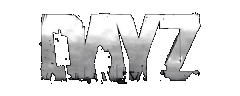 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
7 if (mask_array.Count() != 4)
9 mask_array = {0.0,0.0,0.0,0.0};
11 GetGame().AddPPMask(mask_array[0], mask_array[1], mask_array[2], mask_array[3]);
14 if (lens_array.Count() != 4)
16 lens_array = {0.0,0.0,0.0,0.0};
PostProcessEffectType
Post-process effect type.
proto native CGame GetGame()
Base Param Class with no parameters. Used as general purpose parameter overloaded with Param1 to Para...
GaussFilter - PostProcessEffectType.GaussFilter.
Glow - PostProcessEffectType.Glow.
base, not to be used directly, would lead to layering collisions!
PPOperators
PP operators, specify operation between subsequent layers.
DOF postprocess, does not directly use materials.