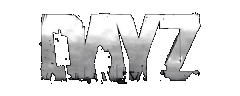 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
4 const string ANIMATION_OPENED =
"LidOff";
5 const string ANIMATION_CLOSED =
"LidOn";
7 protected bool m_IsOpenedClient =
false;
25 RegisterNetSyncVariableBool(
"m_Openable.m_IsOpened");
48 m_AreaDamage.SetExtents(
"-0.15 0 -0.15",
"0.15 0.75 0.15");
51 m_AreaDamage.SetHitZones({
"Head",
"Torso",
"LeftHand",
"LeftLeg",
"LeftFoot",
"RightHand",
"RightLeg",
"RightFoot"});
63 return "disableContainerDamage";
68 super.OnWasAttached(parent, slot_id);
75 super.OnWasDetached(parent, slot_id);
80 override bool CanDetachAttachment(
EntityAI parent )
88 super.OnStoreSave( ctx );
90 ctx.Write( m_Openable.IsOpened() );
95 if ( !super.OnStoreLoad( ctx, version ) )
99 if ( version >= 110 && !ctx.Read( opened ) )
123 super.OnVariablesSynchronized();
135 SoundBarrelOpenPlay();
140 SoundBarrelClosePlay();
154 if ( GetHealthLevel() ==
GameConstants.STATE_RUINED || GetHierarchyRootPlayer() !=
null )
162 return super.CanReceiveAttachment(attachment, slotId);
169 return super.CanReceiveAttachment(attachment, slotId);
183 return super.CanLoadAttachment(attachment);
188 super.EEItemAttached(item, slot_name);
198 bool edible_base_attached =
false;
201 case "DirectCookingA":
203 edible_base_attached =
true;
205 case "DirectCookingB":
207 edible_base_attached =
true;
209 case "DirectCookingC":
211 edible_base_attached =
true;
216 edible_base_attached =
true;
220 edible_base_attached =
true;
224 edible_base_attached =
true;
228 edible_base_attached =
true;
233 if (
GetGame().IsServer() && edible_base_attached)
238 if (edBase.GetFoodStage())
240 edBase.SetCookingTime(0);
255 super.EEItemDetached(item, slot_name);
266 case "DirectCookingA":
269 case "DirectCookingB":
272 case "DirectCookingC":
295 cooking_pot.RemoveAudioVisualsOnClient();
300 FryingPan frying_pan = FryingPan.Cast(item);
301 frying_pan.RemoveAudioVisualsOnClient();
311 if ( !super.CanPutInCargo( parent ) )
334 return super.CanReceiveItemIntoCargo( item );
339 if (!super.CanLoadItemIntoCargo( item ))
345 if ( GetHierarchyParent() )
351 override bool CanReleaseCargo(
EntityAI cargo )
359 if (!super.CanPutIntoHands(parent))
369 if ( !GetInventory().IsAttachment() &&
IsOpen() )
381 if( !super.CanDisplayCargo() )
393 if( !super.CanDisplayAttachmentCategory( category_name ) )
399 if ( ( category_name ==
"CookingEquipment" ) || ( category_name ==
"Smoking" ) )
434 override void Close()
456 return m_Openable.IsOpened();
459 protected void UpdateVisualState()
463 SetAnimationPhase( ANIMATION_OPENED, 0 );
464 SetAnimationPhase( ANIMATION_CLOSED, 1 );
468 SetAnimationPhase( ANIMATION_OPENED, 1 );
469 SetAnimationPhase( ANIMATION_CLOSED, 0 );
506 override bool CanIgniteItem(
EntityAI ignite_target = NULL )
516 override bool IsIgnited()
521 override void OnIgnitedTarget(
EntityAI ignited_item )
536 void SoundBarrelOpenPlay()
539 sound.SetAutodestroy(
true );
542 void SoundBarrelClosePlay()
545 sound.SetAutodestroy(
true );
548 void DestroyClutterCutter(
Object clutter_cutter )
550 GetGame().ObjectDelete( clutter_cutter );
553 override bool IsThisIgnitionSuccessful(
EntityAI item_source = NULL )
576 return "placeBarrel_SoundSet";
593 super.OnDebugSpawn();
protected void RefreshFireParticlesAndSounds(bool force_refresh)
proto native CGame GetGame()
override void OnWasAttached(EntityAI parent, int slot_id)
override void OnIgnitedThis(EntityAI fire_source)
Executed on Server when some item ignited this one.
void ClearCookingEquipment(ItemBase pItem)
protected void AddToFireConsumables(ItemBase item)
override bool CanReceiveItemIntoCargo(EntityAI item)
protected ItemBase m_DirectCookingSlots[DIRECT_COOKING_SLOT_COUNT]
protected int PARTICLE_NORMAL_SMOKE
protected int PARTICLE_SMALL_SMOKE
bool DirectCookingSlotsInUse()
override void OnVariablesSynchronized()
const int ECE_PLACE_ON_SURFACE
protected bool m_RoofAbove
protected bool IsFuel(ItemBase item)
Returns if item attached to fireplace is fuel.
const string OBJECT_CLUTTER_CUTTER
override void SetTakeable(bool pState)
Serialization general interface. Serializer API works with:
class Hatchback_02_Blue extends Hatchback_02 OnDebugSpawn
class JsonUndergroundAreaTriggerData GetPosition
Wrapper class for managing sound through SEffectManager.
override void Open()
Implementations only.
override bool CanPutIntoHands(EntityAI parent)
override bool CanPutInCargo(EntityAI parent)
override bool HasFlammableMaterial()
override int GetDamageSystemVersionChange()
void CreateAreaDamage(string slot_name, float rotation_angle=0)
void AddAction(typename actionName)
protected int PARTICLE_STEAM_END
protected ItemBase m_SmokingSlots[SMOKING_SLOT_COUNT]
protected float m_LightDistance
Deferred version of AreaDamageLooped.
override bool CanRemoveFromCargo(EntityAI parent)
override void OnWasDetached(EntityAI parent, int slot_id)
protected FireConsumable GetFireConsumableByItem(ItemBase item)
protected int PARTICLE_FIRE_START
protected bool IsKindling(ItemBase item)
Returns if item attached to fireplace is kindling.
override bool IsPrepareToDelete()
void DestroyAreaDamage(string slot_name)
protected ref AreaDamageManager m_AreaDamage
void OnStoreSave(ParamsWriteContext ctx)
override bool IsBeingPlaced()
override string GetInvulnerabilityTypeString()
protected int PARTICLE_SMALL_FIRE
override bool CanDisplayCargo()
int GetNumberOfItems()
Returns the number of items in cargo, otherwise returns 0(non-cargo objects). Recursive.
protected bool m_Initialized
void StartFire(bool force_start=false)
override bool CanDisplayAttachmentCategory(string category_name)
override bool CanLoadAttachment(EntityAI attachment)
protected int PARTICLE_NORMAL_FIRE
protected int PARTICLE_FIRE_END
override void EEItemAttached(EntityAI item, string slot_name)
override bool CanLoadItemIntoCargo(EntityAI item)
Manager class for managing Effect (EffectParticle, EffectSound)
override bool CanReceiveAttachment(EntityAI attachment, int slotId)
override void EEItemDetached(EntityAI item, string slot_name)
override protected bool CanBeIgnitedBy(EntityAI igniter=NULL)
bool OnStoreLoad(ParamsReadContext ctx, int version)
protected void RemoveFromFireConsumables(FireConsumable fire_consumable)
override string GetPlaceSoundset()
bool IsSoundSynchRemote()
void RefreshFireplaceVisuals()