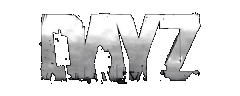 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file. 1 class TSelectableActionInfoArray
extends array<ref TSelectableActionInfo>
3 bool IsSameAs(TSelectableActionInfoArray other)
5 if (this.
Count() != other.Count())
10 for (
int i = 0; i <
Count(); ++i)
15 if (ai1.param2 != ai2.param2)
20 if (ai1.param3 != ai2.param3)
79 ac.ConstructActions(m_ActionsArray, m_ActionNameActionMap);
132 int AcknowledgmentID;
136 pCtx.Read(AcknowledgmentID);
141 pCtx.Read(AcknowledgmentID);
154 static ActionBase GetActionVariant(
typename actionName)
156 if (m_ActionNameActionMap)
158 ActionBase base_action = m_ActionNameActionMap.Get(actionName);
161 new_action.CreateConditionComponents();
162 new_action.SetID(base_action.GetID());
163 new_action.SetInput(base_action.GetInput());
172 if (m_ActionNameActionMap)
173 return m_ActionNameActionMap.Get(actionName);
180 return m_ActionsArray.Get(actionID);
223 if (
m_Player.GetWeaponManager().IsRunning())
271 Debug.ActionLog(
"Action data cleared ", this.
ToString() ,
"n/a",
"ActionEnd", m_CurrentActionData.m_Player.ToString());
int m_SelectedActionIndex
void OnContinuousCancel()
ActionReciveData GetReciveData()
protected ActionTarget m_SecondaryActionTarget
protected ItemBase m_TestedActionItem
protected void SetActionContext(ActionTarget target, ItemBase item)
bool m_TertiaryActionEnabled
Base Param Class with no parameters. Used as general purpose parameter overloaded with Param1 to Para...
void OnInstantAction(typename user_action_type, Param data=null)
float GetActionComponentProgress()
protected ref ActionData m_CurrentActionData
protected bool m_ActionsEnabled
protected bool m_ActionWantEndRequest
ActionBase GetRunningAction()
returns -1 when no action is running or RELOAD,MECHANISM, ....
class TSelectableActionInfoArray extends array< ref TSelectableActionInfo > m_Player
void Update(int pCurrentCommandID)
ActionBase GetContinuousAction()
ref TSelectableActionInfoArray m_SelectableActions
void SelectNextActionCategory()
void ActionManagerBase(PlayerBase player)
GetSelectedActionCategory()
protected ActionBase m_PrimaryAction
protected ActionBase m_SecondaryAction
Serialization general interface. Serializer API works with:
ItemBase GetRunningActionMainitem()
protected ActionTarget m_TestedActionTarget
TSelectableActionInfoArray GetSelectableActions()
protected void LocalInterrupt()
class ActionTargets ActionTarget
void OnSyncJuncture(int pJunctureID, ParamsReadContext pCtx)
bool OnInputUserDataProcess(int userDataType, ParamsReadContext ctx)
DayZPlayerConstants
defined in C++
protected int m_PendingActionAcknowledgmentID
bool ActionPossibilityCheck(int pCurrentCommandID)
void EnableActions(bool enable)
array< ref PlayerStatBase > Get()
ActionTarget FindActionTarget()
protected ItemBase m_PrimaryActionItem
Result for an object found in CGame.IsBoxCollidingGeometryProxy.
bool IsSelectableActionsChanged()
bool m_SelectableActionsHasChanged
void SelectPrevActionCategory()
protected ActionTarget m_PrimaryActionTarget
protected bool m_ActionInputWantEnd
int GetSelectedActionIndex()
ActionBase GetSingleUseAction()
void StartDeliveredAction()
protected ItemBase m_SecondaryActionItem
bool m_SecondaryActionEnabled
protected bool m_ActionsAvaibale
int GetActionState(ActionBase action)
void SelectFirstActionCategory()
bool m_PrimaryActionEnabled