()
const protected int VALVES_COUNT
protected int m_ValveManipulatedIndexPrev
protected ref array< float > m_WaterLevelTimesAccumulated
const protected int VALVE_INDEX_FILL
protected float AdjustTime(float originalTime)
const protected int PARTICLE_FILL_PIPE_MAX_PRESSURE
const protected int PIPE_INDEX_BROKEN1
class WaterLevelSnapshot OBJECT_NAME_WATER_PLANE
class WaterLevelSettings PressureLevel
protected void SyncValveVariables()
protected int PackArrayOfBoolStatesIntoBits(array< bool > pStates)
protected int m_ValveStatesPacked
void AddAction(typename actionName)
protected WaterLevelSettings PreviousWaterLevelStageSettings(int pValveIndex)
const protected int PIPES_BROKEN_COUNT
const protected string PIPE_CREAKING_SOUND_LOCATIONS[PIPE_CREAKING_SOUND_LOCATIONS_COUNT]
protected void HandleSoundEffectsPipeCreaking()
override void GetDebugActions(out TSelectableActionInfoArrayEx outputList)
const protected int PARTICLE_FILL_PIPE_JET_WEAK
protected ref array< string > m_ValveNames
protected vector GetMemoryPointPosition(string pMemoryPoint)
protected ref array< ref WaterLevelSettings > m_FillValveWaterStageSettings
for deanimation purposes
void OnValveManipulationCanceled(int pValveIndex)
const protected string VALVE_NAME_FILL
const protected string VALVE_NAME_DRAIN
protected float PressureLevelToValue(int pPressureLevel)
array< vector > GetValveAligningPointsWS(int pValveIndex)
void OnValveManipulationEnd(int pValveIndex)
protected int m_DrainValveWaterLevelStageIndex
pointing to specific stage for each valve/pipe
const protected int PIPE_CREAKING_MIN_TIME_DELAY_MS
void OnValveManipulationStart(int pValveIndex)
const protected int VALVE_INDEX_DRAIN
override bool OnAction(int action_id, Man player, ParamsReadContext ctx)
const protected string PIPE_NAME_BROKEN1
const protected string WATER_LEVELS[WATER_LEVELS_COUNT]
Result for an object found in CGame.IsBoxCollidingGeometryProxy.
void ~Land_Underground_WaterReservoir()
protected void HandleSoundEffects()
void PressureLevelSettings(int pPressureLevel, float pDuration)
const protected string SOUND_NAME_UPIPE_SPRINKLING_END
protected void CleanVisualEffects()
override void EOnPostSimulate(IEntity other, float timeSlice)
const protected string SOUND_NAME_PIPE_SPRINKLING_LOOP2
protected void AnimateValve(int pValveIndex, float pPhase)
protected ref ParticleSourceArray m_PipeBrokenParticles
protected void SetLastActiveValve(int pValveIndex)
const protected int PL_MAX
void Land_Underground_WaterReservoir()
protected void HandleSoundEffectsPipeSprinkling()
const protected string SOUND_NAME_PIPE_SPRINKLING_LOOP1
const protected string SOUND_NAME_WATER_DRAIN_LOOP
const protected int WL_MIN
protected int m_LastActiveValve
override void OnVariablesSynchronized()
protected ref array< float > m_PressureTimesAccumulated
protected ref EffectSound m_WaterLevelMovementSound
const protected int PIPE_CREAKING_SOUND_LOCATIONS_COUNT
const protected int PL_AVERAGE
const int ECE_CREATEPHYSICS
const protected int PARTICLE_DRAIN_PIPE_MAX_PRESSURE
protected void TranslateMemoryPointsToWaterLevels()
protected bool IsValveActive(int pValveIndex)
const int SAT_DEBUG_ACTION
protected void AdvanceToNextPressureLevelStageSettings(int pValveIndex)
protected void AdvanceToNextWaterLevelStageSettings(int pValveIndex)
const protected int PL_MIN
const protected string PIPE_NAME_BROKEN2
protected int m_WaterLevelActual
override bool IsValveTurnable(int pValveIndex)
const protected string ANIM_PHASE_VALVE_FILL
void ParticleManager(ParticleManagerSettings settings)
Constructor (ctor)
EntityEvent
Entity events for event-mask, or throwing event from code.
Param4< int, int, string, int > TSelectableActionInfoWithColor
protected int m_WaterLevelPrev
Manager class for managing Effect (EffectParticle, EffectSound)
protected bool m_PipeCreakingSoundRequested
proto native vector Vector(float x, float y, float z)
Vector constructor from components.
protected void HandleSoundEffectsWaterLevelMovementSounds()
const protected int WL_ABOVE_PIPES
protected void RegisterValve(string pCompName, int pIndex)
const protected int WL_MAX
protected void Init()
Launched from 'DayZGame.DeferredInit' to make earlier access, use, and updates impossible (downside o...
const protected string SOUND_NAME_UPIPE_SPRINKLING_START
const protected string ANIM_PHASE_VALVE_GAUGE_DRAIN
tighter broken pipe