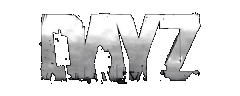 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
8 private const string m_RootPath =
"Gui/layouts/new_ui/hints/in_game_hints.layout";
9 private const string m_DataPath =
"Scripts/data/hints.json";
44 Print(
"ERROR: UiHintPanel - Could not create the hint panel. The data are missing!");
proto native CGame GetGame()
protected void StartSlideshow()
protected string m_RootPath
protected void SetHintDescription()
proto void Print(void var)
Prints content of variable to console/log.
protected TextWidget m_UiHeadlineLabel
void UiHintPanel(Widget parent_widget)
protected ImageWidget m_UiHintImage
protected Widget m_SpacerFrame
protected TextWidget m_UiPageingLabel
protected void ShowNextPage()
override bool OnMouseLeave(Widget w, Widget enterW, int x, int y)
protected void LoadContentList()
protected void RestartSlideShow()
protected ref array< ref HintPage > m_ContentList
protected int m_SlideShowDelay
protected ButtonWidget m_UiRightButton
protected void SlideshowThread()
protected void PopulateLayout()
protected void StopSlideShow()
const protected string m_DataPath
protected void SetHintPaging()
protected void SetHintHeadline()
protected void RandomizePageIndex()
protected void SetHintImage()
protected RichTextWidget m_UiDescLabel
override bool OnClick(Widget w, int x, int y, int button)
protected Widget m_RootFrame
override bool OnMouseEnter(Widget w, int x, int y)
protected void ShowPreviousPage()
protected ButtonWidget m_UiLeftButton
protected void BuildLayout(Widget parent_widget)
protected int m_PageIndex