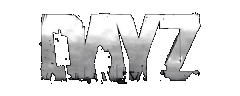 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
7 override void SetActions()
14 float GetFishingEffectivityBonus()
19 override bool IsOpen()
24 void AnimateFishingRod(
bool state) {}
26 override void OnDebugSpawn()
28 GetInventory().CreateAttachment(
"Bait");
29 for (
int i = 0; i < 6; ++i)
95 m_Player.Message(
"I have started fishing.",
"colorFriendly");
121 if ( rand <= 2 && m_Pull <= TOO_MUCH_PULL && m_Pull >=
TOO_FEW_PULL )
124 if (
Math.RandomInt(0, 1) )
152 m_Cycler.Run(0.3,
this,
"Pulling", NULL,
true);
168 m_Player.Message(
"",
"colorStatusChannel");
169 m_Player.Message(
"HOLD right mouse button to pull the fishing line",
"colorAction");
170 m_Player.Message(
"",
"colorStatusChannel");
171 m_Player.Message(
"",
"colorStatusChannel");
172 m_Player.Message(
"",
"colorStatusChannel");
173 m_Player.Message(
"Start pulling when J and <*)))>< meet:",
"colorStatusChannel");
174 m_Player.Message(
"",
"colorStatusChannel");
175 m_Player.Message(
"J"+fline+
" <*)))><",
"colorImportant");
185 float agent_speed_distance =
vector.Distance(
"0 0 0",
m_Player.GetModelSpeed());
186 if (
m_Player.GetItemInHands() !=
this || !
m_Player.IsInWater() || agent_speed_distance > 1 )
196 pcatch.SetQuantity(800,
false);
238 m_Player.Message(
"",
"colorStatusChannel");
239 m_Player.Message(
"",
"colorStatusChannel");
240 m_Player.Message(
"",
"colorStatusChannel");
241 m_Player.Message(
"The fish had broken the fishing line and swam away.",
"colorImportant");
242 m_Player.Message(
"",
"colorStatusChannel");
243 m_Player.Message(
"",
"colorStatusChannel");
244 m_Player.Message(
"",
"colorStatusChannel");
245 m_Player.Message(
"",
"colorStatusChannel");
249 m_Player.Message(
"",
"colorStatusChannel");
250 m_Player.Message(
"",
"colorStatusChannel");
251 m_Player.Message(
"",
"colorStatusChannel");
252 m_Player.Message(
"The fish escaped.",
"colorImportant");
253 m_Player.Message(
"",
"colorStatusChannel");
254 m_Player.Message(
"",
"colorStatusChannel");
255 m_Player.Message(
"",
"colorStatusChannel");
256 m_Player.Message(
"",
"colorStatusChannel");
260 m_Player.Message(
"",
"colorStatusChannel");
261 m_Player.Message(
"",
"colorStatusChannel");
262 m_Player.Message(
"",
"colorStatusChannel");
263 m_Player.Message(
"I caught the fish!",
"colorFriendly");
264 m_Player.Message(
"",
"colorStatusChannel");
265 m_Player.Message(
"",
"colorStatusChannel");
266 m_Player.Message(
"",
"colorStatusChannel");
267 m_Player.Message(
"",
"colorStatusChannel");
271 m_Player.Message(
"",
"colorStatusChannel");
272 m_Player.Message(
"",
"colorStatusChannel");
273 m_Player.Message(
"",
"colorStatusChannel");
274 m_Player.Message(
"Fishing time is over.",
"colorFriendly");
275 m_Player.Message(
"",
"colorStatusChannel");
276 m_Player.Message(
"",
"colorStatusChannel");
277 m_Player.Message(
"",
"colorStatusChannel");
278 m_Player.Message(
"",
"colorStatusChannel");
282 Print(
"Wrong number");
296 for (
int i = 0; i <
m_Pull; i++)
300 m_Player.Message(
"",
"colorStatusChannel");
301 m_Player.Message(
"HOLD right mouse button to pull the fishing line",
"colorAction");
302 m_Player.Message(
"RELEASE right mouse button to loosen the fishing line",
"colorAction");
303 m_Player.Message(
"",
"colorStatusChannel");
304 m_Player.Message(
"",
"colorStatusChannel");
305 m_Player.Message(
"Fishing line stretch :",
"colorStatusChannel");
306 m_Player.Message(
"",
"colorStatusChannel");
309 m_Player.Message(
"<"+fline+
">",
"colorImportant");
313 m_Player.Message(
"<"+fline+
">",
"colorAction");
315 if ( m_Pull < HIGH_PULL && m_Pull >
LOW_PULL)
317 m_Player.Message(
"<"+fline+
">",
"colorFriendly");
321 m_Player.Message(
"<"+fline+
">",
"colorStatusChannel");
325 m_Player.Message(
"<"+fline+
">",
"colorImportant");
332 if( !super.CanPutInCargo(parent) ) {
return false;}
override void SetActions()
proto native CGame GetGame()
const float MIN_FISHING_TIME
proto void Print(void var)
Prints content of variable to console/log.
const int ECE_PLACE_ON_SURFACE
void AddPull(float delta)
const int FISH_PULL_CHANCE
const float INIT_LINE_STRETCH
class JsonUndergroundAreaTriggerData GetPosition
const float MAX_FISHING_TIME
FishingRod_Base_New ANIM_PHASE_OPENED
void AddAction(typename actionName)
override bool CanPutInCargo(EntityAI parent)
const string ANIM_PHASE_CLOSED
protected void GetPlayer()
float GetFishingEffectivityBonus()
ref Timer m_CyclerCatching
void Init()
Launched from 'DayZGame.DeferredInit' to make earlier access, use, and updates impossible (downside o...
const float TOO_MUCH_PULL