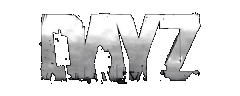 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
22 proto native
void SetDisabled(
bool pState);
25 proto
void GetMovement(out
float pSpeed, out
vector pLocalDirection);
28 proto native
float GetHeadingAngle();
31 proto native
vector GetAimChange();
34 proto native
vector GetAimDelta(
float dt);
37 proto native
vector GetTracking();
40 proto native
bool CameraViewChanged();
43 proto native
bool CameraIsFreeLook();
46 proto native
void ResetFreeLookToggle();
49 proto native
bool CameraIsTracking();
52 proto native
bool Camera3rdIsRightShoulder();
57 proto native
bool IsStanceChange();
60 proto native
bool IsJumpClimb();
66 proto native
bool IsMeleeEvade();
69 proto native
bool IsMeleeFastAttackModifier();
72 proto native
int IsMeleeLREvade();
75 proto native
bool IsMeleeWeaponAttack();
80 proto native
bool WeaponWasRaiseClick();
83 proto native
bool IsWeaponRaised();
86 proto native
bool WeaponADS();
89 proto native
void ResetADS();
92 proto native
bool IsThrowingModeChange();
95 proto native
void ResetThrowingMode();
98 proto native
bool IsWalkToggled();
106 proto native
bool IsUseButton();
112 proto native
bool IsUseButtonDown();
115 proto native
bool IsUseItemButton();
117 proto native
bool IsUseItemButtonDown();
120 proto native
bool IsAttackButton();
122 proto native
bool IsAttackButtonDown();
125 proto native
bool IsSingleUse();
128 proto native
bool IsContinuousUse();
131 proto native
bool IsContinuousUseStart();
134 proto native
bool IsContinuousUseEnd();
138 proto native
bool IsImmediateAction();
144 proto native
bool IsReloadOrMechanismSingleUse();
147 proto native
bool IsReloadOrMechanismContinuousUse();
150 proto native
bool IsReloadOrMechanismContinuousUseStart();
153 proto native
bool IsReloadOrMechanismContinuousUseEnd();
156 proto native
bool IsZoom();
159 proto native
bool IsZoomToggle();
162 proto native
void ResetZoomToggle();
165 proto native
bool IsSightChange();
168 proto native
bool IsZoomIn();
171 proto native
bool IsZoomOut();
174 proto native
bool IsFireModeChange();
177 proto native
bool IsZeroingUp();
180 proto native
bool IsZeroingDown();
183 proto native
bool IsHoldBreath();
186 proto native
void ResetHoldBreath();
192 proto native
int IsGestureSlot();
195 proto native
bool IsOtherController();
200 proto native
int IsQuickBarSlot();
204 proto native
bool IsQuickBarSingleUse();
208 proto native
bool IsQuickBarContinuousUse();
212 proto native
bool IsQuickBarContinuousUseStart();
216 proto native
bool IsQuickBarContinuousUseEnd();
224 proto native
void LimitsDisableSprint(
bool pDisable);
227 proto native
bool LimitsIsSprintDisabled();
318 proto native
void Cancel();
349 static const int STATE_NONE = 0;
352 static const int STATE_LOOP_IN = 1;
353 static const int STATE_LOOP_LOOP = 2;
354 static const int STATE_LOOP_END = 3;
355 static const int STATE_LOOP_END2 = 4;
356 static const int STATE_LOOP_LOOP2 = 5;
357 static const int STATE_LOOP_ACTION = 6;
360 static const int STATE_NORMAL = 7;
374 case STATE_NONE:
return "NONE";
376 case STATE_LOOP_IN:
return "LOOP_IN";
377 case STATE_LOOP_LOOP:
return "LOOP_LOOP";
378 case STATE_LOOP_END:
return "LOOP_END";
379 case STATE_LOOP_END2:
return "LOOP_END2";
380 case STATE_LOOP_LOOP2:
return "LOOP_LOOP2";
381 case STATE_LOOP_ACTION:
return "LOOP_ACTION";
384 case STATE_NORMAL:
return "ONE TIME";
403 void OnAnimationEvent(
