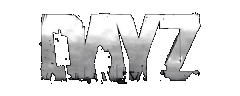 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
49 RegisterNetSyncVariableBool(
"m_CookingIsDone");
50 RegisterNetSyncVariableBool(
"m_CookingIsEmpty");
51 RegisterNetSyncVariableBool(
"m_CookingIsBurned");
64 super.EEDelete( parent );
72 super.EECargoIn(item);
74 MiscGameplayFunctions.SoakItemInsideParentContainingLiquidAboveThreshold(
ItemBase.Cast(item),
this);
91 super.OnRPC(sender, rpc_type, ctx);
93 Param1<bool> p =
new Param1<bool>(
false);
121 super.OnVariablesSynchronized();
164 switch (cooking_method)
245 vector localPos = MiscGameplayFunctions.GetSteamPosition(GetHierarchyParent());
291 sound.SetAutodestroy(
true);
297 string surfaceType =
GetGame().GetPlayer().GetSurfaceType();
298 string soundSet =
"";
300 bool diggable =
GetGame().IsSurfaceDigable(surfaceType);
304 soundSet = GetEmptyingLoopSoundsetHard();
308 soundSet = GetEmptyingLoopSoundsetSoft();
310 else if (
GetGame().SurfaceIsPond(pos[0], pos[2]) ||
GetGame().SurfaceIsSea(pos[0], pos[2]))
312 soundSet = GetEmptyingLoopSoundsetWater();
321 string surfaceType =
GetGame().GetPlayer().GetSurfaceType();
322 string soundSet =
"";
324 bool diggable =
GetGame().IsSurfaceDigable(surfaceType);
328 soundSet = GetEmptyingEndSoundsetHard();
332 soundSet = GetEmptyingEndSoundsetSoft();
334 else if (
GetGame().SurfaceIsPond(pos[0], pos[2]) ||
GetGame().SurfaceIsSea(pos[0], pos[2]))
336 soundSet = GetEmptyingEndSoundsetWater();
342 string GetPouringSoundset();
343 string GetEmptyingLoopSoundsetHard();
344 string GetEmptyingLoopSoundsetSoft();
345 string GetEmptyingLoopSoundsetWater();
346 string GetEmptyingEndSoundsetHard();
347 string GetEmptyingEndSoundsetSoft();
348 string GetEmptyingEndSoundsetWater();
356 override void SetActions()
const string SOUND_BOILING_EMPTY
void StopEmptyingLoopSound()
proto native CGame GetGame()
protected int PARTICLE_BAKING_DONE
protected int m_ParticlePlaying
void RemoveAudioVisuals()
protected CookingMethodType m_CookingMethod
float GetLiquidEmptyRate()
Returns base liquid empty rate (absolute)..preferrably use the 'GetLiquidThroughputCoef' instead.
protected int PARTICLE_DRYING_DONE
Legacy way of using particles in the game.
protected int PARTICLE_BAKING_START
const string SOUND_BAKING_DONE
protected bool m_CookingIsEmpty
override void EEDelete(EntityAI parent)
const private float QUANTITY_EMPTIED_PER_SEC_DEFAULT
void RefreshAudioVisualsOnClient(CookingMethodType cooking_method, bool is_done, bool is_empty, bool is_burned)
const string SOUND_BOILING_START
const string SOUND_DRYING_DONE
override void OnVariablesSynchronized()
protected void SoundCookingStart(string sound_name)
protected int PARTICLE_BOILING_START
override void OnDebugSpawn()
const string SOUND_BOILING_DONE
Serialization general interface. Serializer API works with:
The class that will be instanced (moddable)
protected int PARTICLE_BOILING_DONE
void ParticleCookingStop()
class JsonUndergroundAreaTriggerData GetPosition
Wrapper class for managing sound through SEffectManager.
void ParticleCookingStart(int particle_id)
override void EECargoIn(EntityAI item)
string GetEmptyingLoopSoundset()
protected int PARTICLE_DRYING_START
protected int PARTICLE_BOILING_EMPTY
void StopPouringLoopSound()
void AddAction(typename actionName)
override void Synchronize()
void PlayEmptyingLoopSound()
void PlayPouringLoopSound()
protected int PARTICLE_BURNING_DONE
void RemoveAudioVisualsOnClient()
const string SOUND_BAKING_START
protected void SoundCookingStop()
const string SOUND_BURNING_DONE
protected bool m_CookingIsBurned
protected bool m_CookingIsDone
override void OnRPC(PlayerIdentity sender, int rpc_type, ParamsReadContext ctx)
ActionFertilizeSlotCB ActionExtinguishFireplaceByLiquid
void RefreshAudioVisuals(CookingMethodType cooking_method, bool is_done, bool is_empty, bool is_burned)
void ParticleManager(ParticleManagerSettings settings)
Constructor (ctor)
string GetEmptyingEndSoundset()
Manager class for managing Effect (EffectParticle, EffectSound)
enum SoundTypeBottle m_ParticleCooking
protected EffectSound m_EmptyingLoopSound
protected EffectSound m_PouringLoopSound
const string SOUND_DRYING_START