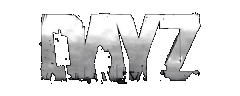 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
13 m_ToggleAnimations.Insert(
new ToggleAnimations(
"EntranceO",
"EntranceC", OPENING_0), 0 );
14 m_ToggleAnimations.Insert(
new ToggleAnimations(
"Window1O",
"Window1C", OPENING_1), 0 );
15 m_ToggleAnimations.Insert(
new ToggleAnimations(
"Window2O",
"Window2C", OPENING_2), 0 );
16 m_ToggleAnimations.Insert(
new ToggleAnimations(
"Window3O",
"Window3C", OPENING_3), 0 );
17 m_ToggleAnimations.Insert(
new ToggleAnimations(
"Window4O",
"Window4C", OPENING_4), 0 );
18 m_ToggleAnimations.Insert(
new ToggleAnimations(
"Window5O",
"Window5C", OPENING_5), 0 );
19 m_ToggleAnimations.Insert(
new ToggleAnimations(
"Window6O",
"Window6C", OPENING_6), 0 );
20 m_ToggleAnimations.Insert(
new ToggleAnimations(
"Window7O",
"Window7C", OPENING_7), 0 );
22 m_ShowAnimationsWhenPitched.Insert(
"Body" );
31 m_ShowAnimationsWhenPitched.Insert(
"Pack" );
33 m_ShowAnimationsWhenPacked.Insert(
"Inventory" );
45 super.OnRPC(sender, rpc_type, ctx);
47 Param1<bool> p =
new Param1<bool>(
false);
93 super.OnItemLocationChanged(old_owner, new_owner);
98 return "LargeTent_Door_Open_SoundSet";
103 return "LargeTent_Door_Close_SoundSet";
108 return "LargeTent_Window_Open_SoundSet";
113 return "LargeTent_Window_Close_SoundSet";
123 return "LargeTentClutterCutter";
132 super.OnPlacementComplete( player, position, orientation );
148 return "placeLargeTent_SoundSet";
153 return "largetent_deploy_SoundSet";
override string GetSoundClose()
proto native CGame GetGame()
override void OnRPC(PlayerIdentity sender, int rpc_type, ParamsReadContext ctx)
override void OnItemLocationChanged(EntityAI old_owner, EntityAI new_owner)
override string GetSoundOpenWindow()
override void OnPlacementComplete(Man player, vector position="0 0 0", vector orientation="0 0 0")
Container_Base m_HalfExtents
const int ECE_PLACE_ON_SURFACE
override string GetSoundOpen()
override string GetLoopDeploySoundset()
Serialization general interface. Serializer API works with:
The class that will be instanced (moddable)
class JsonUndergroundAreaTriggerData GetPosition
Wrapper class for managing sound through SEffectManager.
override string GetClutterCutter()
protected Object m_ClutterCutter
override bool HasClutterCutter()
override string GetSoundCloseWindow()
enum SoundTypeTent m_RepackingLoopSound
void StopRepackingLoopSound()
Manager class for managing Effect (EffectParticle, EffectSound)
proto native vector Vector(float x, float y, float z)
Vector constructor from components.
override string GetDeploySoundset()
override void SetActions()
void PlayRepackingLoopSound()