int pEventID) {};
406 void OnFinish(
bool pCanceled) {};
409 void OnStateChange(
int pOldState,
int pCurrentState) {};
423 bool IsSymptomCallback()
440 proto native
float GetCurrentMovementAngle();
445 proto
bool GetCurrentInputAngle(out
float pAngle);
448 proto native
float GetCurrentMovementSpeed();
451 proto native
bool IsChangingStance();
454 proto native
bool IsOnBack();
457 proto native
bool IsInRoll();
460 proto native
bool IsLeavingUncon();
463 proto native
bool IsStandingFromBack();
466 proto native
void StartMeleeEvade();
469 proto native
void StartMeleeEvadeA(
float pDirAngle);
472 proto native
bool IsMeleeEvade();
475 proto native
void SetMeleeBlock(
bool pBlock);
479 proto native
void ForceStance(
int pStanceIdx);
484 proto native
void ForceStanceUp(
int pStanceIdx);
488 proto native
void SetRunSprintFilterModifier(
float value);
491 proto native
void SetDirectionFilterModifier(
float value);
494 proto native
void SetDirectionSprintFilterModifier(
float value);
497 proto native
void SetTurnSpanModifier(
float value);
500 proto native
void SetTurnSpanSprintModifier(
float value);
503 proto native
void SetCurrentWaterLevel(
float value);
523 proto native
bool WasHit();
526 proto native
void Cancel();
541 static const int HIT_TYPE_LIGHT = 0;
542 static const int HIT_TYPE_HEAVY = 1;
543 static const int HIT_TYPE_FINISHER = 2;
544 static const int HIT_TYPE_FINISHER_NECK = 3;
547 proto native
void ContinueCombo(
bool pHeavyHit,
float pComboValue,
EntityAI target =
null,
vector hitPos =
vector.Zero);
550 proto native
bool IsInComboRange();
553 proto native
bool WasHit();
556 proto native
void Cancel();
559 proto native
bool IsOnBack();
561 proto native
int GetComboCount();
563 proto native
int GetCurrentHitType();
565 proto native
bool IsFinisher();
577 static const int LANDTYPE_NONE = 0;
578 static const int LANDTYPE_LIGHT = 1;
579 static const int LANDTYPE_MEDIUM = 2;
580 static const int LANDTYPE_HEAVY = 3;
586 proto native
void Land(
int pLandType);
600 void OnSimulationEnd() {};
603 bool ShouldSimulationBeDisabled() {
return true; };
624 proto native
void WakeUp(
int targetStance = -1);
625 proto native
bool IsWakingUp();
627 proto native
bool IsOnLand();
628 proto native
bool IsInWater();
650 proto native
bool CanExit();
653 proto native
void Exit();
656 proto native
static bool DebugDrawLadder(Building pBuilding,
int pLadderIndex);
659 proto native
static int DebugGetLadderIndex(
string pComponentName);
663 proto native
vector GetLogoutPosition();
681 proto native
static vector WaterLevelCheck(Human pHuman,
vector pPosition);
695 proto native
int GetVehicleClass();
696 proto native
int GetVehicleSeat();
697 proto native
void SetVehicleType(
int pVehicleType);
698 proto native
int GetVehicleType();
700 proto native
void GetOutVehicle();
701 proto native
void KnockedOutVehicle();
702 proto native
bool ShouldBeKnockedOut();
703 proto native
void JumpOutVehicle();
704 proto native
void SwitchSeat(
int pTransportPositionIndex,
int pVehicleSeat);
705 proto native
bool IsGettingIn();
706 proto native
bool IsGettingOut();
707 proto native
bool IsSwitchSeat();
708 proto native
bool WasGearChange();
709 proto native
void SetClutchState(
bool pState);
710 proto native
void KeepInVehicleSpaceAfterLeave(
bool pState);
712 bool IsObjectIgnoredOnGettingOut(
IEntity entity)
715 if (!
Class.CastTo(
object, entity))
726 if (
object == transport ||
object.
