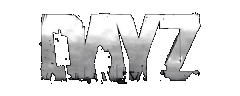 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
23 SetEnginePos(
"0 0.7 1.7");
47 super.OnEngineStart();
117 if (!super.CanReleaseAttachment(attachment))
122 string attType = attachment.GetType();
150 if ( !super.CanDisplayAttachmentCategory(category_name))
155 category_name.ToLower();
156 if (category_name.Contains(
"engine"))
169 if ( !super.CanDisplayCargo() )
182 Class.CastTo( carDoor, FindAttachmentBySlotName( slotType ) );
190 case "Offroad_02_Door_1_1":
193 case "Offroad_02_Door_2_1":
196 case "Offroad_02_Door_1_2":
199 case "Offroad_02_Door_2_2":
202 case "Offroad_02_Hood":
205 case "Offroad_02_Trunk":
254 return "doors_driver";
257 return "doors_codriver";
260 return "doors_cargo1";
263 return "doors_cargo2";
267 return super.GetDoorSelectionNameFromSeatPos(posIdx);
275 return "Offroad_02_Door_1_1";
277 return "Offroad_02_Door_2_1";
279 return "Offroad_02_Door_1_2";
281 return "Offroad_02_Door_2_2";
284 return super.GetDoorInvSlotNameFromSeatPos(posIdx);
313 return Math.Clamp(newValue, 0, 1);
317 return super.OnSound(ctrl, oldValue);
325 return "DoorsDriver";
326 case "doors_codriver":
327 return "DoorsCoDriver";
329 return "DoorsCargo1";
331 return "DoorsCargo2";
346 return nextSeat == 1;
348 return nextSeat == 0;
350 return nextSeat == 3;
352 return nextSeat == 2;
360 switch (pCurrentSeat)
363 return pDoorsSelection ==
"DoorsDriver";
365 return pDoorsSelection ==
"DoorsCoDriver";
367 return pDoorsSelection ==
"DoorsCargo1";
369 return pDoorsSelection ==
"DoorsCargo2";
396 GetInventory().CreateInInventory(
"Offroad_02_Wheel");
397 GetInventory().CreateInInventory(
"Offroad_02_Wheel");
398 GetInventory().CreateInInventory(
"Offroad_02_Wheel");
399 GetInventory().CreateInInventory(
"Offroad_02_Wheel");
401 GetInventory().CreateInInventory(
"Offroad_02_Door_1_1");
402 GetInventory().CreateInInventory(
"Offroad_02_Door_1_2");
403 GetInventory().CreateInInventory(
"Offroad_02_Door_2_1");
404 GetInventory().CreateInInventory(
"Offroad_02_Door_2_2");
405 GetInventory().CreateInInventory(
"Offroad_02_Hood");
406 GetInventory().CreateInInventory(
"Offroad_02_Trunk");
409 GetInventory().CreateInInventory(
"Offroad_02_Wheel");
410 GetInventory().CreateInInventory(
"Offroad_02_Wheel");
proto native CGame GetGame()
protected ref UniversalTemperatureSource m_UTSource
override float OnSound(CarSoundCtrl ctrl, float oldValue)
string m_CarDoorCloseSound
override void OnEngineStart()
Gets called everytime the engine starts.
protected CarRearLightBase CreateRearLight()
protected void SpawnAdditionalItems()
override bool CrewCanGetThrough(int posIdx)
string m_CarHornLongSoundName
protected CarLightBase CreateFrontLight()
override bool CanReleaseAttachment(EntityAI attachment)
string GetAnimSourceFromSelection(string selection)
override int GetAnimInstance()
string m_CarHornShortSoundName
original Timer deletes m_params which is unwanted
int GetCarDoorsState(string slotType)
string m_CarDoorOpenSound
CarSoundCtrl
Car's sound controller list. (native, do not change or extend)
override float GetTransportCameraDistance()
protected ref UniversalTemperatureSourceSettings m_UTSSettings
class Hatchback_02_Blue extends Hatchback_02 OnDebugSpawn
protected bool CanManipulateSpareWheel(string slotSelectionName)
DayZPlayerConstants
defined in C++
string GetDoorSelectionNameFromSeatPos(int posIdx)
bool IsVitalTruckBattery()
protected void SpawnUniversalParts()
protected ref UniversalTemperatureSourceLambdaEngine m_UTSLEngine
override void EOnPostSimulate(IEntity other, float timeSlice)
string GetDoorInvSlotNameFromSeatPos(int posIdx)
override bool CanReachDoorsFromSeat(string pDoorsSelection, int pCurrentSeat)
string m_EngineStartBattery
protected void FillUpCarFluids()
CarDoorState TranslateAnimationPhaseToCarDoorState(string animation)
override int GetSeatAnimationType(int posIdx)
override bool CanDisplayCargo()
override bool CanReachSeatFromSeat(int currentSeat, int nextSeat)
override bool CanDisplayAttachmentCategory(string category_name)
Super root of all classes in Enforce script.
string m_EngineStartOK
Sounds.
override void OnEngineStop()
Gets called everytime the engine stops.