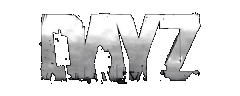 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
45 for (; i < newSize; i++)
48 if ( entity != NULL && entity.GetHierarchyRootPlayer() ==
_player )
56 for (i--; i >= newSize; i--)
63 _player.m_Hud.RefreshQuickbar(
true);
124 _player.m_Hud.RefreshQuickbar(
true);
134 _player.m_Hud.RefreshQuickbar(
true);
140 entity.GetInventory().GetCurrentInventoryLocation(loc);
143 return (entity && entity.GetHierarchyRootPlayer() ==
_player && parent.CanAssignAttachmentsToQuickbar() && entity.CanAssignToQuickbar());
184 if (!ctx.Read(param))
199 if (!ctx.Read(index))
203 if (!ctx.Read(force))
213 if ( entity == NULL )
230 int slotsCount =
_player.GetQuickBarBonus();
231 int attCount =
_player.GetInventory().AttachmentCount();
233 for (
int i = 0; i < attCount; ++i)
235 slotsCount +=
_player.GetInventory().GetAttachmentFromIndex(i).GetQuickBarBonus();
252 _player.m_Hud.RefreshQuickbar(
true);
272 _player.m_Hud.RefreshQuickbar(
true);
286 if (hic && hic.IsQuickBarSlot() == index + 1)
287 _player.OnQuickBarContinuousUseEnd(index + 1);
proto native CGame GetGame()
void QuickBarBase(PlayerBase player)
void OnSetEntityRequest(ParamsReadContext ctx)
Reaction on SetEntityShortcut from client.
protected void _SetEntity(EntityAI entity, int index, bool force=false)
protected void _SetEntityShortcut(EntityAI entity, int index, bool force=false)
void OnSetEntityNoSync(EntityAI entity, int index, bool force=false)
protected int m_slotsCount
bool CanAddAsShortcut(EntityAI entity)
protected void _RemoveEntity(int index)
int FindEntityIndex(EntityAI entity)
Serialization general interface. Serializer API works with:
void OnSetEntityRPC(ParamsReadContext ctx)
Reaction on Rpc from server for set inicial state for quickbar.
protected PlayerBase _player
void UpdateShotcutVisibility(int index)
class QuickBarItem m_aQuickbarEnts[MAX_QUICKBAR_SLOTS_COUNT]
void SetSize(int newSize)
EntityAI GetEntity(int index)
void SetShotcutEnable(int index, bool value)
bool OnInputUserDataProcess(int userDataType, ParamsReadContext ctx)
const int MAX_QUICKBAR_SLOTS_COUNT
void SetEntityShortcut(EntityAI entity, int index, bool force=false)
protected void CancelContinuousUse(int index)