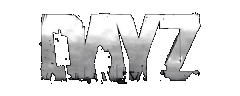 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
34 proto
vobject CreateXOB(
int nsurfaces,
int nverts[],
int numindices[],
string materials[]);
@ GRAVITY_SCALE_RND
Float R/W.
@ LIFETIME_BY_ANIM
Bool R/W.
proto native int ClearAnimMask(notnull IEntity ent, int mask)
proto native int IsAnimSlotPlaying(notnull IEntity ent, int mask)
@ CURRENT_TIME
current efector's time. Float R/W
proto native void SetAnimMask(notnull IEntity ent, int mask)
@ REPEAT
should efector repeate after time up? Bool R/W
class BoneMask NOANIMHOOKS
Animhooks will not be called.
proto native void SetAnimSlot(notnull IEntity ent, int slot, vobject anim, float blendin, float blendout, BoneMask mask, int fps, AnimFlags flags)
proto native void ChangeAnimSlotFPS(notnull IEntity ent, int slot, int fps)
proto native int GetParticleCount(notnull IEntity ent)
proto int GetObjectMaterials(vobject object, string materials[])
@ RANDOM_ROT
rotate in random direction. Bool R/W
proto native bool SetBoneMatrix(notnull IEntity ent, int bone, vector mat[4])
proto native bool GetBoneMatrix(notnull IEntity ent, int bone, vector mat[4])
proto native void ReleaseObject(vobject object, int flag=0)
proto int GetParticleEmitors(notnull IEntity ent, out string emitors[], int max)
proto native void SetBone(notnull IEntity ent, int bone, vector angles, vector trans, float scale)
proto string vtoa(vobject vobj)
Returns name of visual object.
@ EFFECT_TIME
efector's total time. Float R/W
proto int GetParticleEmitorCount(notnull IEntity ent)
proto vobject CreateXOB(int nsurfaces, int nverts[], int numindices[], string materials[])
Dynamic MeshObject.
class BoneMask NOANIMEND
EntityEvent.ANIMEND will not be called.
proto native int GetNumAnimFrames(vobject anim)
Returns number of frames, if the object is animation.
@ BIRTH_RATE_RND
Float R/W.
bool ParticleHasActive(IEntity ent)
proto void GetParticleParmOriginal(notnull IEntity ent, int emitor, EmitorParam parameter, out void value)
proto native void ChangeAnimSlotMask(notnull IEntity ent, int slot, float blendin, BoneMask mask)
proto native void RestartParticle(notnull IEntity ent)
@ ACTIVE_PARTICLES
number of active particles. Int R
class BoneMask ONCE
animation is played only once and then if freezes at the last frame. EntityEvent.ANIMEND is called
class BoneMask USER
animation waits on the first frame. Frame is set by calling SetFrame()
proto native bool SetMorphState(notnull IEntity ent, string morph, float value)
@ AIR_RESISTANCE
Float R/W.
PlayerSpawnPresetDiscreteItemSetSlotData name
one set for cargo
proto native vobject GetObject(string name)
Loads object from data, or gets it from cache. Object must be released when not used.
@ GRAVITY_SCALE
Float R/W.
proto void UpdateVertsEx(notnull IEntity ent, int surf, vector verts[], float uv[])
int ParticleGetCount(IEntity ent)
proto void SetParticleParm(notnull IEntity ent, int emitor, EmitorParam parameter, void value)
proto native void SetBoneGlobal(notnull IEntity ent, int bone, vector mat[4])
proto void GetParticleParm(notnull IEntity ent, int emitor, EmitorParam parameter, out void value)
@ AIR_RESISTANCE_RND
Float R/W.
proto native bool GetBoneLocalMatrix(notnull IEntity ent, int bone, vector mat[4])
@ RANDOM_ANGLE
begin with random rotation. Bool R/W
class BoneMask RESET
forces animation to start from begining (including blending)
proto native void ResetParticlePosition(notnull IEntity ent)
proto native void SetAnimFrame(notnull IEntity ent, int slot, float frame)
proto bool HasActiveParticle(notnull IEntity ent)
proto void UpdateIndices(vobject obj, int surf, int indices[])