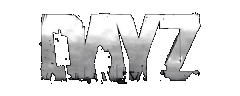 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
3 private float m_EffectPhase = 0;
4 private float m_Magnitude = 0;
5 private float m_MinMagnitude = 0.3;
6 private float m_MaxMagnitude = 0.3;
7 private float m_Frequency = 5;
8 private float m_Stamina01;
18 override protected void OnUpdate(
float delta)
20 super.OnUpdate(delta);
25 m_EffectPhase += delta * m_Frequency / (m_Stamina01 + 1);
27 float currentVal = (
Math.Sin(m_EffectPhase) +1)/2;
28 currentVal =
Easing.EaseInExpo(currentVal);
29 currentVal *= currentVal * m_MaxMagnitude;
30 currentVal += m_MinMagnitude;
31 m_Magnitude =
Math.Lerp(m_Magnitude, currentVal, delta * m_Frequency);
37 void SetStamina01(
float stamina01)
39 m_Stamina01 = stamina01;
PostProcessEffectType
Post-process effect type.
Base Param Class with no parameters. Used as general purpose parameter overloaded with Param1 to Para...
GodRays - PostProcessEffectType.GodRays.
Input value between 0 and 1, returns value adjusted by easing, no automatic clamping of input(do your...
Glow - PostProcessEffectType.Glow.
base, not to be used directly, would lead to layering collisions!
PPOperators
PP operators, specify operation between subsequent layers.
enum EAchievementError OnStart