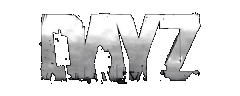 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
3 [
EditorAttribute(
"box",
"GameLib/Scripted",
"Render target",
"-0.25 -0.25 -0.25",
"0.25 0.25 0.25",
"255 0 0 255")]
4 class RenderTargetClass
9 RenderTargetClass RenderTargetSource;
11 class RenderTarget: GenericEntity
13 [
Attribute(
"0",
"slider",
"Camera index",
"0 31 1")]
15 [
Attribute(
"0",
"editbox",
"Position X <0, 1>")]
17 [
Attribute(
"0",
"editbox",
"Position Y <0, 1>")]
19 [
Attribute(
"1",
"editbox",
"Render target width <0, 1>")]
21 [
Attribute(
"1",
"editbox",
"Render target height <0, 1>")]
23 [
Attribute(
"-1",
"editbox",
"Sort index (the lesser the more important)")]
27 [
Attribute(
"0",
"combobox",
"Forcing creation of render target for camera #0 in Workbench",
"", {
ParamEnum(
"No",
"0"),
ParamEnum(
"Yes",
"1") } )]
30 ref RenderTargetWidget m_RenderWidget;
46 delete m_RenderWidget;
51 #ifdef WORKBENCH // Workbench is using its own renderer for main camera, it is not using render target widget.
52 if (!ForceCreation && CameraIndex == 0)
59 int posX = (
float)(screenW *
X);
60 int posY = (
float)(screenH *
Y);
61 int widthPix = (
float)(screenW * Width);
62 int heightPix = (
float)(screenH * Height);
65 m_RenderWidget.Show(m_Show);
74 m_RenderWidget.Show(m_Show);
proto native CGame GetGame()
proto void Sort(void param_array[], int num)
Sorts static array of integers(ascendically) / floats(ascendically) / strings(alphabetically)
proto void GetScreenSize(out int x, out int y)
protected override void EOnInit(IEntity other, int extra)
class InventoryGridController extends ScriptedWidgetEventHandler Init
void EditorAttribute(string style, string category, string description, vector sizeMin, vector sizeMax, string color, string color2="0 0 0 0", bool visible=true, bool insertable=true, bool dynamicBox=false)
proto native void SetFlags(ShapeFlags flags)
void ParamEnum(string key, string value, string desc="")
EntityEvent
Entity events for event-mask, or throwing event from code.
Super root of all classes in Enforce script.