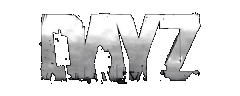 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
3 protected Widget m_FollowerRoot;
4 protected Widget m_FollowerButton;
10 protected float m_MaxFade;
11 protected float m_MinFade;
13 protected CameraToolsMenu
m_Menu;
17 DestroyFollowedObject();
18 delete m_FollowerRoot;
21 void CreateFollowedObject(
string type )
28 void DestroyFollowedObject()
30 if( m_FollowedObject )
32 GetGame().ObjectDelete( m_FollowedObject );
36 void Update(
float timeslice )
43 return m_FollowedObject;
49 if( m_FollowedObject )
51 m_FollowedObject.SetPosition( position );
70 if( m_FollowedObject && m_FollowedObject.GetPosition() !=
m_Position )
79 if( m_FollowedObject )
81 return m_FollowedObject.GetOrientation();
96 if( relativePos[0] >= 1 || relativePos[0] == 0 || relativePos[1] >= 1 || relativePos[1] == 0 )
98 m_FollowerRoot.Show(
false );
101 else if( relativePos[2] < 0 )
103 m_FollowerRoot.Show(
false );
108 m_FollowerRoot.Show(
true );
112 m_FollowerRoot.GetSize(
x,
y );
114 m_FollowerRoot.SetPos( relativePos[0], relativePos[1] );
119 m_FollowerRoot.Show(
true );
124 m_FollowerRoot.Show(
false );
127 void Fade(
bool fade )
131 m_FollowerRoot.SetAlpha( m_MinFade );
135 m_FollowerRoot.SetAlpha( m_MaxFade );
151 if( w == m_FollowerButton && button ==
MouseState.LEFT )
155 m_Menu.SelectActor( CTActor.Cast(
this ) );
164 if( w == m_FollowerButton && button ==
MouseState.LEFT )
168 m_Menu.SelectActor(
null );
proto native CGame GetGame()
protected vector m_Position
Cached world position.
protected ServerBrowserMenuNew m_Menu
class JsonUndergroundAreaTriggerData GetPosition
override bool OnDoubleClick(Widget w, int x, int y, int button)
bool OnMouseButtonDown(Widget w, int x, int y, int button)
proto native void SetPosition(vector position)
Set the world position of the Effect.
override bool OnClick(Widget w, int x, int y, int button)
buttons clicks