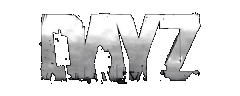 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
9 void SetByFloat(
float value);
10 void SetByFloatEx(
float value,
string system =
"" );
15 float GetNormalized();
29 protected string m_ValueLabel;
30 protected int m_Flags;
36 void PlayerStat(T min, T max,T
init,
string label,
int flags)
47 override void Init(
int id)
63 void Set( T value,
string system =
"" )
88 void SetByFloat(
float value,
string system =
"" )
94 override void SetByFloatEx(
float value,
string system =
"" )
96 SetByFloat(value, system);
106 void Add( T value,
string system =
"" )
116 override string GetLabel()
131 override float GetNormalized()
141 void CreateRecord(
float value,
string system)
proto native CGame GetGame()
void Add(string name, string value)
eBleedingSourceType m_Type
ModifiersManager m_Manager
proto native float GetMax()
Serialization general interface. Serializer API works with:
class InventoryGridController extends ScriptedWidgetEventHandler Init
proto native float GetMin()
array< ref PlayerStatBase > Get()
enum MagnumStableStateID init
void OnStoreSave(ParamsWriteContext ctx)
Super root of all classes in Enforce script.
bool OnStoreLoad(ParamsReadContext ctx, int version)