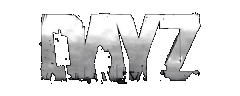 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
34 super.OnEngineStart();
87 case "Truck_02_Door_1_1":
88 Class.CastTo( carDoor, FindAttachmentBySlotName( slotType ) );
91 if ( GetAnimationPhase(
"DoorsDriver") > 0.5 )
104 case "Truck_02_Door_2_1":
105 Class.CastTo( carDoor, FindAttachmentBySlotName( slotType ) );
108 if ( GetAnimationPhase(
"DoorsCoDriver") > 0.5 )
185 return "DoorsDriver";
186 case "doors_codriver":
187 return "DoorsCoDriver";
192 case "wheelsideplate1":
193 return "WheelSidePlate1";
194 case "wheelsideplate2":
195 return "WheelSidePlate2";
228 switch( currentSeat )
246 switch( pCurrentSeat )
249 if (pDoorsSelection ==
"DoorsDriver")
256 if (pDoorsSelection ==
"DoorsCoDriver")
268 switch (pDoorSelection)
273 case "DoorsCoDriver":
284 if (
Class.CastTo(entity,
this) )
286 entity.GetInventory().CreateInInventory(
"Truck_02_Wheel" );
287 entity.GetInventory().CreateInInventory(
"Truck_02_Wheel" );
288 entity.GetInventory().CreateInInventory(
"Truck_02_Wheel" );
289 entity.GetInventory().CreateInInventory(
"Truck_02_Wheel" );
291 entity.GetInventory().CreateInInventory(
"TruckBattery" );
292 entity.GetInventory().CreateInInventory(
"SparkPlug" );
294 entity.GetInventory().CreateInInventory(
"Truck_02_Door_1_1" );
295 entity.GetInventory().CreateInInventory(
"Truck_02_Door_2_1" );
297 entity.GetInventory().CreateInInventory(
"HeadlightH7" );
298 entity.GetInventory().CreateInInventory(
"HeadlightH7" );
proto native CGame GetGame()
protected ref UniversalTemperatureSource m_UTSource
override float OnSound(CarSoundCtrl ctrl, float oldValue)
override void OnEngineStart()
Gets called everytime the engine starts.
override bool CrewCanGetThrough(int posIdx)
string GetAnimSourceFromSelection(string selection)
override int GetAnimInstance()
original Timer deletes m_params which is unwanted
int GetCarDoorsState(string slotType)
CarSoundCtrl
Car's sound controller list. (native, do not change or extend)
protected ref UniversalTemperatureSourceSettings m_UTSSettings
class Hatchback_02_Blue extends Hatchback_02 OnDebugSpawn
CarFluid
Type of vehicle's fluid. (native, do not change or extend)
override int GetSeatIndexFromDoor(string pDoorSelection)
DayZPlayerConstants
defined in C++
protected ref UniversalTemperatureSourceLambdaEngine m_UTSLEngine
override void EOnPostSimulate(IEntity other, float timeSlice)
override bool CanReachDoorsFromSeat(string pDoorsSelection, int pCurrentSeat)
override int GetSeatAnimationType(int posIdx)
override bool CanReachSeatFromSeat(int currentSeat, int nextSeat)
Super root of all classes in Enforce script.
protected vector m_enginePtcPos
override void OnEngineStop()
Gets called everytime the engine stops.