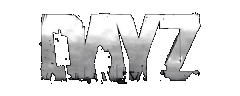 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
4 private const int GARDEN_SLOT_COUNT = 9;
18 if ( !super.OnStoreLoad(ctx, version) )
32 super.EEDelete(parent);
48 return GARDEN_SLOT_COUNT;
53 HideSelection(
"SeedBase_1");
54 HideSelection(
"SeedBase_2");
55 HideSelection(
"SeedBase_3");
56 HideSelection(
"SeedBase_4");
57 HideSelection(
"SeedBase_5");
58 HideSelection(
"SeedBase_6");
59 HideSelection(
"SeedBase_7");
60 HideSelection(
"SeedBase_8");
61 HideSelection(
"SeedBase_9");
62 HideSelection(
"slotCovered_01");
63 HideSelection(
"slotCovered_02");
64 HideSelection(
"slotCovered_03");
65 HideSelection(
"slotCovered_04");
66 HideSelection(
"slotCovered_05");
67 HideSelection(
"slotCovered_06");
68 HideSelection(
"slotCovered_07");
69 HideSelection(
"slotCovered_08");
70 HideSelection(
"slotCovered_09");
82 override void OnHologramBeingPlaced( Man player )
89 super.OnPlacementComplete( player, position, orientation );
115 GetGame().SurfaceGetType3D( position[0], position[1], position[2], surface_type );
117 return GetGame().IsSurfaceFertile(surface_type);
122 class GardenPlotPolytunnel : GardenPlot
132 HideSelection(
"SeedBase_1");
133 HideSelection(
"SeedBase_2");
134 HideSelection(
"SeedBase_3");
135 HideSelection(
"SeedBase_4");
136 HideSelection(
"SeedBase_5");
137 HideSelection(
"SeedBase_6");
138 HideSelection(
"SeedBase_7");
139 HideSelection(
"SeedBase_8");
140 HideSelection(
"SeedBase_9");
141 HideSelection(
"SeedBase_10");
142 HideSelection(
"SeedBase_11");
143 HideSelection(
"SeedBase_12");
144 HideSelection(
"SeedBase_13");
149 class GardenPlotPlacing
extends GardenPlot
proto native CGame GetGame()
override void OnPlacementStarted(Man player)
GardenPlotGreenhouse GardenPlot EOnInit(IEntity other, int extra)
override void SyncSlots()
override void RefreshSlots()
class PASBroadcaster extends AdvancedCommunication IsInventoryVisible
const int ECE_PLACE_ON_SURFACE
override void OnPlacementComplete(Man player, vector position="0 0 0", vector orientation="0 0 0")
override void EEDelete(EntityAI parent)
Serialization general interface. Serializer API works with:
class JsonUndergroundAreaTriggerData GetPosition
protected Object m_ClutterCutter
override bool CanBePlaced(Man player, vector position)
class GardenPlot extends GardenBase POLYTUNNEL_SLOT_COUNT
bool OnStoreLoad(ParamsReadContext ctx, int version)
override int GetGardenSlotsCount()