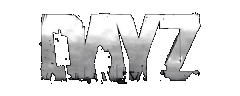 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
33 super.OnEngineStart();
93 Class.CastTo( carDoor, FindAttachmentBySlotName( slotType ) );
99 case "Van_01_Door_1_1":
100 if ( GetAnimationPhase(
"DoorsDriver") > 0.5 )
107 case "Van_01_Door_2_1":
108 if ( GetAnimationPhase(
"DoorsCoDriver") > 0.5 )
115 case "Van_01_Door_2_2":
116 if ( GetAnimationPhase(
"DoorsCargo1") > 0.5 )
123 case "Van_01_Trunk_1":
124 if ( GetAnimationPhase(
"DoorsCargo3") > 0.5 )
130 case "Van_01_Trunk_2":
131 if ( GetAnimationPhase(
"DoorsCargo2") > 0.5 )
227 return "DoorsDriver";
228 case "doors_codriver":
229 return "DoorsCoDriver";
231 return "DoorsCargo1";
233 return "DoorsCargo2";
235 return "DoorsCargo3";
249 return "seat_con_1_1";
251 case "seat_codriver":
252 return "seat_con_2_1";
257 return "seat_con_1_2";
279 switch( currentSeat )
305 switch( pCurrentSeat )
308 if (pDoorsSelection ==
"DoorsDriver")
314 if (pDoorsSelection ==
"DoorsCoDriver")
320 if (pDoorsSelection ==
"DoorsCargo1")
326 if (pDoorsSelection ==
"DoorsCargo2")
proto native CGame GetGame()
protected ref UniversalTemperatureSource m_UTSource
override float OnSound(CarSoundCtrl ctrl, float oldValue)
string GetDoorConditionPointFromSelection(string selection)
override void OnEngineStart()
Gets called everytime the engine starts.
override bool CrewCanGetThrough(int posIdx)
string GetAnimSourceFromSelection(string selection)
override int GetAnimInstance()
original Timer deletes m_params which is unwanted
int GetCarDoorsState(string slotType)
CarSoundCtrl
Car's sound controller list. (native, do not change or extend)
protected ref UniversalTemperatureSourceSettings m_UTSSettings
DayZPlayerConstants
defined in C++
bool IsVitalTruckBattery()
protected ref UniversalTemperatureSourceLambdaEngine m_UTSLEngine
override void EOnPostSimulate(IEntity other, float timeSlice)
override bool CanReachDoorsFromSeat(string pDoorsSelection, int pCurrentSeat)
override int GetSeatAnimationType(int posIdx)
override bool CanReachSeatFromSeat(int currentSeat, int nextSeat)
Super root of all classes in Enforce script.
override void OnEngineStop()
Gets called everytime the engine stops.