GetParent() == transport)
731 return transport.IsIgnoredObject(
object);
740 class SHumanCommandClimbResult
777 proto native
int GetState();
780 proto native
vector GetGrabPointWS();
783 proto native
vector GetClimbOverStandPointWS();
787 proto native
static bool DoClimbTest(Human pHuman, SHumanCommandClimbResult pResult,
int pDebugDrawLevel);
791 proto native
static bool DebugDrawClimb(Human pHuman,
int pLevel);
859 enum WeaponActionMechanismTypes
943 case WeaponActions.MECHANISM:
return typename.EnumToString(WeaponActionMechanismTypes, AT);
992 proto native
bool IsActionFinished();
995 proto native
int GetRunningAction();
998 proto native
int GetRunningActionType();
1001 proto native
void SetActionProgressParams(
float pStart,
float pEnd);
1004 proto native
bool StartAction(
WeaponActions pAction,
int pActionType);
1007 proto native
void RegisterEvent(
string pName,
int pId);
1010 proto native
int IsEvent();
1013 proto native
bool IsInWeaponReloadBulletSwitchState();
1016 proto native
void SetADS(
bool pState);
1019 proto native
void LiftWeapon(
bool pState);
1022 void RegisterDefaultEvents()
1057 proto native
void SetInitState(
int pFrameIndex);
1060 proto native
static void StaticSetInitState(Human pHuman,
int pFrameIdx);
1067 proto native
float GetBaseAimingAngleUD();
1070 proto native
float GetBaseAimingAngleLR();
1075 proto native
void SetThrowingMode(
bool pState);
1077 proto native
bool IsThrowingMode();
1079 proto native
void ThrowItem(
int throwType);
1081 proto native
bool WasItemLeaveHandsEvent();
1087 proto native
int DebugIsEvent();
1090 proto native
void DebugResetEvents();
1103 proto native
void SetInjured(
float pValue,
bool pInterpolate);
1106 proto native
void SetExhaustion(
float pValue,
bool pInterpolate);
1127 int m_CommandTypeId;
1132 int m_LocalMovement = -1;
1141 bool IsRaisedInProne()
1153 bool IsInRaisedProne()
1173 class HumanCommandScript
1264 class Human
extends Man
class HumanInputController TAnimGraphCommand
bool PostPhysUpdate(float pDt)
@ RELOADRIFLE_CLIP_NOBULLET
class HumanAnimInterface HumanCommandActionCallback()
proto native HumanCommandDamage GetCommandModifier_Damage()
@ UNJAMMING_START
unjam action types
enum WeaponActionReloadClipTypes MECHANISM_CLOSED_UNCOCKED
vector m_ClimbGrabPointNormal
grab point for climb && climb over (in local space of it's parent)
proto native void SetFlagFinished(bool pFinished)
this terminates human command script and shows CommandHandler( ... pCurrentCommandFinished == true );
proto native HumanCommandFall GetCommand_Fall()
@ CHAMBERING_CROSSBOW_OPENED
@ ONE_FRAME
Permenantly active until DISABLED is passed.
proto native int GetCommandModifierID(int pIndex)
returns COMMANDID_ .. type id of command modifier on index pIndex
@ HIDESHOW_SLOT_2HDRIGHTBACK
class HumanCommandWeapons HumanCommandAdditives()
proto native bool IsDeathProcessed()
void OnCommandMelee2Finish()
void OnCommandMeleeStart()
proto native bool PrePhys_IsTag(int pTag)
proto native void SetAligning(vector pPositionWS, vector pDirectionWS)
enables character aligning to desired position and direction in world space
proto native HumanCommandDamage AddCommandModifier_Damage(int pType, float pDirection)
— modifier for light Damages
proto native HumanCommandLadder GetCommand_Ladder()
proto native bool IsLanding()
returns true if fall is in landing state
string GetStateString()
returns debug string of current state
proto native int GetState()
returns one of STATE_...
class HumanCommandMove HumanCommandMelee()
proto native void RegisterAnimationEvent(string pAnimationEventStr, int pId)
registers animation event, when event registered and received - it sends OnAnimationEvent with regist...
void OnCommandMoveStart()
@ CHAMBERINGLOADER_OPENED
void OnCommandLadderFinish()
void OnCommandVehicleFinish()
void PrePhysUpdate(float pDt)
private void ~HumanCommandMelee()
enum WeaponActions HumanCommandFullBodyDamage
@ RELOADRIFLE_MAGAZINE_DETACH
class HumanCommandDeathCallback HumanCommandDeath()
class HumanMovementState OnActivate()
HumanCommandScript fully scriptable command.
string WeaponActionTypeToString(int A, int AT)
proto native void GetMovementState(HumanMovementState pState)
returns movement state (current command id, )
void OnCommandClimbFinish()
proto native void DeleteCommandModifier_Action(HumanCommandActionCallback pCallback)
force remove - normally if action is ended or interrupted - this is not needed to call
@ RELOADRIFLE_MAGAZINE_BULLET
reload action types - rifles
proto native HumanCommandScript StartCommand_Script(HumanCommandScript pHumanCommand)
— SCRIPTED COMMANDS
@ RELOADSRIFLE_NOMAGAZINE_BULLET
void OnCommandMeleeFinish()
@ RELOADRIFLE_NOMAGAZINE_NOBULLET_OPEN
@ CHAMBERING_STARTLOOPABLE_SHOTGUN_UNCOCKED
proto native void SetInjured(float pValue, bool pInterpolate)
sets injury level 0..1, interpolate == false -> resets the value, otherwise it's interpolating toward...
