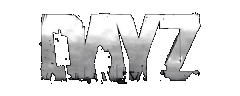 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
85 proto native
float GetSpeedometer();
88 float GetSpeedometerAbsolute()
90 return Math.AbsFloat(GetSpeedometer());
93 override bool IsAreaAtDoorFree(
int currentSeat,
float maxAllowedObjHeight = 0.5,
float horizontalExtents = 0.5,
float playerHeight = 1.7 )
99 extents[0] = horizontalExtents;
100 extents[1] = playerHeight;
101 extents[2] = horizontalExtents;
103 float speed = GetSpeedometerAbsolute();
105 extents[2] = extents[2] * 6;
109 return IsAreaAtDoorFree( currentSeat, maxAllowedObjHeight, extents, transform );
112 override Shape DebugFreeAreaAtDoor(
int currentSeat,
float maxAllowedObjHeight = 0.5,
float horizontalExtents = 0.5,
float playerHeight = 1.7 )
114 int color =
ARGB(20, 0, 255, 0);
120 extents[0] = horizontalExtents;
121 extents[1] = playerHeight;
122 extents[2] = horizontalExtents;
124 float speed = GetSpeedometerAbsolute();
126 extents[2] = extents[2] * 6;
130 if (!IsAreaAtDoorFree( currentSeat, maxAllowedObjHeight, extents, transform ))
132 color =
ARGB(20, 255, 0, 0);
135 Shape shape =
Debug.DrawBox(-extents * 0.5, extents * 0.5, color);
136 shape.SetMatrix(transform);
142 return EInventoryIconVisibility.HIDE_VICINITY;
149 proto native
float GetSteering();
156 proto native
void SetSteering(
float in,
bool analog =
false );
159 proto native
float GetThrustTurbo();
161 proto native
float GetThrustGentle();
163 proto native
float GetThrust();
171 proto native
void SetThrust(
float in,
float gentle = 0,
float turbo = 0 );
174 proto native
float GetBrake();
181 proto native
void SetBrake(
float in,
float panic = 0,
bool gentle =
false );
184 proto native
float GetHandbrake();
190 proto native
void SetHandbrake(
float in );
195 proto native
void SetBrakesActivateWithoutDriver(
bool activate =
true );
198 proto native
float GetClutch();
202 proto native
void SetClutchState(
bool in );
205 proto native
int GetGear();
207 proto native
void ShiftUp();
208 proto native
void ShiftTo( CarGear
gear );
209 proto native
void ShiftDown();
221 proto native
float GetFluidCapacity(
CarFluid fluid );
229 proto native
float GetFluidFraction(
CarFluid fluid );
232 proto native
void Leak(
CarFluid fluid,
float amount );
235 proto native
void LeakAll(
CarFluid fluid );
238 proto native
void Fill(
CarFluid fluid,
float amount );
256 proto native
float EngineGetRPMMin();
259 proto native
float EngineGetRPMIdle();
262 proto native
float EngineGetRPMMax();
265 proto native
float EngineGetRPMRedline();
268 proto native
float EngineGetRPM();
271 proto native
bool EngineIsOn();
274 proto native
void EngineStart();
291 proto native
void EngineStop();
297 proto native
vector GetEnginePos();
299 proto native
void SetEnginePos(
vector pos);
308 proto native
int GetGearsCount();
332 proto native
bool WheelIsAnyLocked();
338 proto native
bool WheelIsLocked(
int wheelIdx );
341 proto native
int WheelCount();
344 proto native
int WheelCountPresent();
384 void OnInput(
float dt ) {}
395 proto native
void ForcePosition(
vector pos );
397 proto native
void ForceDirection(
vector dir );
409 proto
float GetSteering();
416 proto
void SetSteering(
float in,
bool analog =
false );
419 proto
float GetThrustTurbo();
421 proto
float GetThrustGentle();
423 proto
float GetThrust();
431 proto
void SetThrust(
float in,
float gentle = 0,
float turbo = 0 );
434 proto
float GetBrake();
441 proto
void SetBrake(
float in,
float panic = 0 );
446 proto
void ShiftUp();
447 proto
void ShiftTo( CarGear
gear );
448 proto
void ShiftDown();
override void OnFluidChanged(CarFluid fluid, float newValue, float oldValue)
enum CarGearboxType THIRTEENTH
override float OnSound(CarSoundCtrl ctrl, float oldValue)
override int GetHideIconMask()
enum CarGearboxType FIRST
override void OnEngineStart()
Gets called everytime the engine starts.
enum CarGearboxType ELEVENTH
@ USER4
reserved for user / modding support
override bool OnBeforeEngineStart()
@ USER3
reserved for user / modding support
< h scale="0.8">< image set="dayz_gui" name="icon_pin"/> Welcome to the DayZ Stress Test Branch</h >< h scale="0.6"> This branch serves for time limited development tests that are open to the community Our goal in each of these tests is to gather performance and stability data from servers under heavy load</h ></br >< h scale="0.8">< image set="dayz_gui" name="icon_pin"/> Stress test Schedule</h >< h scale="0.6"> We ll only run the Stress Tests when our development team needs data and or specific feedback Stress Tests will be announced on our Twitter and and will usually run for a couple of hours</h ></br >< h scale="0.8">< image set="dayz_gui" name="icon_pin"/> Current Stress Test</h >< h scale="0.6"> In the first bunch of Stress we ll mostly focus on watching server performance under heavy PvP gameplay load For detailed information about an ongoing Stress please visit dayz com dev hub</h ></br >< h scale="0.8">< image set="dayz_gui" name="icon_pin"/> Important Note</h >< h scale="0.6"> Stress Tests do not represent a typical DayZ gameplay experience Spawn starting gear
override void OnContact(string zoneName, vector localPos, IEntity other, Contact data)
WARNING: Can be called very frequently in one frame, use with caution.
enum CarGearboxType TENTH
DEPRECATED class left for backwards compatibility, methods are available on car itself now.
enum CarGearboxType NINTH
CarSoundCtrl
Car's sound controller list. (native, do not change or extend)
enum CarGearboxType THIRD
@ AUTOMATIC
automatic transmission with torque converter between engine and gearbox
enum CarGearboxType SEVENTH
CarGearboxType
Enumerated gearbox types. (native, do not change or extend)
CarFluid
Type of vehicle's fluid. (native, do not change or extend)
enum CarGearboxType SIXTEENTH
enum CarGearboxType NEUTRAL
@ ENGINE
indicates if engine is ON
enum CarGearboxType SECOND
@ USER1
reserved for user / modding support
Base native class for all motorized wheeled vehicles.
override void OnGearChanged(int newGear, int oldGear)
enum CarGearboxType SIXTH
enum CarGearboxType FIFTH
enum CarGearboxType FOURTEENTH
enum CarGearboxType TWELFTH
@ MANUAL
classic manual transmission with friction plates between engine and gearbox
@ USER2
reserved for user / modding support
@ PLAYER
indicates if driver is controlled by player
@ DOORS
indicates if doors are open
enum CarGearboxType FIFTEENTH
@ SPEED
speed of the car in km/h
enum CarGearboxType EIGTH
enum CarGearboxType FOURTH
int ARGB(int a, int r, int g, int b)
class DiagMenu Shape
don't call destructor directly. Use Destroy() instead
CarAutomaticGearboxMode
Enumerated automatic gearbox modes. (native, do not change or extend)
override void OnEngineStop()
Gets called everytime the engine stops.
enum CarGearboxType REVERSE
Enumerated vehicle's gears. (native, do not change or extend)