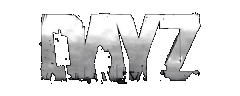 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
3 static const int FOLDED = 0;
4 static const int UNFOLDED = 1;
5 static const int FILLED = 2;
6 static const int PERCENTUAL_DAMAGE = 1;
18 RegisterNetSyncVariableInt(
"m_State",
FOLDED, FILLED );
19 RegisterNetSyncVariableBool(
"m_IsSoundSynchRemote");
20 RegisterNetSyncVariableBool(
"m_IsDeploySound");
28 override bool HasProxyParts()
35 if( !super.CanPutIntoHands( parent ) )
39 return CanBeManipulated();
49 super.OnVariablesSynchronized();
84 bool CanBeFilledAtPosition(
vector position )
87 GetGame().SurfaceGetType( position[0], position[2], surface_type );
89 return GetGame().IsSurfaceDigable(surface_type);
92 bool CanBeManipulated()
106 this.ShowSelection(
"inventory" );
107 this.HideSelection(
"placing" );
108 this.HideSelection(
"filled" );
115 SetAllowDamage(
true);
117 float fold_damage = ( GetMaxHealth(
"",
"" ) / 100 ) * PERCENTUAL_DAMAGE;
118 DecreaseHealth(
"",
"", fold_damage );
124 this.HideSelection(
"inventory" );
125 this.ShowSelection(
"placing" );
126 this.HideSelection(
"filled" );
133 SetAllowDamage(
true);
135 float unfold_damage = ( GetMaxHealth(
"",
"" ) / 100 ) * PERCENTUAL_DAMAGE;
136 DecreaseHealth(
"",
"", unfold_damage );
142 super.EEItemLocationChanged (oldLoc, newLoc);
149 super.RefreshPhysics();
151 if (
this && !ToDelete() )
153 RemoveProxyPhysics(
"inventory" );
154 RemoveProxyPhysics(
"placing" );
155 RemoveProxyPhysics(
"filled" );
163 AddProxyPhysics(
"placing" );
168 AddProxyPhysics(
"inventory" );
172 AddProxyPhysics(
"filled" );
180 this.HideSelection(
"inventory" );
181 this.HideSelection(
"placing" );
182 this.ShowSelection(
"filled" );
190 DecreaseHealth(
"",
"", 5 );
191 SetAllowDamage(
false);
197 super.OnStoreSave(ctx);
205 if ( !super.OnStoreLoad(ctx, version) )
210 if ( !ctx.Read(state) )
240 super.OnPlacementComplete( player, position, orientation );
257 return "placeHescoBox_SoundSet";
262 return "hescobox_deploy_SoundSet";
267 if ( !
GetGame().IsDedicatedServer() )
278 if ( !
GetGame().IsDedicatedServer() )
proto native CGame GetGame()
PlaceObjectActionReciveData ActionReciveData ActionDeployObject()
proto native int GetState()
returns one of STATE_...
override void EEItemLocationChanged(notnull InventoryLocation oldLoc, notnull InventoryLocation newLoc)
void Synchronize(eInjuryHandlerLevels level)
void SetIsDeploySound(bool is_deploy_sound)
override void OnVariablesSynchronized()
void PlayDeployLoopSound()
override void OnPlacementComplete(Man player, vector position="0 0 0", vector orientation="0 0 0")
override string GetLoopDeploySoundset()
Serialization general interface. Serializer API works with:
class JsonUndergroundAreaTriggerData GetPosition
Wrapper class for managing sound through SEffectManager.
override bool CanPutIntoHands(EntityAI parent)
void SetState(bool state)
void AddAction(typename actionName)
override bool IsDeployable()
protected ref EffectSound m_DeployLoopSound
void OnStoreSave(ParamsWriteContext ctx)
void StopDeployLoopSound()
bool CanPlayDeployLoopSound()
enum eWireMaterial FOLDED
Manager class for managing Effect (EffectParticle, EffectSound)
bool OnStoreLoad(ParamsReadContext ctx, int version)
override string GetDeploySoundset()
protected float m_DrainThreshold protected bool m_State