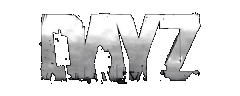 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
11 static int m_PrimaryDropsAmount = -1;
12 static int m_SecondaryDropsAmount = -1;
13 static float m_ToolDamage = -1.0;
14 static float m_CycleTimeOverride = -1.0;
15 static string m_PrimaryOutput =
"";
16 static string m_SecondaryOutput =
"";
17 static string m_BarkType =
"";
48 return ConfigGetBool(
"isCuttable");
53 return ConfigGetInt(
"primaryDropsAmount");
58 return ConfigGetInt(
"secondaryDropsAmount");
63 return ConfigGetFloat(
"toolDamage");
68 return ConfigGetFloat(
"cycleTimeOverride");
73 return ConfigGetString(
"primaryOutput");
78 return ConfigGetString(
"secondaryOutput");
83 return ConfigGetString(
"barkType");
92 if ( IsTree() && item && ( item.KindOf(
"Knife") || item.IsInherited(Screwdriver) ) )
103 if ( item && ( item.KindOf(
"Knife") || item.IsInherited(Screwdriver) ) )
107 else if ( item && item.KindOf(
"Axe") )
150 if ( IsTree() && item && ( item.KindOf(
"Knife") || item.IsInherited(Screwdriver) ) &&
GetBarkType() !=
"" )
179 if ( item && item.KindOf(
"Knife") )
183 else if ( item && item.KindOf(
"Axe") )
194 if ( item && item.KindOf(
"Knife") )
198 else if ( item && item.KindOf(
"Axe") )
override bool CanBeActionTarget()
int GetAmountOfDrops(ItemBase item)
void GetMaterialAndQuantityMap(ItemBase item, out map< string, int > output_map)
protected bool IsDamageDestroyed(ActionTarget target)
float GetDamageToMiningItemEachDrop(ItemBase item)
enum EHarvestType m_IsCuttable
override bool IsWoodBase()
int GetAmountOfDropsEx(ItemBase item, EHarvestType type)
void GetMaterialAndQuantityMapEx(ItemBase item, out map< string, int > output_map, EHarvestType type)
int GetSecondaryDropsAmount()
float GetCycleTimeOverride()
string GetSecondaryOutput()
override bool IsCuttable()
string GetPrimaryOutput()
float GetDamageToMiningItemEachDropEx(ItemBase item, EHarvestType type)
int GetPrimaryDropsAmount()