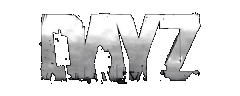 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
3 protected static float m_DefaultBrightness = 10;
4 protected static float m_DefaultRadius = 30;
8 SetVisibleDuringDaylight(
false);
11 SetFlareVisible(
false);
12 SetAmbientColor(1.0, 1.0, 0.3);
13 SetDiffuseColor(1.0, 1.0, 0.3);
15 SetDisableShadowsWithinRadius(-1);
50 RegisterNetSyncVariableBool(
"m_Armed");
51 RegisterNetSyncVariableBool(
"m_Defused");
62 super.OnExplosionEffects(source, directHit, componentIndex, surface, pos, surfNormal, energyFactor, explosionFactor, isWater, ammoType);
67 if (GetHierarchyParent())
69 parent = GetHierarchyParent();
82 super.EEDelete(parent);
95 super.EEKilled(killer);
107 if (!IsRuined() &&
GetArmed() && GetPairDevice())
126 GetPairDevice().UnpairRemote();
135 super.OnPlacementComplete(player, position, orientation);
139 SetOrientation(orientation);
163 for (
int i = 0; i < count; i++)
189 if (!super.IsInventoryVisible())
194 return GetAnimationPhase(
"Visibility") == 0;
199 return super.IsTakeable() && GetAnimationPhase(
"Visibility") == 0;
249 if (!super.CanPutInCargo(parent))
259 if (!super.CanPutIntoHands(parent))
304 if (GetHierarchyParent())
314 if (GetHierarchyParent())
322 super.OnStoreSave(ctx);
329 if (!super.OnStoreLoad(ctx, version))
335 if (!ctx.Read(armed))
protected ref array< string > m_AmmoTypes
proto native CGame GetGame()
protected vector m_ParticleOrientation
void SetParticleExplosion(int particle)
void OnDisarmed(bool pWithTool)
Legacy way of using particles in the game.
override void SetActions()
override void EEDelete(EntityAI parent)
override bool IsTakeable()
protected ExplosiveLight m_Light
light
override void EEKilled(Object killer)
override void Explode(int damageType, string ammoType="")
override bool OnStoreLoad(ParamsReadContext ctx, int version)
protected ref Timer m_DeleteTimer
override void OnExplosionEffects(Object source, Object directHit, int componentIndex, string surface, vector pos, vector surfNormal, float energyFactor, float explosionFactor, bool isWater, string ammoType)
protected float m_DefaultRadius
protected void InitiateExplosion()
override void OnPlacementComplete(Man player, vector position="0 0 0", vector orientation="0 0 0")
Serialization general interface. Serializer API works with:
override bool IsInventoryVisible()
protected ref array< ParticleSource > m_ParticleExplosionArr
class JsonUndergroundAreaTriggerData GetPosition
override void OnStoreSave(ParamsWriteContext ctx)
override bool CanPutIntoHands(EntityAI parent)
override bool CanPutInCargo(EntityAI parent)
ExplosiveLight DEFAULT_AMMO_TYPE
protected void DestroyParticle(Particle p)
void SetParticleOrientation(vector local_ori)
protected void CreateLight()
protected void SetDefused(bool state)
DamageType
exposed from C++ (do not change)
override bool CanRemoveFromHands(EntityAI parent)
void AddAction(typename actionName)
enum eAreaDecayStage m_DefaultBrightness
void LockExplosivesSlots()
override RemotelyActivatedItemBehaviour GetRemotelyActivatedItemBehaviour()
protected void OnExplode()
ActionAttachWheels ActionAttach
override void OnCEUpdate()
Entity which has the particle instance as an ObjectComponent.
override void UnpairRemote()
protected vector m_ParticlePosition
void Disarm(bool pWithTool=false)
void UpdateLED(int pState)
HELPERS.
proto native void SetPosition(vector position)
Set the world position of the Effect.
void SetAmmoType(string pAmmoType)
void SetParticlePosition(vector local_pos)
set position for smoke particle - needs to be in Local Space
bool HasLockedTriggerSlots()
protected Particle m_ParticleExplosion
particle
void UnlockTriggerSlots()
const protected string ANIM_PHASE_VISIBILITY
protected int m_ParticleExplosionId
void ParticleManager(ParticleManagerSettings settings)
Constructor (ctor)
void UnlockExplosivesSlots()
void SetAmmoTypes(array< string > pAmmoTypes)
protected void SetArmed(bool state)
override bool IsExplosive()