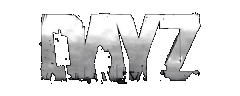 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
24 SetEnginePos(
"0 1.4 2.25");
48 super.OnEngineStart();
82 override vector GetTransportCameraOffset()
119 if (!super.CanReleaseAttachment(attachment))
124 if (EngineIsOn() && attachment.GetType() ==
"TruckBattery")
134 if (slotSelectionName ==
"wheel_spare_1")
136 return GetAnimationPhase(
"wheelSidePlate1") == 1.0);
139 if (slotSelectionName ==
"wheel_spare_2")
141 return GetAnimationPhase(
"wheelSidePlate2") == 1.0);
144 return super.CanManipulateSpareWheel(slotSelectionName);
173 return "doors_driver";
176 return "doors_codriver";
180 return super.GetDoorSelectionNameFromSeatPos(posIdx);
188 return "Truck_01_Door_1_1";
191 return "Truck_01_Door_2_1";
195 return super.GetDoorInvSlotNameFromSeatPos(posIdx);
216 return Math.Clamp(newValue, 0, 1);
220 return super.OnSound(ctrl, oldValue);
225 super.OnAnimationPhaseStarted(animSource, phase);
230 case "wheelsideplate1":
231 case "wheelsideplate2":
244 sound.SetAutodestroy(
true);
254 Class.CastTo(carDoor, FindAttachmentBySlotName(slotType));
262 case "Truck_01_Door_1_1":
264 case "Truck_01_Door_2_1":
266 case "Truck_01_Hood":
278 return "DoorsDriver";
279 case "doors_codriver":
280 return "DoorsCoDriver";
285 case "wheelsideplate1":
286 return "WheelSidePlate1";
287 case "wheelsideplate2":
288 return "WheelSidePlate2";
299 return nextSeat == 1;
301 return nextSeat == 0;
309 switch (pCurrentSeat)
312 return pDoorsSelection ==
"DoorsDriver";
315 return pDoorsSelection ==
"DoorsCoDriver";
323 switch (pDoorSelection)
328 case "DoorsCoDriver":
379 GetInventory().CreateInInventory(
"Truck_01_Wheel");
380 GetInventory().CreateInInventory(
"Truck_01_Wheel");
382 GetInventory().CreateInInventory(
"Truck_01_WheelDouble");
383 GetInventory().CreateInInventory(
"Truck_01_WheelDouble");
384 GetInventory().CreateInInventory(
"Truck_01_WheelDouble");
385 GetInventory().CreateInInventory(
"Truck_01_WheelDouble");
387 GetInventory().CreateInInventory(
"Truck_01_Door_1_1");
388 GetInventory().CreateInInventory(
"Truck_01_Door_2_1");
389 GetInventory().CreateInInventory(
"Truck_01_Hood");
392 GetInventory().CreateInInventory(
"Truck_01_Wheel");
393 GetInventory().CreateInInventory(
"Truck_01_Wheel");
394 GetInventory().CreateInInventory(
"Truck_01_WheelDouble");
395 GetInventory().CreateInInventory(
"Truck_01_WheelDouble");
proto native CGame GetGame()
protected ref UniversalTemperatureSource m_UTSource
override float OnSound(CarSoundCtrl ctrl, float oldValue)
string m_CarDoorCloseSound
ActionDetachFromTarget_SpecificSlot_WoodenPlanks ActionDetachFromTarget_SpecificSlot ActionDetachFromTarget_SpecificSlot_MetalSheets()
class Truck_01_Base extends CarScript OnDebugSpawn
override void OnEngineStart()
Gets called everytime the engine starts.
protected CarRearLightBase CreateRearLight()
ActionEnterLadder ActionDetachFromTarget_SpecificSlotsCategory_WoodenCrate
protected void SpawnAdditionalItems()
override bool CrewCanGetThrough(int posIdx)
string m_CarHornLongSoundName
protected CarLightBase CreateFrontLight()
override bool CanReleaseAttachment(EntityAI attachment)
string GetAnimSourceFromSelection(string selection)
override int GetAnimInstance()
string m_CarHornShortSoundName
original Timer deletes m_params which is unwanted
int GetCarDoorsState(string slotType)
string m_CarDoorOpenSound
CarSoundCtrl
Car's sound controller list. (native, do not change or extend)
override float GetTransportCameraDistance()
protected ref UniversalTemperatureSourceSettings m_UTSSettings
class JsonUndergroundAreaTriggerData GetPosition
Wrapper class for managing sound through SEffectManager.
protected bool CanManipulateSpareWheel(string slotSelectionName)
override int GetSeatIndexFromDoor(string pDoorSelection)
DayZPlayerConstants
defined in C++
string GetDoorSelectionNameFromSeatPos(int posIdx)
void AddAction(typename actionName)
protected void SpawnUniversalParts()
override void OnAnimationPhaseStarted(string animSource, float phase)
protected ref UniversalTemperatureSourceLambdaEngine m_UTSLEngine
override void EOnPostSimulate(IEntity other, float timeSlice)
string GetDoorInvSlotNameFromSeatPos(int posIdx)
override bool CanReachDoorsFromSeat(string pDoorsSelection, int pCurrentSeat)
string m_EngineStartBattery
protected void FillUpCarFluids()
CarDoorState TranslateAnimationPhaseToCarDoorState(string animation)
override int GetSeatAnimationType(int posIdx)
override bool CanReachSeatFromSeat(int currentSeat, int nextSeat)
Super root of all classes in Enforce script.
Manager class for managing Effect (EffectParticle, EffectSound)
string m_EngineStartOK
Sounds.
protected vector m_enginePtcPos
ActionDetachFromTarget_SpecificSlotsCategory ActionDetachFromTarget ActionDetachFromTarget_SpecificSlot_WoodenLogs()
override void OnEngineStop()
Gets called everytime the engine stops.