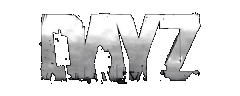 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
14 static const float UPDATE_TICK_RATE = 1;
55 int alarm_hand_in_mins = ConvertAlarmHand01ToMins12h(
m_AlarmTime01);
57 int pass, hour, minute;
58 GetGame().GetWorld().GetDate(pass, pass, pass, hour, minute);
60 int curr_time_in_minutes = ConvertTimeToMins12h(hour, minute);
61 int ring_in_mins = GetTimeDiffInMins12h(curr_time_in_minutes, alarm_hand_in_mins);
65 static int ConvertAlarmHand01ToMins12h(
float time01)
67 return Math.Lerp(0,12*60,time01);
70 static int ConvertAlarmHand01ToMins(
float time01,
int mins_max)
72 return Math.Lerp(0,mins_max,time01);
75 static float ConvertMins12hToAlarmHand01(
int mins)
77 return Math.InverseLerp(0,12*60,mins);
81 static int ConvertTimeToMins12h(
int hour,
int minute)
85 return hour * 60 + minute;
88 static int GetTimeDiffInMins12h(
int from_mins,
int to_mins)
90 if (to_mins > from_mins)
92 return to_mins - from_mins;
94 else if (to_mins < from_mins)
96 return ((12 * 60) - from_mins) + to_mins;
124 super.EEKilled(killer);
130 super.EEHitByRemote(damageType, source,
component, dmgZone, ammo, modelPos);
136 super.OnDamageDestroyed(oldLevel);
173 int pass, hour, minute;
174 GetGame().GetWorld().GetDate(pass, pass, pass, hour, minute);
175 int mins12h = ConvertTimeToMins12h(hour, minute) + in_mins;
176 float time01 = ConvertMins12hToAlarmHand01(mins12h);
190 m_TimerUpdate.Run(UPDATE_TICK_RATE ,
this,
"OnUpdate",
null,
true);
213 if (GetHierarchyParent())
218 parent.OnActivatedByItem(
this);
245 super.OnVariablesSynchronized();
322 SetAnimationPhaseNow(
"ClockAlarm", time01);
proto native CGame GetGame()
const float RINGING_DURATION_MAX
void SetAlarmInXMins(int in_mins)
string GetDestroyedSound()
void SetAlarmTimeServer(float time01)
protected void OnRingingStartServer()
protected void SetupTimerServer()
override void SetActions()
override void OnVariablesSynchronized()
ActionBreakLongWoodenStickCB ActionAttachExplosivesTrigger
protected void OnRingingStopClient()
override void EEHitByRemote(int damageType, EntityAI source, int component, string dmgZone, string ammo, vector modelPos)
override void EEKilled(Object killer)
EffectSound m_TurnOnSound
float GetRingingDurationMax()
class BoxCollidingParams component
ComponentInfo for BoxCollidingResult.
protected void MakeRingingStop()
protected void MakeRingingStart()
EffectSound m_WorkingSound
override void OnDamageDestroyed(int oldLevel)
Wrapper class for managing sound through SEffectManager.
void AddAction(typename actionName)
enum EAlarmClockState m_AlarmTime01
void Init()
Launched from 'DayZGame.DeferredInit' to make earlier access, use, and updates impossible (downside o...
EffectSound m_RingingSoundLoop
EffectSound m_DestoryedSound
protected void ActivateParent()
Manager class for managing Effect (EffectParticle, EffectSound)
protected void OnRingingStartClient()
protected void OnRingingStopServer()
protected void SetState(EAlarmClockState state)
protected int GetAlarmInMin()