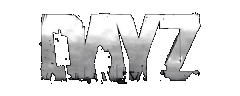 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
128 m_EntityIsTakeable =
true;
134 m_SoundSetOneTime =
"";
135 m_SoundSetPersistent=
"";
137 m_ReplaceWithEntity =
"";
138 m_KeepHealthOnReplace =
true;
139 m_ReplaceDelay = 1000;
141 m_HasExplosionDamage =
true;
147 override void OnEntityDestroyedOneTimeClient(
EntityAI entity,
int oldLevel,
string zone)
151 override void OnEntityDestroyedOneTimeServer(
EntityAI entity,
int oldLevel,
string zone)
155 override void OnEntityDestroyedPersistentClient(
EntityAI entity,
string zone)
159 override void OnEntityDestroyedPersistentServer(
EntityAI entity,
string zone)
163 override void OnExplosionEffects(
Object source,
Object directHit,
int componentIndex,
string surface,
vector pos,
vector surfNormal,
float energyFactor,
float explosionFactor,
bool isWater,
string ammoType)
172 m_EntityIsTakeable =
true;
178 m_SoundSetOneTime =
"AlarmClock_Destroyed_SoundSet";
179 m_SoundSetPersistent=
"";
181 m_ReplaceWithEntity =
"";
182 m_KeepHealthOnReplace =
true;
183 m_ReplaceDelay = 1000;
185 m_HasExplosionDamage =
false;
207 override void OnExplosionEffects(
Object source,
Object directHit,
int componentIndex,
string surface,
vector pos,
vector surfNormal,
float energyFactor,
float explosionFactor,
bool isWater,
string ammoType)
216 m_EntityIsTakeable =
false;
221 m_SoundSetOneTime =
"";
222 m_SoundSetPersistent=
"";
224 m_ReplaceWithEntity =
"";
225 m_KeepHealthOnReplace =
false;
228 m_HasExplosionDamage =
false;
234 override void OnEntityDestroyedOneTimeClient(
EntityAI entity,
int oldLevel,
string zone)
238 override void OnEntityDestroyedOneTimeServer(
EntityAI entity,
int oldLevel,
string zone)
242 override void OnEntityDestroyedPersistentClient(
EntityAI entity,
string zone)
246 override void OnEntityDestroyedPersistentServer(
EntityAI entity,
string zone)
250 override void OnExplosionEffects(
Object source,
Object directHit,
int componentIndex,
string surface,
vector pos,
vector surfNormal,
float energyFactor,
float explosionFactor,
bool isWater,
string ammoType)
override void OnEntityDestroyedPersistentClient(EntityAI entity, string zone)
override void OnEntityDestroyedPersistentServer(EntityAI entity, string zone)
override void OnEntityDestroyedOneTimeClient(EntityAI entity, int oldLevel, string zone)
override void OnEntityDestroyedOneTimeServer(EntityAI entity, int oldLevel, string zone)
DamageType
exposed from C++ (do not change)
DestructionEffectGasCanister DestructionEffectBase Init()
Launched from 'DayZGame.DeferredInit' to make earlier access, use, and updates impossible (downside o...
override void OnExplosionEffects(Object source, Object directHit, int componentIndex, string surface, vector pos, vector surfNormal, float energyFactor, float explosionFactor, bool isWater, string ammoType)