proto native HumanCommandDeathCallback GetCommand_Death()
void OnCommandSwimFinish()
proto native HumanCommandFullBodyDamage GetCommand_Damage()
vector m_ClimbStandPoint
normal to grabpoint position (used for character orientation)
private void ~HumanCommandAdditives()
enum WeaponActionReloadClipTypes MECHANISM_SPECIAL
proto native void ResetDeath()
proto native void PostPhys_GetPosition(out vector pOutTransl)
script function usable in PostPhysUpdate
proto native void StartModifier(int pType)
starts modifier
proto float CollisionMoveTest(vector dir, vector offset, float xzScale, IEntity ignoreEntity, out IEntity hitEntity, out vector hitPosition, out vector hitNormal)
makes test if character can physically move in given direction - length of dir means distance,...
void OnCommandSwimStart()
proto native void PrePhys_SetRotation(float pInRot[4])
WeaponActionUnjammingTypes
proto native void EnableCancelCondition(bool pEnable)
proto native void SetExhaustion(float pValue, bool pInterpolate)
sets exhaustion level 0..1, interpolate == false -> resets the value, otherwise it's interpolating to...
bool IsUserActionCallback()
@ CHAMBERING_ONEBULLET_CLOSED_UNCOCKED
proto native void EnableStateChangeCallback()
enables state change callback OnStateChange
private void ~HumanCommandActionCallback()
void OnCommandVehicleStart()
private void ~HumanCommandDamage()
proto native bool IsOnBack()
return true if prone is on back
@ RELOADPISTOL_MAGAZINE_BULLET_CLOSED
proto native bool PrePhys_IsEvent(int pEvent)
script function usable in PrePhysUpdate
@ RELOADPISTOL_NOMAGAZINE_NOBULLET_CLOSED_UNCOCKED
proto native HumanCommandLadder StartCommand_Ladder(Building pBuilding, int pLadderIndex)
--— LADDER --—
proto native void PhysicsSetRagdoll(bool pEnable)
Sets and synchronize interaction layers 'RAGDOLL' and 'RAGDOLL_NO_CHARACTER' to prevent body stacking...
void OnCommandLadderStart()
enum HumanMoveCommandID GetTransformWS(out vector pTm[4])
gets human transform in World Space
proto native void ContinueCombo()
marks command to continue to combo
@ RELOADSRIFLE_MAGAZINE_NOBULLET
proto native void PrePhys_SetTranslation(vector pInTransl)
proto native HumanCommandFall StartCommand_Fall(float pYVelocity)
--— FALL --—
proto native int GetCurrentCommandID()
returns current command ID (see DayZPlayerConstants.COMMANDID_...)
IEntity m_ClimbStandPointParent
parent of grabpoint
proto native void DeleteCommandModifier_Damage(HumanCommandDamage pDamage)
proto native HumanCommandAdditives GetCommandModifier_Additives()
default (always-on modifiers)
vector m_ClimbOverStandPoint
where climb ends (in local space of it's parent)
proto native HumanCommandMelee StartCommand_Melee(EntityAI pTarget)
--— MELEE --—
@ RELOADRIFLE_NOMAGAZINE_NOBULLET
proto native HumanCommandMelee2 StartCommand_Melee2(EntityAI pTarget, int pHitType, float pComboValue, vector hitPos=vector.Zero)
starts command - melee2
proto native void PreAnim_SetBool(int pVar, bool pBool)
@ CHAMBERING_TWOBULLETS_START
void OnCommandFallStart()
@ HIDESHOW_SLOT_1HDRIGHTBACK
proto native HumanItemAccessor GetItemAccessor()
proto native bool IsDeathConditionMet()
@ HIDESHOW_SLOT_RFLLEFTBACK
IEntity m_GrabPointParent
where climb over ends (in local space of it's parent)
proto native HumanAnimInterface GetAnimInterface()
returns animation interface - usable in HumanCommandScript implementations
bool CanChangeStance(int previousStance, int newStance)
Called by code to see if it can.
