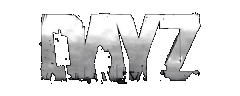 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
14 protected void OnErrorThrown(
int errorCode, owned
string additionalInfo =
"")
22 string GetClientMessage(
int errorCode,
string additionalInfo =
"")
24 return GetSimpleMessage(errorCode, additionalInfo);
28 string GetLastClientMessage(
int errorCode)
30 return GetSimpleMessage(errorCode);
34 string GetServerMessage(
int errorCode,
string additionalInfo =
"")
36 return GetSimpleMessage(errorCode, additionalInfo);
40 string GetLastServerMessage(
int errorCode)
42 return GetSimpleMessage(errorCode);
46 string GetSimpleMessage(
int errorCode,
string additionalInfo =
"")
48 return string.Format(
"[%1]: %2",
ErrorModuleHandler.GetErrorHex(errorCode), additionalInfo);
119 Error(
string.Format(
"[EHM] Could not find any properties for error %1(%2) in %3", errorCode,
ErrorModuleHandler.GetErrorHex(errorCode),
this));
136 return properties.GetClientMessage(additionalInfo);
140 return additionalInfo;
173 return properties.GetServerMessage(additionalInfo);
177 return additionalInfo;
206 protected override void OnErrorThrown(
int errorCode, owned
string additionalInfo =
"")
208 super.OnErrorThrown(errorCode, additionalInfo);
217 properties.HandleError(errorCode, additionalInfo);
224 properties.HandleError(errorCode, additionalInfo);
233 void InsertDialogueErrorProperties(
int code,
string message,
int dialogButtonType = DBT_OK,
int defaultButton = DBB_OK,
int dialogMeaningType = DMT_EXCLAMATION,
bool displayAdditionalInfo =
true)
235 m_ErrorDataMap.Insert(code,
DialogueErrorProperties(
string.Format(
"%1%2",
m_Prefix, message), message,
m_Header,
m_UIHandler, dialogButtonType, defaultButton, dialogMeaningType, displayAdditionalInfo));
239 void InsertHeaderDialogueErrorProperties(
int code,
string message,
string header,
int dialogButtonType = DBT_OK,
int defaultButton = DBB_OK,
int dialogMeaningType = DMT_EXCLAMATION,
bool displayAdditionalInfo =
true)
241 m_ErrorDataMap.Insert(code,
DialogueErrorProperties(
string.Format(
"%1%2",
m_Prefix, message), message, header,
m_UIHandler, dialogButtonType, defaultButton, dialogMeaningType, displayAdditionalInfo));
245 void InsertPrefixDialogueErrorProperties(
int code,
string message,
string prefix,
int dialogButtonType = DBT_OK,
int defaultButton = DBB_OK,
int dialogMeaningType = DMT_EXCLAMATION,
bool displayAdditionalInfo =
true)
247 m_ErrorDataMap.Insert(code,
DialogueErrorProperties(
string.Format(
"%1%2", prefix, message), message,
m_Header,
m_UIHandler, dialogButtonType, defaultButton, dialogMeaningType, displayAdditionalInfo));
253 m_ErrorDataMap.Insert(code,
DialogueErrorProperties(
string.Format(
"%1%2%3",
m_Prefix, prefix, message), message,
m_Header,
m_UIHandler, dialogButtonType, defaultButton, dialogMeaningType, displayAdditionalInfo));
257 void InsertExtendedPrefixSplitDialogueErrorProperties(
int code,
string message,
string prefix,
string serverMessage,
int dialogButtonType = DBT_OK,
int defaultButton = DBB_OK,
int dialogMeaningType = DMT_EXCLAMATION,
bool displayAdditionalInfo =
true)
259 m_ErrorDataMap.Insert(code,
DialogueErrorProperties(
string.Format(
"%1%2%3",
m_Prefix, prefix, message), serverMessage,
m_Header,
m_UIHandler, dialogButtonType, defaultButton, dialogMeaningType, displayAdditionalInfo));
263 void InsertSplitDialogueErrorProperties(
int code,
string message,
string serverMessage,
int dialogButtonType = DBT_OK,
int defaultButton = DBB_OK,
int dialogMeaningType = DMT_EXCLAMATION,
bool displayAdditionalInfo =
true)
265 m_ErrorDataMap.Insert(code,
DialogueErrorProperties(
string.Format(
"%1%2",
m_Prefix, message), serverMessage,
m_Header,
m_UIHandler, dialogButtonType, defaultButton, dialogMeaningType, displayAdditionalInfo));
override string GetLastServerMessage(int errorCode)
Fetches the Server message for the error code, attempting to retrieve the data from the latest.
void Error(string err)
Messagebox with error message.
override string GetLastClientMessage(int errorCode)
Fetches the Client message for the error code, attempting to retrieve the data from the latest.
