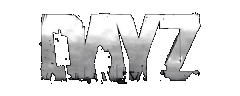 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
51 return GetGame().GetSoundScene().BuildSoundObject(
this);
54 proto native
void Initialize(
SoundParams soundParams);
56 proto native
void AddEnvSoundVariables(
vector position);
57 proto native
void AddVariable(
string name,
float value);
61 void UpdateEnvSoundControllers(
vector position)
63 AddEnvSoundVariables(position);
67 void SetVariable(
string name,
float value)
69 AddVariable(
name, value);
104 proto native
bool Load(
string name);
105 proto native
bool IsValid();
106 proto
string GetName();
109 class AbstractWaveEvents
120 private void InitEvents()
122 AbstractWaveEvents events =
new AbstractWaveEvents();
126 #ifdef DIAG_DEVELOPER
133 proto
void SetUserData(
Managed inst);
138 void PlayWithOffset(
float offset)
141 SetStartOffset(offset);
145 proto
void Restart();
146 proto
void SetStartOffset(
float offset);
148 proto
float GetLength();
150 proto
float GetCurrPosition();
151 proto
void Loop(
bool setLoop);
152 proto
float GetVolume();
153 proto
void SetVolume(
float value);
154 proto
void SetVolumeRelative(
float value);
155 proto
void SetFrequency(
float value);
156 proto
float GetFrequency();
157 proto
void SetPosition(
vector position,
vector velocity =
"0 0 0");
158 proto
void SetFadeInFactor(
float volume);
159 proto
void SetFadeOutFactor(
float volume);
160 proto
void SetDoppler(
bool setDoppler);
161 proto
void Skip(
float timeSec);
162 proto
bool IsHeaderLoaded();
164 AbstractWaveEvents GetEvents()
171 GetEvents().Event_OnSoundWaveStarted.Invoke(
this);
176 GetEvents().Event_OnSoundWaveStopped.Invoke(
this);
181 GetEvents().Event_OnSoundWaveLoaded.Invoke(
this);
186 GetEvents().Event_OnSoundWaveHeaderLoaded.Invoke(
this);
191 GetEvents().Event_OnSoundWaveEnded.Invoke(
this);
proto native float GetVOIPVolume()
proto native CGame GetGame()
ref ScriptInvoker Event_OnSoundWaveStopped
ref ScriptInvoker Event_OnSoundWaveEnded
proto native void SetSoundVolume(float vol, float time)
proto native void SetOcclusionObstruction(float occlusion, float obstruction)
proto native int GetHierarchyPivot()
ParticleSource Play(int particle_id, Object parent_obj, vector local_pos="0 0 0", vector local_ori="0 0 0")
Legacy function for backwards compatibility with 1.01 and below.
proto native void SetParent(IEntity parent, int pivot=-1)
proto native AbstractWave Play2D(SoundObject soundObject, SoundObjectBuilder soundBuilder)
proto native void SetKind(WaveKind kind)
proto native float GetSpeechExVolume()
proto native void SetRadioVolume(float vol, float time)
proto native float GetMusicVolume()
class SoundObjectBuilder SoundObject(SoundParams soundParams)
proto native vector GetSpeed()
private void ~AbstractSoundScene()
proto native void Initialize(SoundParams soundParams)
ref ScriptInvoker Event_OnSoundWaveHeaderLoaded
class SoundParams Event_OnSoundWaveStarted
proto native void SetSpeed(vector speed)
Note: Sets the speed locally if parented, retrieves globally with the parent speed.
proto native SoundObject BuildSoundObject(SoundObjectBuilder soundObjectbuilder)
proto native void SetVOIPVolume(float vol, float time)
proto native void SetMusicVolume(float vol, float time)
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
proto native AbstractWave Play3D(SoundObject soundObject, SoundObjectBuilder soundBuilder)
proto native void SetPosition(vector position)
Note: Sets the position locally if parented, retrieves globally with the sound offset.
enum WaveKind AbstractSoundScene()
proto void UpdateVariables(notnull array< float > values)
proto native vector GetPosition()
Get the world position of the Effect.
proto native float GetAudioLevel()
proto native void SetSpeechExVolume(float vol, float time)
proto native float GetRadioVolume()
proto native float GetSilenceThreshold()
proto native float GetSoundVolume()
ref ScriptInvoker Event_OnSoundWaveLoaded
ScriptInvoker Class provide list of callbacks usage: