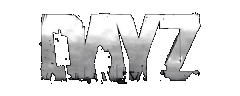 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
74 const string Economy =
"economy";
75 const string EconomyRespawn =
"economy_respawn";
76 const string RespawnQueue =
"respawn_queue";
78 const string Matrix =
"matrix";
79 const string UniqueLoot =
"uniqueloot";
80 const string Bind =
"bind";
81 const string SetupFail =
"setupfail";
82 const string Storage =
"storage";
83 const string Classes =
"class";
84 const string Category =
"category";
85 const string Tag =
"tag";
86 const string SCategory =
"s_category";
87 const string STag =
"s_tag";
88 const string SAreaflags =
"s_areaflags";
89 const string SCrafted =
"s_crafted";
90 const string MapGroup =
"map_group";
91 const string MapComplete =
"map_complete";
92 const string InfectedZone =
"infected_zone";
112 static string Category(
string category)
114 return string.Format(
"category:%1", category);
127 static string Tag(
string tag)
129 return string.Format(
"tag:%1", tag);
167 const string LINKS =
"links";
172 const string SUSPICIOUS =
"suspicious";
177 const string DE_CLOSE_POINT =
"declosepoint";
182 const string ABANDONED =
"abandoned";
187 const string EMPTY =
"empty";
193 const string CLOSE =
"close";
198 const string WORLD =
"world";
203 const string STATUS =
"status";
208 const string LOOT_SIZE =
"lootsize";
276 proto native
void MarkCloseProxy(
float fRadius,
bool bAllSelections );
321 proto native
void TimeShift(
float fShift );
363 proto native
void SpawnDE(
string sEvName,
vector vPos,
float fAngle = -1 );
378 proto native
void SpawnDEEx(
string sEvName,
vector vPos,
float fAngle,
int uFlags );
393 proto native
void SpawnLoot(
string sEvName,
vector vPos,
float fAngle,
int iCount = 1,
float fRange = 1 );
409 proto native
void SpawnDynamic(
vector vPos,
bool bShowCylinders =
true,
float fDefaultDistance = 0 );
424 proto native
void SpawnVehicles(
vector vPos,
bool bShowCylinders =
false,
float fDefaultDistance = 20 );
440 proto native
void SpawnBuilding(
vector vPos,
bool bShowCylinders =
false,
float fDefaultDistance = 20 );
455 proto native
void SpawnEntity(
string sClassName,
vector vPos,
float fRange,
int iCount );
482 proto native
void SpawnRotation(
string sClassName,
vector vPos,
float fRange,
int iCount,
int iFlags );
494 proto native
void SpawnPerfTest(
string sClassName,
int iCount );
513 proto native
void EconomyLog(
string sLogType );
526 proto native
void EconomyMap(
string sMapType );
536 proto native
void EconomyOutput(
string sOutputType,
float fRange );
753 proto native
int GetNominal();
754 proto native
int GetMin();
756 proto native
float GetQuantityMin();
757 proto native
float GetQuantityMax();
759 proto native
float GetQuantity();
761 proto native
float GetLifetime();
762 proto native
float GetRestock();
764 proto native
int GetCost();
766 proto native
int GetUsageFlags();
767 proto native
int GetValueFlags();
proto native void AnimalSpawn()
"Animal Spawn"
proto native bool AvoidVehicle(vector vPos, float fDistance, string sDEName="")
Check if there is a vehicle within a radius.
proto native void LootSetDamageToOne()
"Set Damage = 1.0"
const string ALL_PROXY_ABANDONED
All abandoned loot spawns.
const string ALL_ANIMAL
All animals.
class CEItemProfile CEApi
API to interact with Central Economy.
proto native void SpawnBuilding(vector vPos, bool bShowCylinders=false, float fDefaultDistance=20)
Spawn all entities with building category through CE.
proto native void OverrideLifeTime(float fLifeTime)
Fills in the Debug Lifetime, which will be used for any new DE spawned.
proto native float GetCEGlobalFloat(string varName)
Get float from globals.xml.
proto native void ToggleDynamicEventStatus(bool mode)
"Dynamic Events Status"
const int ECE_EQUIP_ATTACHMENTS
proto native void LootDepleteAndDamage()
"Damage + Deplete"
proto native void ExportProxyData(vector vCenter=vector.Zero, float fRadius=0)
Generates "storage/export/mapgrouppos.xml".
const int ECE_NOPERSISTENCY_WORLD
proto native void EconomyOutput(string sOutputType, float fRange)
Outputs debug logs into server log or rpt.
proto native void LootToggleSpawnSetup(bool mode)
"Setup Vis"
proto native void SpawnVehicles(vector vPos, bool bShowCylinders=false, float fDefaultDistance=20)
Spawn all entities with vehicles category through CE.
proto native void SpawnEntity(string sClassName, vector vPos, float fRange, int iCount)
Spawn an entity through CE.
proto native void RadiusLifetimeDecrease(vector vCenter, float fRadius, float fValue)
Process lifetime decrease within radius by value (sec)
proto native void ToggleClusterVisualisation(bool mode)
"Cluster Vis"
const string ALL_INFECTED
All infected.
proto native void LootExportMap()
"<<< Export Map" / GetCEApi.ExportProxyData(vector.Zero, 0);
const string ALL_LOOT
All loot.
proto native void EconomyMap(string sMapType)
Outputs debug file to storage/lmap/*.tga showing the current places this is spawned.
proto native void SpawnDE(string sEvName, vector vPos, float fAngle=-1)
Force spawn specific dynamic event.
proto native void ListCloseProxy(float fRadius)
Outputs a list of all loot points closer than specified radius.
proto native void SpawnLoot(string sEvName, vector vPos, float fAngle, int iCount=1, float fRange=1)
Spawn an item through CE.
const int ECE_PLACE_ON_SURFACE
proto native void RadiusLifetimeIncrease(vector vCenter, float fRadius, float fValue)
Process lifetime increase within radius by value (sec)
proto native void RemoveCloseProxy()
Removes all invalid points.
const string ALL_PROXY_STATIC
All static loot spawns.
proto native void LootToggleProxyEditing(bool mode)
proto native void SpawnRotation(string sClassName, vector vPos, float fRange, int iCount, int iFlags)
Spawn an entity through CE.
