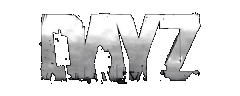 |
Dayz Explorer
1.24.157551 (v105080)
Dayz Code Explorer by Zeroy
|
Go to the documentation of this file.
22 void SetMask(
int mask)
25 m_BitCount =
Math.GetNumberOfSetBits(mask);
61 data.SetMask(data.GetMask() | mask);
85 return cache_data.GetMask();
93 return cache_data.GetBitCount();
103 Print(
"m_BitCount:"+
m_Recipes.GetElement(i).m_BitCount.ToString());
class RecipeCacheData m_Recipes
proto void Print(void var)
Prints content of variable to console/log.
int GetBitCountByRecipeID(int recipe_id)
array< int > GetRecipes()
bool IsContainRecipe(int recipe_id)
Result for an object found in CGame.IsBoxCollidingGeometryProxy.
bool AddRecipe(int recipe_id, int mask)
void UpdateMask(int recipe_id, int mask)
ref array< int > m_RecipeIDs
int GetMaskByRecipeID(int recipe_id)