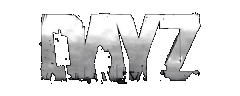 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
11 static int IKSETTING_AIMING = 0x1;
12 static int IKSETTING_RHAND = 0x2;
13 static int IKSETTING_LHAND = 0x4;
18 int m_StanceMovements[6];
19 int m_StanceRotation[6];
21 int m_IKSettingsMelee[2];
22 int m_iPerItemCameraUserData;
25 float m_fMoveHeadingFilterSpan;
26 float m_fMoveHeadingSprintFilterSpan;
27 float m_fMoveHeadingProneFilterSpan;
28 float m_fMoveHeadingFilterSpeed;
31 float m_fMeleeEvadeHeadingFilterSpan;
32 float m_fMeleeEvadeHeadingFilterSpeed;
41 private bool m_bPlaceholder;
44 void SetIK(
int pStance,
int pMovement,
bool pAim,
bool pRArm,
bool pLArm)
47 if (pAim) val |= IKSETTING_AIMING;
48 if (pRArm) val |= IKSETTING_RHAND;
49 if (pLArm) val |= IKSETTING_LHAND;
52 pStance = pStance * 4;
53 pStance = pStance + pMovement;
59 void SetIKStance(
int pStance,
bool pAim,
bool pRArm,
bool pLArm)
62 if (pAim) val |= IKSETTING_AIMING;
63 if (pRArm) val |= IKSETTING_RHAND;
64 if (pLArm) val |= IKSETTING_LHAND;
68 for (
int i = 0; i < 4; i++)
74 void SetIKMelee(
int pHitType,
bool pAim,
bool pRArm,
bool pLArm)
77 if (pAim) val |= IKSETTING_AIMING;
78 if (pRArm) val |= IKSETTING_RHAND;
79 if (pLArm) val |= IKSETTING_LHAND;
84 void SetIKAll(
bool pAim,
bool pRArm,
bool pLArm)
87 if (pAim) val |= IKSETTING_AIMING;
88 if (pRArm) val |= IKSETTING_RHAND;
89 if (pLArm) val |= IKSETTING_LHAND;
92 for (i = 0; i < 24; i++)
97 for (i = 0; i < 2; i++)
proto native void ResetWeaponInHands()
reset weapon anim override in case of premature death
class HumanItemBehaviorCfg OnItemInHandsChanged(bool pInstant=false)
signalization from script to engine that item in hands changed
proto native bool IsItemInHandsWeapon()
returns true if item in hands is a weapon
private void ~HumanItemAccessor()
proto native bool IsItemInHandsHidden()
returns if item in hands is hidden visually
proto native HumanItemBehaviorCfg GetItemInHandsBehaviourCfg()
proto native bool WeaponGetCameraPointMSTransform(notnull EntityAI pCamEntity, vector pCamPoint, vector pCamDir, out vector pTm[4])
returns true if weapon is item and it has camera point - model space matrix -
int m_IKSettingsMelee[2]
[stance][movement] mask for ik
proto native bool WeaponGetCameraPointBoneRelative(notnull EntityAI pCamEntity, vector pCamPoint, vector pCamDir, int pBoneIndex, out vector pTm[4])
returns true if weapon is item and it has camera point - model space matrix -
proto native bool WeaponGetAimingModelDirTm(out vector pTm[4])
return current aiming point from aiming model (additive swaying applied - no recoil points)
private void HumanItemAccessor()
proto native void HideItemInHands(bool pState)
hides item in hands visually
proto native bool WeaponGetCameraPoint(out vector pPos, out vector pRot)
returns true if weapon is item and it has camera point - local pos, dir in weapon space