Base Param Class with no parameters. Used as general purpose parameter overloaded with Param1 to Para...
void InsertHeaderDialogueErrorProperties(int code, string message, string header, int dialogButtonType=DBT_OK, int defaultButton=DBB_OK, int dialogMeaningType=DMT_EXCLAMATION, bool displayAdditionalInfo=true)
Insert an error with Dialogue as handling with custom header.
protected override void OnErrorThrown(int errorCode, owned string additionalInfo="")
Event that gets triggered when an error of the owned category is thrown. Do not call directly!...
proto void Print(void var)
Prints content of variable to console/log.
Class which holds the properties and handling of an error.
override string GetServerMessage(int errorCode, string additionalInfo="")
Fetches the Server message for the error code.
void InitOptionalVariables()
Function which gets called before FillErrorDataMap, designed to set Optional Variales before ErrorPro...
void InsertPrefixDialogueErrorProperties(int code, string message, string prefix, int dialogButtonType=DBT_OK, int defaultButton=DBB_OK, int dialogMeaningType=DMT_EXCLAMATION, bool displayAdditionalInfo=true)
Insert an error with Dialogue as handling with custom prefix.
void InsertErrorProperties(int code, string message="")
Insert an error with no handling.
ErrorCategory
ErrorCategory - To decide what ErrorHandlerModule needs to be called and easily identify where it cam...
void DialogueErrorProperties(string message, string serverMessage, string header, UIScriptedMenu handler=null, int dialogButtonType=DBT_OK, int defaultButton=DBB_OK, int dialogMeaningType=DMT_EXCLAMATION, bool displayAdditionalInfo=true)
void InsertDialogueErrorProperties(int code, string message, int dialogButtonType=DBT_OK, int defaultButton=DBB_OK, int dialogMeaningType=DMT_EXCLAMATION, bool displayAdditionalInfo=true)
Insert an error with Dialogue as handling, using the Optional Variables.
void InsertExtendedPrefixSplitDialogueErrorProperties(int code, string message, string prefix, string serverMessage, int dialogButtonType=DBT_OK, int defaultButton=DBB_OK, int dialogMeaningType=DMT_EXCLAMATION, bool displayAdditionalInfo=true)
Insert an error with Dialogue as handling with extended prefix and separate server message.
void ~ErrorHandlerModuleScript()
void FillErrorDataMap()
Function to fill up m_ErrorDataMap, gets called in the Constructor.
protected ref map< int, ref ErrorProperties > m_ErrorDataMap
Map containing the codes that exist for the ErrorHandlerModule The code links to ErrorProperties This...
protected int m_LastErrorThrown
Holds the last thrown error in this module, defaults to 0.
void InsertSplitDialogueErrorProperties(int code, string message, string serverMessage, int dialogButtonType=DBT_OK, int defaultButton=DBB_OK, int dialogMeaningType=DMT_EXCLAMATION, bool displayAdditionalInfo=true)
Insert an error with Dialogue as handling with separate server message.
class ErrorHandlerModule m_Header
This is where to input logic and extend functionality of ErrorHandlerModule.
protected string m_LastAdditionalInfo
Holds the last additional info passed in.
protected string m_Prefix
Optional: Prefix (e.g. Fixed text at the start of the messages in the module)
void InsertExtendedPrefixDialogueErrorProperties(int code, string message, string prefix, int dialogButtonType=DBT_OK, int defaultButton=DBB_OK, int dialogMeaningType=DMT_EXCLAMATION, bool displayAdditionalInfo=true)
Insert an error with Dialogue as handling with extended prefix.
ErrorProperties GetProperties(int errorCode)
Fetches the ErrorProperties for the error code.
Definition and API of an ErrorHandlerModule - Do not insert any logic here! (as this class is not mod...
void ErrorHandlerModuleScript()
Constructor, by default calls the function that will fill the ErrorDataMap.
override string GetClientMessage(int errorCode, string additionalInfo="")
Fetches the Client message for the error code.
The error handler itself, for managing and distributing errors to modules Manages the ErrorHandlerMod...
protected ref UIScriptedMenu m_UIHandler
Optional: The UI the handler might generally use