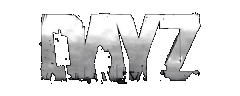 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
22 data.MissionPaths = {dzg.GetMissionFolderPath()};
23 if (!JsonFileLoader<JsonMissionLoaderData>.SaveFile(
path, data, errorMessage))
28 if (!JsonFileLoader<JsonMissionLoaderData>.LoadFile(
path, data, errorMessage))
49 layoutRoot =
GetGame().GetWorkspace().CreateWidgets(
"gui/layouts/day_z_mission_loader.layout");
51 m_WgtLstMsnList = TextListboxWidget.Cast( layoutRoot.FindAnyWidget(
"wgt_lst_missions") );
52 m_WgtBtnMsnPlay = ButtonWidget.Cast( layoutRoot.FindAnyWidget(
"wgt_btn_mission_play") );
53 m_WgtBtnMsnClose = ButtonWidget.Cast( layoutRoot.FindAnyWidget(
"wgt_btn_mission_close") );
65 super.OnClick(w,
x,
y, button);
76 string missionPath =
m_MissionData.MissionPaths.Get(rowIndex);
77 GetGame().PlayMission(missionPath);
86 super.OnClick(w,
x,
y, button);
91 string missionPath =
m_MissionData.MissionPaths.Get(rowIndex);
92 GetGame().PlayMission(missionPath);
99 super.OnKeyDown(w,
x,
y,key);
proto native CGame GetGame()
override Widget Init()
Launched from 'DayZGame.DeferredInit' to make earlier access, use, and updates impossible (downside o...
proto bool GetCLIParam(string param, out string val)
Returns command line argument.
override bool OnKeyDown(Widget w, int x, int y, int key)
proto bool FileExist(string name)
Check existence of file.
override bool OnClick(Widget w, int x, int y, int button)
protected ref JsonMissionLoaderData m_MissionData
override bool OnDoubleClick(Widget w, int x, int y, int button)
class JsonMissionLoaderData m_WgtLstMsnList
protected ButtonWidget m_WgtBtnMsnClose
const string CFG_FILE_MISSION_LIST
protected ref TStringArray m_ListMissionsNames
protected ButtonWidget m_WgtBtnMsnPlay