void OnCommandActionFullbodyFinish()
class HumanCommandUnconscious HumanCommandDamage()
int m_iStanceIdx
current command's id
proto native void PhysicsSetSolid(bool pSolid)
proto native HumanCommandSwim StartCommand_Swim()
--— LADDER --—
@ RELOADPISTOL_NOMAGAZINE_NOBULLET_CLOSED_COCKED
void OnCommandActionFullbodyStart()
proto native void RegisterEvent(string pName, int pId)
register events
proto native void CancelModifier()
cancels modifier
@ RELOADPISTOL_MAGAZINE_NOBULLET_CLOSED_UNCOCKED
proto native void StartDeath()
proto native HumanCommandMelee2 GetCommand_Melee2()
@ HIDESHOW_SLOT_RFLRIGHTBACK
@ HIDESHOW_SLOT_2HDLEFTBACK
proto native void Cancel()
cancels action
class HumanCommandMelee2 HumanCommandFall()
proto native owned string DebugGetItemAnimInstance()
returns current item's animation instance
proto native HumanCommandActionCallback GetCommandModifier_Action()
returns callback for action if any is active, null if nothing runs
proto native bool DefaultCancelCondition()
system implemented cancel condition (now raise or sprint cancels action)
DayZPlayerConstants
defined in C++
proto native void SetTalking(bool pValue)
sets talking
proto native void ResetAligning()
disables character aligning
void OnCommandDamageAdditiveStart()
void OnRollStart(bool isToTheRight)
@ RELOADRIFLE_MAGAZINE_NOBULLET_OPEN
void OnCommandMelee2Start()
proto native HumanInputController GetInputController()
returns human input controller
proto native owned string DebugGetItemSuperClass()
returns current item's class that is found in config
@ CHAMBERING_STARTLOOPABLE_SHOTGUN_COCKED
proto native int GetBoneIndexByName(string pBoneName)
returns bone index for a name (-1 if pBoneName doesn't exist)
@ RELOADSRIFLE_NOMAGAZINE_NOBULLET
enum WeaponActionReloadClipTypes MECHANISM_OPENED
mechanism action types
@ RELOADSRIFLE_MAGAZINE_BULLET
void OnCommandDeathStart()
void OnCommandUnconsciousStart()
Base native class for all motorized wheeled vehicles.
@ CHAMBERING_ONEBULLET_UNIQUE_CLOSED
class HumanCommandLadder HumanCommandSwim()
proto native HumanCommandActionCallback AddCommandModifier_Action(int pActionID, typename pCallbackClass)
adds action command modifier, creates callback instance for you
void OnCommandDeathFinish()
proto native void PostPhys_SetRotation(float pInRot[4])
vec3 in world space
proto native void PostPhys_SetPosition(vector pInTransl)
quaternion in world space
proto native HumanCommandActionCallback GetCommand_Action()
is human is in command action - returns its callback, if current command is action
proto native bool WasHit()
is true only once after hit event
@ HIDESHOW_SLOT_PISTOLCHEST
proto native bool CheckFreeSpace(vector localDir, float distance, bool useHeading, vector posOffset=vector.Zero, float xzScale=1.0)
makes test if there's enough space for character's collider assuming that character can be pushed awa...
proto native HumanCommandUnconscious StartCommand_Unconscious(float pType)
starts command - unconscious
void OnCommandFallFinish()
private void ~HumanCommandFullBodyDamage()
proto native void LinkToLocalSpaceOf(notnull IEntity child, vector pLocalSpaceMatrix[4])
proto native owned string DebugGetItemClass()
returns current item's class name
proto native bool PhysicsIsSolid()
IEntity m_ClimbOverStandPointParent
@ RELOADRIFLE_MAGAZINE_NOBULLET
proto native bool IsInComboRange()
returns true if hit is in range, where person can continue to combo
@ CHAMBERINGLOADER_CLOSED
proto native HumanCommandFullBodyDamage StartCommand_Damage(int pType, float pDirection)
--— FullBody Damages --—
@ HIDESHOW_SLOT_PISTOLBELT
proto native void PhysicsGetVelocity(out vector pVelocity)
outs pVelocity - linear velocity of PHYSICS CONTROLLER
proto native HumanCommandSwim GetCommand_Swim()
proto native HumanCommandScript GetCommand_Script()
is human is in command action - returns its callback, if current command is action
proto native HumanCommandScript StartCommand_ScriptInst(typename pCallbackClass)
@ CHAMBERING_TWOBULLETS_END
proto native HumanCommandActionCallback StartCommand_Action(int pActionID, typename pCallbackClass, int pStanceMask)
--— ACTIONS --—
proto native void ResetDeathCooldown()
@ CHAMBERING_STARTLOOPABLE_CLOSED_KEEP
void OnDeactivate()
called when command ends
class HumanCommandVehicle m_bIsClimb
result from static test
private void ~HumanCommandFall()
proto native void Land(int pLandType)
end fall with land
proto native void PreAnim_CallCommand(int pCommand, int pParamInt, float pParamFloat)
function usable in PreAnimUpdate or in !!! OnActivate !!!
