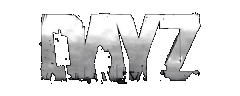 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
31 protected int m_Value;
34 protected ref
Timer m_CooldownTimer;
46 m_PresenceNotifierNotifierEvents.Insert(pEventType, pnne);
53 pnne = m_PresenceNotifierNotifierEvents.Get(pEventType);
55 if (m_CooldownTimer.IsRunning())
57 m_CooldownTimer.Stop();
61 m_CooldownTimer.Run(pnne.GetTimerLength(),
this,
"ResetEvent",
null);
69 protected void ResetEvent()
129 DbgUI.BeginCleanupScope();
136 string visualAlertLevel;
137 string noiseAlertLevel;
142 DbgUI.Text(
"Visual: ");
149 DbgUI.Text(
"Noises: ");
156 DbgUI.Text(
"Result: ");
157 visualAlertLevel =
"";
160 visualAlertLevel +=
"!";
165 noiseAlertLevel =
"";
168 noiseAlertLevel +=
"!";
178 DbgUI.Text(
"Command ID: " + hms.m_CommandTypeId);
179 DbgUI.Text(
"Stance: " + hms.m_iStanceIdx);
180 DbgUI.Text(
"Movement: " + hms.m_iMovement);
184 DbgUI.EndCleanupScope();
207 float visualMean = 0;
233 float speedCoef = 1.0;
255 float stanceCoef = 1.0;
258 switch (hms.m_iStanceIdx)
const float SURFACE_LVL1_THRESHOLD
void Init(PlayerBase player)
void ProcessEvent(EPresenceNotifierNoiseEventType pEventType)
processing of external one-time events (land, fire, etc.)
const int NOISE_LEVEL_MAX
protected ref PresenceNotifierNoiseEvents m_PresenceNotifierNoiseEvents
void PluginPresenceNotifier()
protected float GetMovementStanceVisualCoef()
EPresenceNotifierNoiseEventType
void PresenceNotifierNoiseEvent(float pValue, float pLength)
int GetVisualPresence()
returns actual visibility presence of player
protected int GetExternalNoiseEventsComponent()
DEPRECATED.
const int SURFACE_NOISE_LVL2
enum EPresenceNotifierNoiseEventType m_TimerLength
protected PlayerBase m_pPlayer
protected int GetMovementSpeedNoiseComponent()
void ~PluginPresenceNotifier()
protected int ProcessNoiseComponents()
int GetNoisePresence()
returns actual noise presence of player
protected int ProcessVisualComponents()
const int SURFACE_NOISE_LVL1
void EnableDebug(bool pEnabled)
DayZPlayerConstants
defined in C++
class PresenceNotifierNoiseEvents windowPosX
dbgUI settings
protected void ShowCoefsDbg(bool pEnabled)
const int LAND_NOISE_LVL1
land noise
protected int GetSurfaceNoiseComponent()
DEPRECATED.
const int NOISE_LEVEL_MIN
noise limits
const float SURFACE_LVL2_THRESHOLD
const int LAND_NOISE_LVL2
const int SURFACE_NOISE_LVL0
noise component from surfaces
protected float GetMovementSpeedVisualCoef()
Visibility.
protected int GetBootsNoiseComponent()