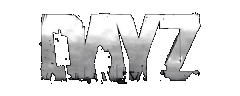 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
46 super.StartActivate(player);
48 if (!
GetGame().IsDedicatedServer())
63 SetHealth(
"",
"", 0.0);
69 if (!
GetGame().IsDedicatedServer())
91 if (victim && victim.IsInherited(
CarScript))
105 if (
GetGame().IsServer() && victim)
107 if (!victim.GetAllowDamage())
115 Param1<EntityAI> params =
new Param1<EntityAI>(victim);
124 if (victim_PB && victim_PB.IsAlive())
127 randNum =
Math.RandomInt(0, 100);
130 float damage = victim_PB.GetMaxHealth(
"RightLeg",
"");
131 victim_PB.DamageAllLegs( damage );
134 randNum =
Math.RandomInt(0, 100);
140 randNum =
Math.RandomIntInclusive(0,
PlayerBase.m_BleedingSourcesLow.Count() - 1);
142 victim_PB.m_BleedingManagerServer.AttemptAddBleedingSourceBySelection(
PlayerBase.m_BleedingSourcesLow[randNum]);
163 super.OnSteppedOn(victim);
181 obj.ProcessDirectDamage(DT_CLOSE_COMBAT,
this,
"",
"LandMineExplosion_CarWheel",
"0 0 0", 1);
201 super.OnItemLocationChanged(old_owner, new_owner);
206 super.EEKilled(killer);
213 if (!
GetGame().IsDedicatedServer())
216 sound.SetAutodestroy(
true);
220 override void Explode(
int damageType,
string ammoType =
"")
224 ammoType = ConfigGetString(
"ammoType");
229 ammoType =
"Dummy_Heavy";
236 DamageSystem.ExplosionDamage(
this, NULL, ammoType,
GetPosition() + offset, damageType);
248 super.OnRPC(sender, rpc_type, ctx);
250 Param1<bool> p =
new Param1<bool>(
false);
255 bool play = p.param1;
287 super.OnPlacementComplete(player, position, orientation);
303 return "landmine_deploy_SoundSet";
327 override void GetDebugActions(out TSelectableActionInfoArrayEx outputList)
333 super.GetDebugActions(outputList);
338 if (super.OnAction(action_id, player, ctx))
342 if (action_id ==
EActions.ACTIVATE_ENTITY)
346 else if (action_id ==
EActions.DEACTIVATE_ENTITY)
proto native CGame GetGame()
enum SoundTypeMine m_TimerLoopSound
protected ref array< int > m_ClothingDmg
PlaceObjectActionReciveData ActionReciveData ActionDeployObject()
void PlayDisarmingLoopSound()
bool m_AddDeactivationDefect
protected ref EffectSound m_SafetyPinSound
void StopDisarmingLoopSound()
const protected int DAMAGE_TRIGGER_MINE
override void OnActivatedByItem(notnull ItemBase item)
Called when this item is activated by other.
const private int MAX_BLEED_SOURCE
override void OnActivate()
HumanCommandScript fully scriptable command.
override void Explode(int damageType, string ammoType="")
protected void DamageClothing(PlayerBase player)
const private int BLEED_SOURCE_PROB
override void OnUpdate(EntityAI victim)
Serialization general interface. Serializer API works with:
override bool IsDeployable()
The class that will be instanced (moddable)
class Hatchback_02_Blue extends Hatchback_02 OnDebugSpawn
class JsonUndergroundAreaTriggerData GetPosition
Wrapper class for managing sound through SEffectManager.
const protected float UPDATE_TIMER_INTERVAL
protected EntityAI GetClosestCarWheel(EntityAI victim)
override void EEKilled(Object killer)
override void SetActions()
override void SetInactive(bool stop_timer=true)
DamageType
exposed from C++ (do not change)
override void OnPlacementComplete(Man player, vector position="0 0 0", vector orientation="0 0 0")
void AddAction(typename actionName)
override void OnSteppedOn(EntityAI victim)
override bool CanExplodeInFire()
override void GetDebugActions(out TSelectableActionInfoArrayEx outputList)
ActionAttachWheels ActionAttach
override bool OnAction(int action_id, Man player, ParamsReadContext ctx)
override void OnRPC(PlayerIdentity sender, int rpc_type, ParamsReadContext ctx)
Result for an object found in CGame.IsBoxCollidingGeometryProxy.
const private int BROKEN_LEG_PROB
override void OnSteppedOut(EntityAI victim)
override void StartActivate(PlayerBase player)
override void OnItemLocationChanged(EntityAI old_owner, EntityAI new_owner)
protected void GetPlayer()
protected ref EffectSound m_DisarmingLoopSound
protected void Synch(EntityAI victim)
keeping "step" here for consistency only
string m_InfoActivationTime
override bool CanBeDisarmed()
protected void OnServerSteppedOn(Object obj, string damageZone)
const int SAT_DEBUG_ACTION
Param4< int, int, string, int > TSelectableActionInfoWithColor
Manager class for managing Effect (EffectParticle, EffectSound)
proto native vector Vector(float x, float y, float z)
Vector constructor from components.
override string GetLoopDeploySoundset()
protected ref Timer m_DeleteTimer