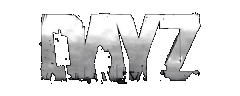 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
12 static const int FOLDED = 3;
13 static const int DEPLOYED = 2;
14 static const int TRIGGERED = 1;
34 RegisterNetSyncVariableInt(
"m_State");
39 super.OnVariablesSynchronized();
49 super.OnStoreSave(ctx);
57 if ( !super.OnStoreLoad(ctx, version) )
61 if ( !ctx.Read( state ) )
73 vector mins =
"-0.75 0.3 -0.01";
74 vector maxs =
"0.75 0.32 0.01";
89 if (!victim.GetAllowDamage())
97 victim.ProcessDirectDamage(DT_CLOSE_COMBAT,
this,
"",
"TripWireHit",
"0 0 0", 1);
105 sound.SetAutodestroy(
true);
111 super.OnItemLocationChanged(old_owner, new_owner);
123 super.EEItemLocationChanged(oldLoc, newLoc);
148 super.EEHealthLevelChanged(oldLevel, newLevel, zone);
162 super.SetInactive(stop_timer);
165 for (
int att = 0; att < GetInventory().AttachmentCount(); att++)
167 ItemBase attachment =
ItemBase.Cast(GetInventory().GetAttachmentFromIndex(att));
170 if (attachment.IsLockedInSlot())
172 attachment.UnlockFromParent();
175 attachment.OnActivatedByItem(
this);
176 GetInventory().DropEntity(
InventoryMode.SERVER,
this, attachment);
204 super.RefreshState();
214 super.SetupTrapPlayer( player, set_position );
220 super.StartDeactivate(player);
232 return super.CanReceiveAttachment( attachment, slotId );
241 return super.CanDisplayAttachmentSlot( slot_id );
246 super.EEItemAttached(item, slot_name);
253 super.EEItemDetached(item, slot_name);
265 super.EEKilled(killer);
289 #ifdef PLATFORM_WINDOWS
291 override int GetViewIndex()
293 if ( MemoryPointExists(
"invView2" ) )
296 GetInventory().GetCurrentInventoryLocation( il );
363 super.OnPlacementCancelled(player);
378 return GetState() != DEPLOYED || (GetInventory().AttachmentCount() == 0 &&
GetState() == DEPLOYED);
383 return "tripwire_deploy_SoundSet";
388 return "tripwiretrap_deploy_SoundSet";
405 if ( GetInventory().AttachmentCount() > 0)
407 ItemBase attachment =
ItemBase.Cast( GetInventory().GetAttachmentFromIndex(0) );
412 for (
int i = 1; i <= 3; i++)
414 HideSelection(
"s" + i +
"_charge");
418 string proxy_to_show =
string.Format(
"s%1_charge",
GetState() );
420 ShowSelection( proxy_to_show );
437 override void GetDebugActions(out TSelectableActionInfoArrayEx outputList)
443 super.GetDebugActions(outputList);
448 if (super.OnAction(action_id, player, ctx))
452 if (action_id ==
EActions.ACTIVATE_ENTITY)
456 else if (action_id ==
EActions.DEACTIVATE_ENTITY)
override void OnSteppedOn(EntityAI victim)
proto native CGame GetGame()
override void EEHealthLevelChanged(int oldLevel, int newLevel, string zone)
PlaceObjectActionReciveData ActionReciveData ActionDeployObject()
override void OnVariablesSynchronized()
protected vector m_TriggerOrientation
protected TrapTrigger m_TrapTrigger
void SetWireType(int wireType)
override string GetLoopDeploySoundset()
void DeferredEnableTrigger()
void UpdateProxySelections()
string m_AnimationPhaseSet
override void OnInventoryEnter(Man player)
enum SoundTypeTrap SPAWN_FLAGS
override void SetTakeable(bool pState)
Serialization general interface. Serializer API works with:
override void SetupTrapPlayer(PlayerBase player, bool set_position=true)
class Hatchback_02_Blue extends Hatchback_02 OnDebugSpawn
override string GetDeploySoundset()
class JsonUndergroundAreaTriggerData GetPosition
Wrapper class for managing sound through SEffectManager.
override void OnItemLocationChanged(EntityAI old_owner, EntityAI new_owner)
protected bool m_ResultOfAdvancedPlacing
InventoryMode
NOTE: PREDICTIVE is not to be used at all in multiplayer.
override void CreateTrigger()
override void SetInactive(bool stop_timer=true)
override void OnPlacementComplete(Man player, vector position="0 0 0", vector orientation="0 0 0")
InventoryLocationType
types of Inventory Location
void AddAction(typename actionName)
override void EEItemLocationChanged(notnull InventoryLocation oldLoc, notnull InventoryLocation newLoc)
override void GetDebugActions(out TSelectableActionInfoArrayEx outputList)
override bool OnStoreLoad(ParamsReadContext ctx, int version)
void SetIsPlaceSound(bool is_place_sound)
string m_AnimationPhaseGrounded
override bool OnAction(int action_id, Man player, ParamsReadContext ctx)
protected vector m_TriggerPosition
override void StartActivate(PlayerBase player)
void SetState(int state_ID)
override void SetActions()
override void RefreshState()
override void EEKilled(Object killer)
string m_InfoActivationTime
override bool IsTakeable()
enum eWireMaterial FOLDED
const int SAT_DEBUG_ACTION
int GetState()
returns one of STATE_...
override bool CanReceiveAttachment(EntityAI attachment, int slotId)
string m_AnimationPhaseTriggered
override void EEItemDetached(EntityAI item, string slot_name)
Param4< int, int, string, int > TSelectableActionInfoWithColor
Manager class for managing Effect (EffectParticle, EffectSound)
override void EEItemAttached(EntityAI item, string slot_name)
override void StartDeactivate(PlayerBase player)
override void OnPlacementCancelled(Man player)
override bool IsDeployable()
override bool CanDisplayAttachmentSlot(int slot_id)
private int m_WireMaterial
override void OnStoreSave(ParamsWriteContext ctx)