Categories for CEApi.EconomyLog.
const int ECE_OBJECT_SWAP
proto native void ExportProxyProto()
Generates "storage/export/mapgroupproto.xml".
proto native void ExportClusterData()
Generates "storage/export/mapgroupcluster.xml".
proto native Object SpawnSingleEntity(string sClassName, vector vPos)
Spawn an entity through CE.
proto native void EconomyLog(string sLogType)
Outputs debug file to storage/log/*.csv.
proto native int GetCEGlobalInt(string varName)
Get int from globals.xml.
class EconomyOutputStrings ADAPTIVE
class EconomyOutputStrings VOLUME
proto native void ExportSpawnData()
Regenerates "storage/spawnpoints.bin" if necessary.
proto native Entity SpawnGroup(string sGroupName, vector vPos, float fAngle=-1)
Force spawn specific prototype group + loot at position.
const int RF_DECORRECTION
proto native void ToggleDynamicEventVisualisation(bool mode)
"Dynamic Events Vis"
class EconomyLogCategories EconomyMapStrings()
Special strings for CEApi.EconomyMap.
proto native void SpawnDynamic(vector vPos, bool bShowCylinders=true, float fDefaultDistance=0)
Spawn all entities with dynamic category through CE.
proto native void SpawnPerfTest(string sClassName, int iCount)
Spawn an entity through CE, x amount of times in a grid.
proto native void InfectedToggleZoneInfo(bool mode)
"Infected Zone Info"
proto native void LootRetraceGroupPoints()
"Re-Trace Group Points"
Special strings for CEApi.EconomyOutput.
proto native void LootDepleteLifetime()
"Deplete Lifetime"
const string ALL_PLAYER
All players.
const int ECE_NOSURFACEALIGN
proto native void CleanMap()
Queue up the depleting of lifetime of everything in the world.
proto native bool AvoidPlayer(vector vPos, float fDistance)
Check if there is a player within a radius.
const int ECE_ROTATIONFLAGS
proto native void LootToggleVolumeEditing(bool mode)
"Edit Volume"
class EconomyOutputStrings OCCUPIED
proto native void TimeShift(float fShift)
Subtracts the supplied value from the current lifetime of all items in the world.
proto native bool SpawnAnalyze(string sClassName)
Will emulate the spawning of the item which is being looked at and generate images (....
proto native void LootExportGroup()
"Export Group >>"
proto native void InfectedToggleVisualisation(bool mode)
"Infected Vis"
const string ALL_VEHICLE
All vehicles.
proto native void AnimalToggleVisualisation(bool mode)
"Animal Vis"
const string ALL_PROXY_DYNAMIC
All dynamic loot spawns.
proto native void RadiusLifetimeReset(vector vCenter, float fRadius)
Process lifetime reset to default value from DB within radius.
proto native void LootExportClusters()
"<<< Export Clusters" / GetCEApi().ExportClusterData()
proto native void MarkCloseProxy(float fRadius, bool bAllSelections)
Invalidates loot spawn points which are closer than the radius supplied.
proto native void PlatformStatTest()
proto native void InfectedResetCleanup()
"Reset Cleanup"
proto native void SpawnDEEx(string sEvName, vector vPos, float fAngle, int uFlags)
Force spawn specific dynamic event.
proto native void ToggleOverallStats(bool mode)
"Overall Stats"
proto native string GetCEGlobalString(string varName)
Get string from globals.xml.
private void ~EconomyMapStrings()
proto native void LootSetSpawnVolumeVisualisation(ESpawnVolumeVis mode)
"Spawn Volume Vis"
proto native void InfectedSpawn()
"Infected Spawn"
const int ECE_UPDATEPATHGRAPH
const int ECE_EQUIP_CONTAINER
const int ECE_DYNAMIC_PERSISTENCY
const int ECE_CREATEPHYSICS
proto native void ToggleLootVisualisation(bool mode)
"Loot Vis"
const int ECE_NOPERSISTENCY_CHAR
proto native void DynamicEventExport()
"Export Dyn Event >>"
proto native void ToggleVehicleAndWreckVisualisation(bool mode)
"Vehicle&Wreck Vis"
proto native void AnimalAmbientSpawn()
"Ambient Spawn"
const string ALL_PROXY
All proxies.
class EconomyOutputStrings OFF
const int ECE_EQUIP_CARGO
proto native int CountPlayersWithinRange(vector vPos, float fRange)
Check if there is a vehicle within a radius.
proto native void DynamicEventSpawn()
"Dynamic Events Spawn"
proto native CEApi GetCEApi()
Get the CE API.
const int ECE_IN_INVENTORY
proto native void LootExportAllGroups()
"Export All Groups >>>>" / GetCEApi.ExportProxyProto();
const string ALL_ALL
Everything.