proto native HumanCommandClimb StartCommand_Climb(SHumanCommandClimbResult pClimbResult, int pType)
--— CLIMB --—
@ CHAMBERING_CROSSBOW_FULL
void OnStanceChange(int previousStance, int newStance)
gets called on stance change
proto native HumanCommandMove StartCommand_Move()
--— MOVE --—
proto native bool IsModifierActive()
is modifier active
enum WeaponActionReloadClipTypes MECHANISM_CLOSED
void PreAnimUpdate(float pDt)
proto native Human GetHuman()
get the human this cb belongs to
proto native void PreAnim_SetFloat(int pVar, float pFlt)
proto native HumanCommandDeathCallback StartCommand_Death(int pType, float pDirection, typename pCallbackClass, bool pKeepInLocalSpaceAfterLeave=false)
--— Death --—
proto native bool PrePhys_GetTranslation(out vector pOutTransl)
@ RELOADPISTOL_NOMAGAZINE_BULLET_CLOSED
@ RELOADPISTOL_NOMAGAZINE_NOBULLET_OPENED
void OnCommandUnconsciousFinish()
@ RELOADPISTOL_MAGAZINE_NOBULLET_OPENED
proto native void PreAnim_SetFilteredHeading(float pYawAngle, float pFilterDt, float pMaxYawSpeed)
sets character rotation (heading)
@ HIDESHOW_SLOT_INVENTORY
void OnCommandClimbStart()
WeaponActionReloadClipTypes
private void ~HumanCommandSwim()
proto native HumanCommandVehicle GetCommand_Vehicle()
proto native bool PhysicsIsFalling(bool pValidate)
returns true if physics controller is falling
proto native bool PhysicsLanded()
this is true when fall has physically landed - need to call Land after this is true
@ HIDESHOW_SLOT_KNIFEBACK
WeaponActionChamberingLoaderTypes
@ HIDESHOW_SLOT_1HDLEFTBACK
void OnCommandActionAdditiveFinish()
proto native HumanCommandUnconscious GetCommand_Unconscious()
void OnCommandDamageFullbodyFinish()
private void HumanItemAccessor()
@ RELOADPISTOL_MAGAZINE_NOBULLET_CLOSED_COCKED
proto native int GetCommandModifierCount()
WeaponActionChamberingTypes
proto native void PostPhys_GetRotation(out float pOutRot[4])
vec3 in world space
proto native void StopSwimming()
@ CHAMBERING_ONEBULLET_UNIQUE_OPENED
private void ~HumanCommandDeath()
proto native HumanCommandVehicle StartCommand_Vehicle(Transport pTransport, int pTransportPositionIndex, int pVehicleSeat, bool fromUnconscious=false)
--— VEHICLE --—
proto native HumanCommandWeapons GetCommandModifier_Weapons()
returns interface for handling weapons
proto native bool PrePhys_GetRotation(out float pOutRot[4])
Super root of all classes in Enforce script.
void OnCommandActionAdditiveStart()
@ RELOADRIFLE_CLIP_BULLET
void OnCommandDamageAdditiveFinish()
float m_fLeaning
current movement (0 idle, 1 walk, 2-run, 3-sprint), only if the command has a movement
@ CHAMBERING_ONEBULLET_OPENED
chambering action types
@ CHAMBERING_ONEBULLET_CLOSED
proto native HumanCommandMelee GetCommand_Melee()
proto native void InternalCommand(int pInternalCommandId)
proto native void PreAnim_SetInt(int pVar, int pInt)
@ RELOADRIFLE_NOMAGAZINE_BULLET
proto native void UnlinkFromLocalSpace()
proto native HumanCommandClimb GetCommand_Climb()
@ CHAMBERING_CROSSBOW_CLOSED
void OnCommandMoveFinish()
void OnCommandDamageFullbodyStart()
proto native void PhysicsEnableGravity(bool pEnable)
proto native HumanCommandMove GetCommand_Move()
ClimbStates
state of climb command
HumanMoveCommandID
do not process rotations !
HumanInputControllerOverrideType
@ CHAMBERING_STARTLOOPABLE_OPENED
proto native void PostPhys_LockRotation()
quaternion in world space
@ CHAMBERING_STARTLOOPABLE_CLOSED