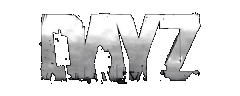 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
3 protected SliderWidget m_Slider;
12 override void Enable()
19 override void Disable()
31 m_ParentClass.OnFocus(
m_Root.GetParent(), -1, m_SelectorType);
32 #ifdef PLATFORM_WINDOWS
33 m_ParentClass.OnMouseEnter(
m_Root.GetParent().GetParent(),
x,
y);
45 m_ParentClass.OnFocus(
null,
x,
y);
46 #ifdef PLATFORM_WINDOWS
47 m_ParentClass.OnMouseLeave(
m_Root.GetParent().GetParent(), enterW,
x,
y);
59 if (button ==
MouseState.LEFT && w == m_Slider)
79 return (w ==
m_Parent || w == m_Slider);
86 #ifdef PLATFORM_CONSOLE
110 float NormalizeInput(
float value)
116 void SetStep(
float step)
118 m_Slider.SetStep(step);
121 void SetValue(
float value,
bool update =
true)
123 m_Slider.SetCurrent(NormalizeInput(value));
134 void SetMax(
float max)
147 m_Slider.SetColor(
ARGB(255, 200, 0, 0));
150 super.ColorHighlight(w);
160 m_Slider.SetColor(
ARGB(140, 255, 255, 255));
163 super.ColorNormal(w);
This Option Selector handles a Slider Marker, which basically has 2 sliders One slider is for selecti...
protected Widget m_Parent
override bool OnMouseLeave(Widget w, Widget enterW, int x, int y)
void ColorNormal(Widget w)
void ColorHighlight(Widget w)
override bool OnFocusLost(Widget w, int x, int y)
string GetValue(int pIndex)
override bool OnFocus(Widget w, int x, int y)
bool OnChange(Widget w, int x, int y, bool finished)
override bool OnMouseEnter(Widget w, int x, int y)
int ARGB(int a, int r, int g, int b)
override void SetValue(int value, bool fire_event=true)
bool IsFocusable(Widget w)